MATLAB Repelem() Function
-
MATLAB
repelem()
Function -
Error Handling and Limitations of the
repelem()
Function in MATLAB - Conclusion
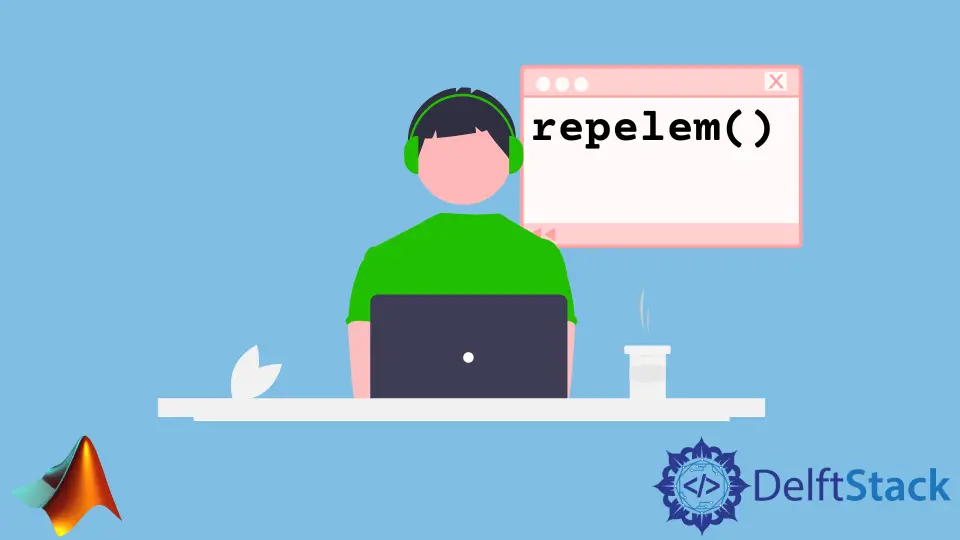
MATLAB, a high-level programming language and environment, is widely used for numerical computing and data analysis. One of the key features of MATLAB is its extensive set of functions designed to simplify various computational tasks.
Among these functions, repelem()
stands out for its versatility and usefulness in repetitive tasks involving array manipulation. In this article, we’ll delve into the details of the repelem()
function and explore its various applications.
MATLAB repelem()
Function
The repelem()
function in MATLAB is primarily used to repeat or replicate elements of an array in a specified pattern. It provides a flexible way to create repeated patterns within an array, allowing for efficient handling of repetitive tasks in data processing and analysis.
The repelem()
function in MATLAB has two primary syntaxes:
Basic Syntax:
output = repelem(v, n)
Where:
v
: The input vector or scalar to be repeated.n
: The number of times each element ofv
should be repeated.- Returns a vector of repeated elements.
Extended Syntax for Multidimensional Arrays:
output = repelem(m, r1, ..., rN)
Where:
m
: The input matrix or array to be repeated.r1, ..., rN
: Block sizes for replication along different dimensions.- Returns a new matrix with repeated elements.
The repelem()
function works by taking an input array or scalar and repeating its elements according to specified parameters.
When given a vector or scalar and a repetition factor, it replicates each element the specified number of times, resulting in a longer output vector. For multidimensional arrays, it repeats elements along each dimension according to the specified block sizes.
Let’s dive into several code examples to illustrate the versatility of the repelem()
function.
Example 1: Basic Replication of a Vector
Let’s start with a simple example to illustrate the basic usage of repelem()
. Suppose we have an array X
:
X = [1 2 3];
Y = repelem(X, 2)
Similarly, in this example, we have a vector X
with the same elements [1 2 3]
. However, instead of a fixed replication count, we provide a vector [1 2 3]
as the second argument to the repelem()
function.
This indicates that each element of X
should be replicated once, twice, and thrice, respectively. As a result, the vector Y
will contain the following elements where: the first element of X
is repeated once, the second element twice, and the third element thrice.
Output:
Y =
1 2 2 3 3 3
Example 3: Replication of Multidimensional Arrays
The repelem()
function is not limited to one-dimensional arrays; it can also handle multidimensional arrays efficiently. When dealing with multidimensional arrays, repelem()
repeats elements along each dimension independently.
X = magic(2);
Y = repelem(X, 2, 2)
In this example, we generate a 2x2 magic square matrix X
using the magic()
function. We then apply the repelem()
function to this matrix, specifying 2
as the block size for both rows and columns.
This means that each element of the matrix X
will be repeated twice along both dimensions. As a result, the resulting matrix Y
will be a 4x4 matrix where each element of X
is repeated twice along both rows and columns, preserving the magic square pattern.
Output:
Y =
4 4 3 3
4 4 3 3
1 1 2 2
1 1 2 2
Example 4: Custom Replication Along Specific Dimensions
Sometimes, you may want to repeat elements along specific dimensions of a multidimensional array. repelem()
allows you to achieve this by specifying an additional argument for the dimension along which repetition should occur.
If certain dimensions shouldn’t be replicated, the value 1
can be passed for those dimensions. Additionally, vectors of the same length as specific dimensions can specify the number of repetitions for individual elements.
X = magic(2);
Y = repelem(X, 1, [3 2])
Here, we create another 2x2 magic square matrix, X
. However, instead of repeating elements along both dimensions, we customize the replication by specifying [3 2]
as the block size for the row dimension.
This means that the first column of X
will be repeated three times, and the second column will be repeated twice. Therefore, the resulting matrix Y
will be a 2x5 matrix with the first column of X
repeated three times and the second column repeated twice.
Output:
Y =
4 4 4 3 3
1 1 1 2 2
Example 5: Replication With Varying Block Sizes
Another useful application of repelem()
is in replicating elements of an array with varying block sizes. By specifying the size of the output array and repetition pattern, we can replicate the input array to fill the specified dimensions of the output array.
matrix = [1 2; 3 4];
Y = repelem(matrix, [2 1], [3 2])
Replicating a large matrix-like X
multiple times can lead to high memory consumption, potentially causing performance issues or memory exhaustion.
Performance Considerations
While repelem()
provides a convenient way to replicate elements, it’s important to consider performance implications when working with large arrays or matrices. Replicating large arrays multiple times can consume significant memory and processing resources.
In such cases, alternative approaches like preallocating memory or using vectorized operations may offer better performance.
For large-scale replication tasks, using functions like repmat()
may provide better performance compared to repelem()
.
Conclusion
The repelem()
function in MATLAB is a powerful tool for repeating elements within arrays or matrices. Its flexibility allows for simple repetition as well as more complex patterns, making it invaluable for various data manipulation tasks.
By understanding its syntax and various usage scenarios, you can leverage repelem()
to streamline your MATLAB code and expedite your workflow.
Whether you’re working with numerical arrays, strings, or custom data types, repelem()
offers a convenient solution for repeating elements, enabling you to focus more on the logic of your algorithms rather than the mechanics of data manipulation. So, next time you find yourself needing to repeat elements in MATLAB, remember the repelem()
function and its versatility.