MATLAB Repmat
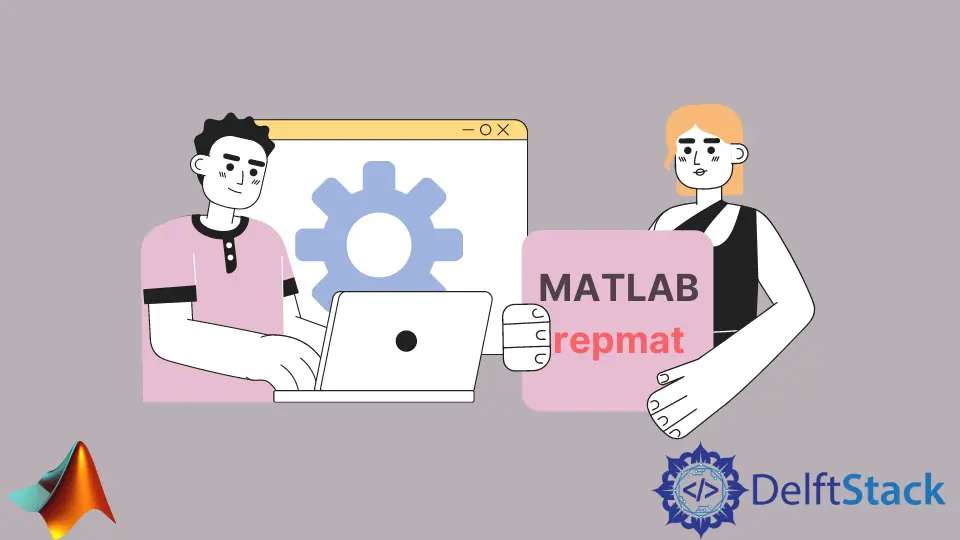
This tutorial will discuss making a matrix with the repeated values of an array using the repmat()
function in MATLAB.
MATLAB repmat()
Function
The repmat()
function creates large matrices from a small array. The function repeats the given values of the array to create the large matrix.
The repmat()
function has three basic syntaxes. The first syntax is given below.
M = repmat(A,n);
The above syntax returns an array containing the n
copies of A
in the dimensions of rows and columns. When A
is a matrix, the output matrix M
will equal the size of matrix A
multiplied by n
, greater than the size of A
.
The first argument of the repmat()
function is the input array can be a scalar, matrix, vector, or multidimensional array. The second argument will set the number of times the input array will be repeated on the row and column dimensions.
The second syntax of the repmat()
function is below.
M = repmat(A,r1,...,rN);
The above syntax returns a list of scalars that describe the arrangement of copies of A
in each dimension. When A
is a matrix with N
dimensions, the size of M
is greater than that of A
.
The r1
will define the number of times the input array will be repeated on the first dimension. The third syntax of the repmat()
function is given below.
M = repmat(A,r);
The above syntax returns an array containing copies of A
in the dimensions of rows and columns according to the repetition scheme with row vector r
.
For example, we can define the number of times the given array or matrix will repeat itself in the row and column dimension using the vector r
, which will contain the number of times the input array or matrix will be repeated to create the output matrix.
We can use the repmat()
function to create a matrix by repeating a single value. For example, let’s create a 2-by-2 matrix containing the value 5.
See the code below.
clc
clear
M = repmat(5,2,2)
Output:
M =
5 5
5 5
The clc
and clear
commands are used to clear the command and workspace window in the above code. The output is a 2-by-2 matrix containing only the number 5.
We can also use the repmat()
function to create a matrix from the repetition of another matrix. For example, let’s create a diagonal matrix using the diag()
function and then create a matrix by repeating the diagonal matrix.
See the code below.
clc
clear
D = diag([5 15 25])
M = repmat(D,2,3)
Output:
D =
5 0 0
0 15 0
0 0 25
M =
5 0 0 5 0 0 5 0 0
0 15 0 0 15 0 0 15 0
0 0 25 0 0 25 0 0 25
5 0 0 5 0 0 5 0 0
0 15 0 0 15 0 0 15 0
0 0 25 0 0 25 0 0 25
In the above code, we repeated the diagonal matrix 2 times in the row dimension and 3 times in the column dimension.
The size of the output matrix will become 6-by-9 because the input has three rows, and we repeated it 2 times so that the output row size will become 6, and the input matrix has three columns, and we repeated them 3 times so that the output column size will become 9.
We can also make 3D matrices with the repmat()
function. We have to use three values in the repetition scheme.
For example, let’s create a 6-by-3-by-2 matrix using the repmat()
function. See the code below.
clc
clear
D = diag([5 15 25])
M = repmat(D,2,1,2)
Output:
D =
5 0 0
0 15 0
0 0 25
M(:,:,1) =
5 0 0
0 15 0
0 0 25
5 0 0
0 15 0
0 0 25
M(:,:,2) =
5 0 0
0 15 0
0 0 25
5 0 0
0 15 0
0 0 25
As we can see, the output matrix is a 3-dimensional matrix. We can also create a matrix with a horizontal or vertical stack of row vectors.
For example, if we don’t want to repeat the vector on a dimension, we can pass 1 in its place. Inside the repmat()
function, the second argument defines the repetition scheme for the first or the row dimension.
The third argument defines the repetition scheme for the second or the column dimension.
For example, let’s create a matrix with a horizontal stack of a row vector. See the code below.
clc
clear
D = (1:5)'
M = repmat(D,1,5)
Output:
D =
1
2
3
4
5
M =
1 1 1 1 1
2 2 2 2 2
3 3 3 3 3
4 4 4 4 4
5 5 5 5 5
In the above code, we took the transpose of the given vector to convert it into a column vector to create the horizontal stack, but in the case of the vertical stack, we don’t have to take the transpose of the given vector.
We can also use the repmat()
function to create a large table from a small table. For example, let’s create a table using the table()
function and create another large table from the first table.
See the code below.
clc
clear
T_1 = table([19; 16],[60; 53],'VariableNames',{'Age' 'Height'})
T_2 = repmat(T_1, 2,2)
Output:
T_1 =
2×2 table
Age Height
___ ______
19 60
16 53
T_2 =
4×4 table
Age Height Age_1 Height_1
___ ______ _____ ________
19 60 19 60
16 53 16 53
19 60 19 60
16 53 16 53
In the above code, we repeated the row and the column dimension 2 times to create the larger table.