The nnz() Function in MATLAB
-
Syntax of the MATLAB
nnz()
Function -
Using Conditions With the
nnz()
Function in MATLAB -
Using Multiple Conditions With the
nnz()
Function in MATLAB -
Advanced Applications of the
nnz()
Function in MATLAB - Conclusion
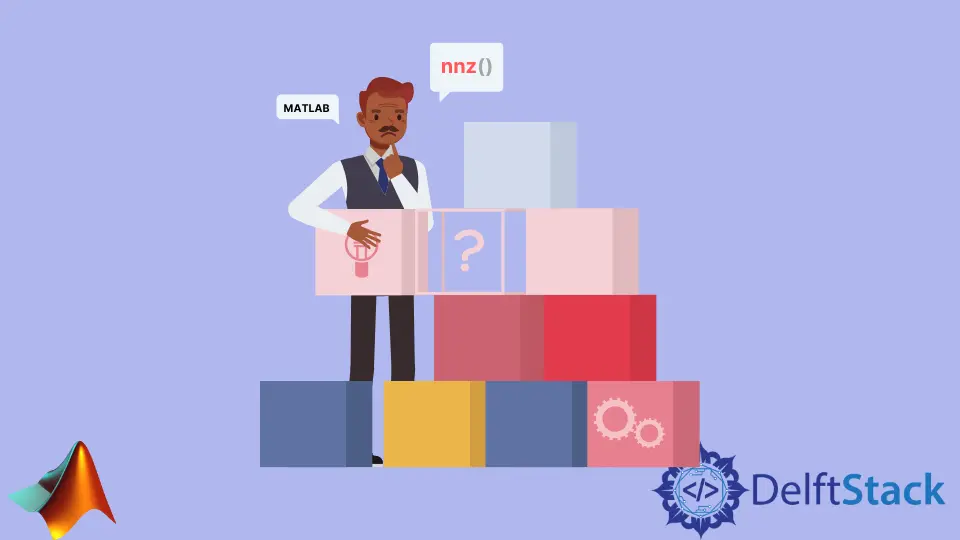
MATLAB, a powerful programming language and environment for numerical computing, offers a wide range of functions to manipulate and analyze data efficiently. One such function that proves invaluable in various scenarios is the nnz()
function.
Short for number of non-zeros
, nnz()
is a versatile tool for counting the non-zero elements in a matrix. In this article, we will explore the syntax, applications, and examples of using the nnz()
function in MATLAB.
Syntax of the MATLAB nnz()
Function
The basic syntax of the nnz()
function is straightforward:
num = nnz(m)
Here, m
represents the matrix or vector in question, and num
stores the total count of nonzero elements. It’s important to note that the input to the nnz()
function must be a matrix or vector.
Example 1: Counting Nonzero Elements in a Vector
Let’s begin with a simple example of counting nonzero elements in a vector.
m = [0 1 0 5]
num = nnz(m)
In this instance, the matrix A
has nine elements, and the nnz()
function effortlessly counts the number of nonzero elements, providing a valuable metric for sparse matrices or matrices with specific patterns.
Output:
A =
1 0 3
0 5 0
7 8 9
num_matrix = 6
Using Conditions With the nnz()
Function in MATLAB
The power of the nnz()
function lies in its ability to incorporate conditions for counting elements based on specific criteria.
Example 2: Counting Elements Greater Than a Threshold
m = [0 1 0 5]
num = nnz(m > 1)
Here, we introduce a condition within the nnz()
function to count elements greater than 1 in the vector m
. The condition is expressed as m > 1
. The resulting count is stored in the variable num
.
In the output below, we can observe that only the element 5
satisfies the condition of being greater than 1. Consequently, the nnz()
function returns 1
as the count of elements meeting the specified condition.
Output:
m =
0 1 0 5
num = 1
Example 3: Counting Elements Greater Than a Negative Value
m = [-5 -1 1 0 5]
num = nnz(m > -5)
In this example, we work with a vector m
containing elements [-5, -1, 1, 0, 5]
. The nnz()
function is used to count elements greater than -5.
Notably, this condition includes zero, as it is greater than -5. As a result, the nnz()
function correctly returns 4
, indicating that four elements in the vector satisfy the condition of being greater than -5.
Output:
m =
-5 -1 1 0 5
num = 4
Using Multiple Conditions With the nnz()
Function in MATLAB
We can also use logical AND (&
) and OR (|
) operators for more intricate conditions.
Example 4: Counting Elements Meeting Multiple Conditions
m = [15 10 1 0 5]
num = nnz(m > 1 & m < 15)
num_2 = nnz(m > 5 | m < 1)
In this example, the matrix B
is defined, and we use nnz(B(:, column_index))
to count the nonzero elements along column 3, storing the result in the variable nonzero_count_column
. Similarly, nnz(B(row_index, :))
is employed to count nonzero elements along row 2, and the result is stored in the variable nonzero_count_row
.
The output reveals the count of nonzero elements for each specified column and row, providing valuable insights into the distribution of nonzero values in the matrix.
Output:
B =
1 0 3
0 5 0
7 8 9
nonzero_count_column = 2
nonzero_count_row = 1
Here, nonzero_count_column
provides the count of nonzero elements in column 3, and nonzero_count_row
gives the count for row 2.
Example 6: Counting Nonzero Elements in a Sparse Matrix
In MATLAB, sparse matrices are often employed to efficiently handle large datasets with mostly zero elements. nnz()
can be used to determine the density of nonzero elements in a sparse matrix, aiding in memory optimization.
% Creating a sparse matrix
S = sparse([1, 2, 2], [2, 1, 2], [3, 4, 5])
count_nonzero_sparse = nnz(S)
Here, we first create a sparse matrix S
using the sparse()
function with specified row indices, column indices, and values. The nnz(S)
function is then employed to count the nonzero elements in the sparse matrix.
The output shows that the sparse matrix S
contains three nonzero elements, illustrating the ability of nnz()
to seamlessly extend to sparse data structures.
Output:
S =
Compressed Column Sparse (rows = 2, cols = 2, nnz = 3 [75%])
(2, 1) -> 4
(1, 2) -> 3
(2, 2) -> 5
count_nonzero_sparse = 3
The sparse matrix S
has three nonzero elements, and nnz()
accurately returns this count.
Example 7: Counting Nonzero Elements in Logical Arrays
Logical arrays, consisting of Boolean values (true
or false
), can be efficiently processed using the nnz()
function.
C = [true, false, true; false, true, false; true, true, true]
count_nonzero_logical = nnz(C)
Here, we create a random binary matrix D
using the randi()
function, generating a 4x4 matrix with elements either 0
or 1
. To count the nonzero elements in the region specified by rows 2 to 3 and columns 2 to 3, we use nnz(D(2:3, 2:3))
.
The output displays that there are four nonzero elements in this defined region, showcasing the versatility of nnz()
in isolating and counting elements within specific matrix regions.
Output:
D =
1 1 1 0
1 0 1 0
0 1 1 1
1 0 0 1
count_nonzero_region = 3
In this example, nnz()
counts the four nonzero elements in the specified region of the matrix D
.
These advanced examples demonstrate the flexibility of the nnz()
function in MATLAB. Whether dealing with specific dimensions, sparse matrices, logical arrays, or isolated regions, nnz()
proves to be a valuable tool for diverse applications.
Conclusion
In conclusion, the nnz()
function in MATLAB serves as a versatile tool for efficiently counting nonzero elements in matrices and vectors. Its basic syntax is user-friendly, making it accessible to users at various proficiency levels.
Whether applied to vectors, matrices, or sparse arrays, the function excels in providing a clear count of elements that meet specific conditions.
By understanding the nnz()
function, you can streamline your data analysis processes. The ability to incorporate conditions within the function enhances its utility, allowing for tailored element counts based on user-defined criteria.
In practical terms, incorporating the nnz()
function into your MATLAB workflow can significantly enhance your efficiency and effectiveness in dealing with numerical data. As you explore its capabilities through examples and applications, you’ll find that the nnz()
function not only simplifies the counting process but also offers a powerful tool for data manipulation and analysis.