How to Sum Elements of an Array in MATLAB
-
Find the Sum of Array Elements in MATLAB Using the
sum()
Function - Find the Sum of Array Elements in MATLAB Using Loops
- Conclusion
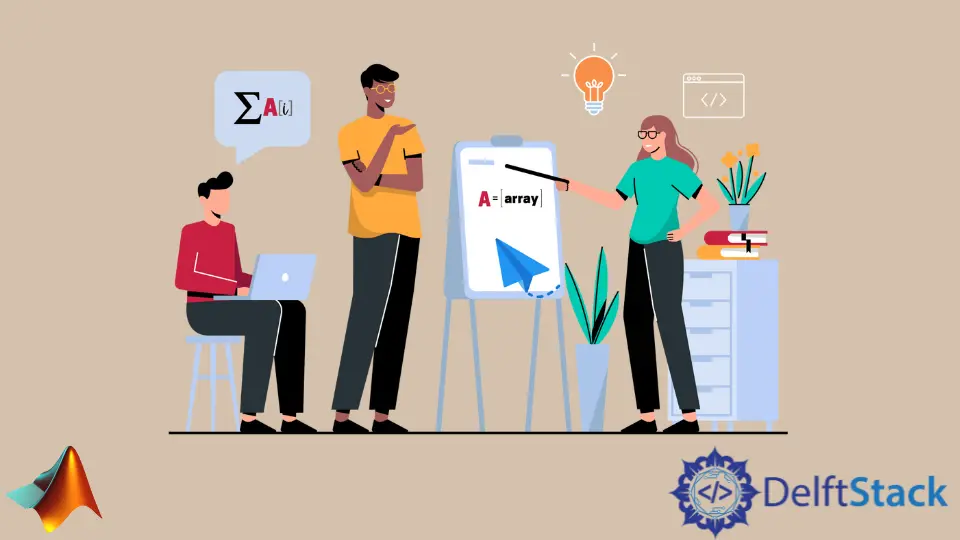
MATLAB, a powerful numerical computing environment, is widely used for various applications in engineering, science, and mathematics. One common task in MATLAB programming is finding the sum of elements in an array.
This process is straightforward and can be accomplished using built-in functions. In this article, we will explore different methods to calculate the sum of array elements in MATLAB.
Find the Sum of Array Elements in MATLAB Using the sum()
Function
The sum()
function in MATLAB is designed to calculate the sum of elements in an array along a specified dimension. It can be applied to vectors, matrices, or multidimensional arrays.
The basic syntax of the sum
function is as follows:
totalSum = sum(array);
Where array
is the input array, and totalSum
is the sum of all its elements.
Example 1: Simple Array Summation
Let’s start with a straightforward example:
array = [1, 2, 3, 4, 5];
totalSum = sum(array);
disp(totalSum);
Here, we start with the basic task of finding the sum of elements in a one-dimensional array. The array [1, 2, 3, 4, 5]
is defined, and the sum
function is applied to calculate the sum of all its elements.
The result, which is the total sum, is then displayed using the disp
function. In this case, the output is 15
, representing the sum of 1 + 2 + 3 + 4 + 5
.
Output:
15
Example 2: Summing Elements With a Condition
In this example, we use the sum
function with a logical condition:
array = [1, 2, 3, 4, 5];
condition = array > 2;
totalSum = sum(array(condition));
disp(totalSum);
We introduce the concept of applying the sum
function with a logical condition. Here, we use the array [1, 2, 3, 4, 5]
again and create a condition (array > 2
).
The condition filters elements greater than 2, and the sum
function is then applied to calculate the sum of these selected elements. The output, which is 12
, reflects the sum of 3 + 4 + 5
.
Output:
12
Example 3: Summing Along a Specific Dimension
The sum
function can operate along a specified dimension in multidimensional arrays:
matrix = [1, 2, 3; 4, 5, 6; 7, 8, 9];
totalSum = sum(matrix, 2);
disp(totalSum);
Here, we explore the ability of the sum
function to operate along a specified dimension in a two-dimensional array (matrix). We create a 3x3 matrix, and by specifying the dimension (2
for columns), the sum
function computes the sum along each column.
The output below represents the sums of the columns.
Output:
6
15
24
Example 4: Cumulative Sum
The cumsum
function can be used for cumulative summation:
array = [1, 2, 3, 4, 5];
cumulativeSum = cumsum(array);
disp(cumulativeSum);
This example introduces the cumsum
function, which calculates the cumulative sum of elements in an array. We apply the cumsum
function to the array [1, 2, 3, 4, 5]
, resulting in a new array where each element is the sum of all preceding elements.
The output below shows the cumulative sums at each position in the array.
Output:
1 3 6 10 15
Example 5: Vectorized Summation
Utilizing vectorized operations for array summation:
matrix = [1, 2, 3; 4, 5, 6; 7, 8, 9];
totalSum = sum(matrix(:));
disp(totalSum);
In this example, we demonstrate a vectorized approach to array summation. In this case, we work with a two-dimensional matrix [1, 2, 3; 4, 5, 6; 7, 8, 9]
.
Using the (:)
operator, we linearize the matrix into a vector, and the sum
function calculates the sum of all elements in the resulting vector. The output generated represents the sum of all elements in the original matrix.
Output:
45
MATLAB’s sum
function provides an efficient way to find the sum of elements in an array, with options for conditional summation, multidimensional arrays, and cumulative summation.
Find the Sum of Array Elements in MATLAB Using Loops
While MATLAB provides convenient built-in functions like sum
for array summation, there are scenarios where using a loop may be necessary or preferred. This approach not only enhances your programming skills but also allows for a deeper understanding of the underlying process.
The basic syntax for finding the sum of elements using a loop is as follows:
for i = 1:length(array)
S = totalSum + array(i);
end
Here, array
is the input array, and S
is the total sum of the array elements. The loop iterates through each element of the array, adding it to the total sum.
Example 1: Simple Array Summation With a Loop
Let’s begin with a straightforward example:
array = [1, 2, 3, 4, 5];
totalSum = 0;
for i = 1:length(array)
totalSum = totalSum + array(i);
end
disp(totalSum);
In this example, we use a loop to iterate through each element of the array [1, 2, 3, 4, 5]
. Inside the loop, each element is added to the totalSum
.
The loop continues until all elements have been processed. Finally, the disp
function is used to showcase the total sum, which, in this case, is 15
.
This approach is a basic illustration of how loops can be employed for array summation.
Output:
15
Example 2: Conditional Summation With a Loop
Expanding on the loop-based approach, we can incorporate a condition:
array = [1, 2, 3, 4, 5];
totalSum = 0;
for i = 1:length(array)
if array(i) > 2
totalSum = totalSum + array(i);
end
end
disp(totalSum);
Building upon the previous example, we introduce a conditional statement within the loop. Here, we add elements to the sum only if they are greater than 2.
The if
statement checks the condition array(i) > 2
, ensuring that only qualifying elements contribute to the final totalSum
.
Output:
12
Example 3: Summation of Absolute Values With a Loop
Another variation involves calculating the sum of absolute values:
array = [-1, 2, -3, 4, -5];
totalSum = 0;
for i = 1:length(array)
totalSum = totalSum + abs(array(i));
end
disp(totalSum);
In this case, we modify the loop to calculate the sum of absolute values in the array [-1, 2, -3, 4, -5]
. Inside the loop, the abs
function is applied to each element before adding it to the totalSum
.
The output should reflect the sum of the absolute values of all elements in the array.
Output:
15
This example illustrates how loops can be utilized for specific arithmetic operations during summation.
Example 4: Cumulative Sum With a Loop
Finally, let’s implement a loop for cumulative summation:
array = [1, 2, 3, 4, 5];
cumulativeSum = zeros(size(array));
for i = 1:length(array)
cumulativeSum(i) = sum(array(1:i));
end
disp(cumulativeSum);
Here, we initialize a cumulativeSum
array with zeros, and within the loop, we calculate the sum of elements from the beginning up to the current position. This process is repeated for each element in the array, resulting in a cumulativeSum
array that represents the cumulative sums at each position.
Output:
1 3 6 10 15
While MATLAB’s sum
function is often more concise and efficient, understanding how to calculate array sums using loops is valuable for learning programming fundamentals.
Conclusion
In MATLAB, finding the sum of elements in an array is a common operation with multiple methods available. The choice between using the sum()
function, a loop, or the cumulative sum method depends on your specific needs and coding preferences.
Understanding these different approaches equips you with the flexibility to efficiently handle array summation tasks in your MATLAB projects.