How to Check Whether an Array or Matrix Is Empty or Not in MATLAB
-
Determine Empty Arrays or Matrices in MATLAB Using the
isempty()
Function -
Determine Empty Arrays or Matrices in MATLAB Using the
numel()
Function -
Determine Empty Arrays or Matrices in MATLAB Using the
size()
Function - Conclusion
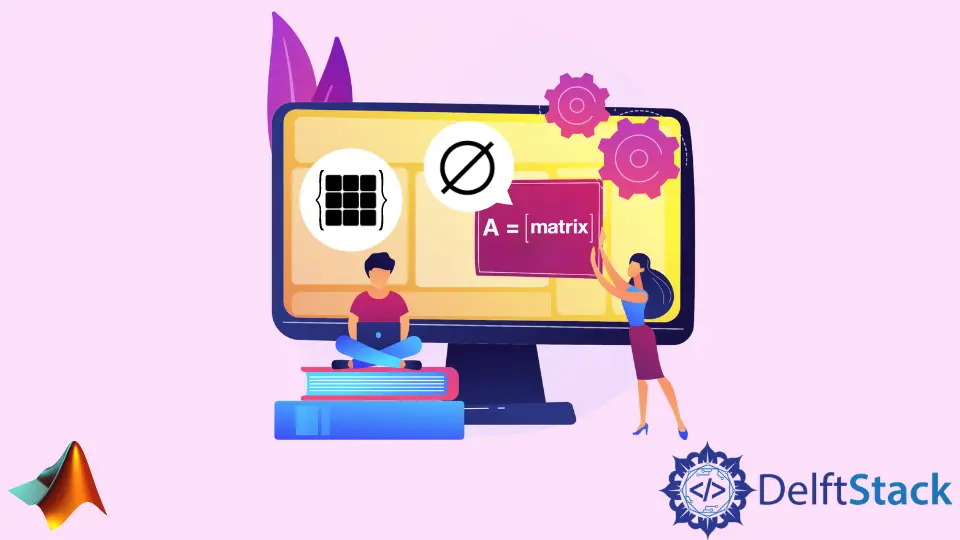
When working with MATLAB, it’s often crucial to assess whether an array or matrix is empty. MATLAB provides several methods for this purpose, each offering unique insights into the nature of the data structure.
In this article, we’ll explore three prominent methods: isempty()
, numel()
, and size()
, and illustrate their application through a comprehensive example.
Determine Empty Arrays or Matrices in MATLAB Using the isempty()
Function
One powerful tool for checking whether an array or matrix is empty is the isempty()
function.
The isempty()
function in MATLAB is used to check if a matrix or array is empty. The syntax is quite straightforward:
result = isempty(A);
Here, A
is the array or matrix being checked. The result
will be 1
if the array is empty and 0
if it is not.
The isempty()
function examines the dimensions of the input array. If the array has dimensions 0 x 0
, it is considered empty.
Additionally, matrices with dimensions 0 x n
or n x 0
are also recognized as empty. The function returns a logical value, where 1
indicates true (empty) and 0
indicates false (not empty).
Now, let’s explore a complete working example to illustrate the practical use of isempty()
.
A = [1, 2, 3];
B = [];
C = rand(2, 2, 2);
D = [1 3 5; 2 4 6; 7 8 10];
D(:, :, :) = []; % Deleting the elements of matrix D
result_A = isempty(A);
result_B = isempty(B);
result_C = isempty(C);
result_D = isempty(D);
disp('Result for array A: '); disp(result_A)
disp('Result for array B: '); disp(result_B)
disp('Result for matrix C: '); disp(result_C)
disp('Result for matrix D: '); disp(result_D)
In this example, we begin by defining various arrays and matrices, namely A
, B
, C
, and D
. We then employ the isempty()
function to check if each of these arrays or matrices is empty.
The results are stored in variables result_A
, result_B
, result_C
, and result_D
.
For array A
, which contains elements, result_A
will be 0
(not empty). For array B
, which is explicitly defined as an empty array, result_B
will be 1
(empty).
Matrix C
with random elements will yield 0
as it is not empty. Matrix D
, after deleting its elements using D(:, :, :) = []
, will be considered empty, resulting in 1
for result_D
.
The disp()
function is then used to display these results, providing a clear output indicating whether each array or matrix is empty or not.
Output:
The isempty()
function proves to be a straightforward yet powerful tool for checking the emptiness of arrays and matrices in MATLAB, enabling efficient and reliable coding practices.
Determine Empty Arrays or Matrices in MATLAB Using the numel()
Function
In addition to the isempty()
function, MATLAB provides another useful tool, the numel()
function, to determine whether an array or matrix is empty.
The numel()
function in MATLAB calculates the number of elements in an array. The syntax is as follows:
num_elements = numel(A);
Here, A
is the array or matrix for which we want to determine the number of elements, and num_elements
will store that count.
When applied to an array or matrix, the numel()
function returns the total number of elements. If the array is empty, the function will return 0
, as there are no elements to count.
This property makes numel()
an effective tool for checking the emptiness of arrays or matrices in MATLAB.
Now, let’s dive into a complete working example to showcase the practical application of numel()
.
A = [1, 2, 3];
B = [];
C = rand(2, 2, 2);
D = [1 3 5; 2 4 6; 7 8 10];
D(:, :, :) = []; % Deleting the elements of matrix D
num_elements_A = numel(A);
num_elements_B = numel(B);
num_elements_C = numel(C);
num_elements_D = numel(D);
disp('Number of elements in array A: '); disp(num_elements_A)
disp('Number of elements in array B: '); disp(num_elements_B)
disp('Number of elements in matrix C: '); disp(num_elements_C)
disp('Number of elements in matrix D: '); disp(num_elements_D)
In this example, we start by defining arrays and matrices (A
, B
, C
, and D
). We then employ the numel()
function to calculate the number of elements in each array or matrix.
The results are stored in variables num_elements_A
, num_elements_B
, num_elements_C
, and num_elements_D
.
For array A
, which has three elements, num_elements_A
will be 3
. For the empty array B
, num_elements_B
will be 0
.
Matrix C
, with random elements, will yield the total count of its elements. Matrix D
, after deleting its elements using D(:, :, :) = []
, will be considered empty, resulting in 0
for num_elements_D
.
The disp()
function is then used to display these results, providing a clear output indicating the number of elements in each array or matrix.
Output
The numel()
function proves to be an effective tool for determining the emptiness of arrays and matrices in MATLAB by counting the total number of elements.
Determine Empty Arrays or Matrices in MATLAB Using the size()
Function
The size()
function offers yet another method to assess whether an array or matrix is empty. The size()
function in MATLAB is employed to determine the dimensions of an array or matrix.
The syntax is as follows:
dimensions = size(A);
Here, A
represents the array or matrix under consideration, and dimensions
will store a vector specifying the size of each dimension.
When applied to an array or matrix, the size()
function returns a vector indicating the size of each dimension. If any dimension has a length of 0
, the array or matrix is considered empty.
This characteristic makes size()
a valuable tool for checking emptiness in MATLAB.
Let’s proceed with a comprehensive example that illustrates the practical use of size()
.
A = [1, 2, 3];
B = [];
C = rand(2, 2, 2);
D = [1 3 5; 2 4 6; 7 8 10];
D(:, :, :) = []; % Deleting the elements of matrix D
dimensions_A = size(A);
dimensions_B = size(B);
dimensions_C = size(C);
dimensions_D = size(D);
disp('Dimensions of array A: '); disp(dimensions_A)
disp('Dimensions of array B: '); disp(dimensions_B)
disp('Dimensions of matrix C: '); disp(dimensions_C)
disp('Dimensions of matrix D: '); disp(dimensions_D)
In this example, arrays and matrices (A
, B
, C
, and D
) are defined. The size()
function is then employed to obtain the dimensions of each array or matrix.
The results are stored in vectors (dimensions_A
, dimensions_B
, dimensions_C
, and dimensions_D
).
For array A
, which is not empty, dimensions_A
will be a vector indicating the size of its dimension(s). For the empty array B
, dimensions_B
will be [0, 0]
, clearly signifying its emptiness.
Matrix C
will yield a vector representing its three-dimensional size. Matrix D
, after deleting its elements using D(:, :, :) = []
, will be considered empty, resulting in [0, 0, 0]
for dimensions_D
.
The disp()
function is then used to display these results, offering clear output regarding the dimensions of each array or matrix.
Output:
Conclusion
MATLAB offers multiple methods, including isempty()
, numel()
, and size()
, for determining whether an array or matrix is empty. Each method provides unique insights into the structure of the data, allowing you to choose the most suitable approach based on your specific needs.
By incorporating these techniques into your MATLAB code, you can efficiently and accurately check for emptiness, contributing to the clarity and reliability of your programming endeavors. Whether examining dimensions, counting elements, or assessing logical conditions, the versatility of these methods empowers MATLAB users to handle empty arrays and matrices with precision and ease.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - MATLAB Array
- MATLAB Repelem() Function
- How to Create Array of Zeros in MATLAB
- How to Find Index of Value in Array in Matlab
- How to Get Average of Array With the mean() Function in Matlab
- How to Sum Elements of an Array in MATLAB