How to Create Array of Zeros in MATLAB
-
Create Arrays of Zeros in MATLAB Using the
zeros()
Function -
Create Sparse Arrays of Zeros in MATLAB Using the
sparse()
Function - Conclusion
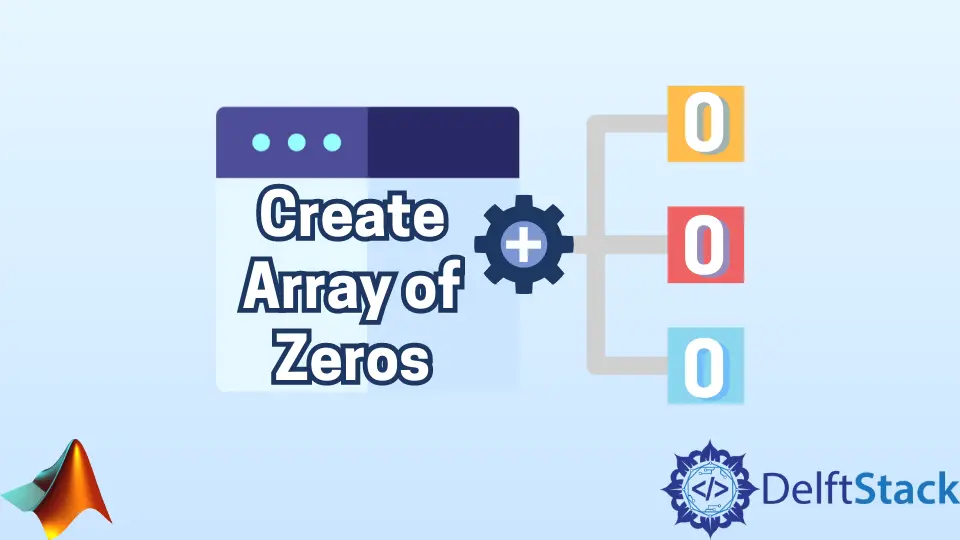
MATLAB, a powerful numerical computing environment, provides a multitude of tools for data manipulation and analysis. One fundamental operation frequently encountered in MATLAB programming is the creation of arrays.
In this article, we will explore the various ways to create an array of zeros in MATLAB.
Create Arrays of Zeros in MATLAB Using the zeros()
Function
MATLAB simplifies the task of generating arrays filled with zeros with the zeros()
function.
The zeros()
function in MATLAB is designed to generate an array filled with zeros. It takes one or more arguments to specify the dimensions of the array.
The basic syntax is as follows:
Z = zeros(m, n);
Here, Z
is the output array of size m
-by-n
filled with zeros. The function can also take additional arguments to create arrays with more than two dimensions.
For example:
Z = zeros(m, n, p, ...);
This creates a multidimensional array with dimensions m
, n
, p
, and so on, filled with zeros.
Example 1: Creating a Square Matrix
% Creating a 3x3 matrix of zeros
ZeroMatrix = zeros(3);
ZeroMatrix
In this example, we demonstrate the fundamental use of the zeros()
function to create a 3x3 matrix filled with zeros. The code simply calls zeros(3)
, where the single scalar input denotes the dimensions of the desired square matrix.
The resulting matrix, stored in the variable ZeroMatrix
, is displayed as:
ZeroMatrix =
0 0 0
0 0 0
0 0 0
This straightforward example demonstrates the basic usage of the zeros()
function for creating square matrices.
Example 2: Specifying Dimensions as a Vector
% Creating a 2x3 matrix of zeros
ZeroMatrix = zeros([2 3]);
ZeroMatrix
Here, we showcase the flexibility of the zeros()
function by passing a vector [2 3]
as input, generating a 2x3 matrix of zeros. The line ZeroMatrix = zeros([2 3])
initializes a matrix with the specified dimensions, and the output is:
Output:
ZeroMatrix =
0 0 0
0 0 0
This example illustrates how to define non-square matrix dimensions using the zeros()
function.
Example 3: Creating a 3D Array
% Creating a 1x2x3 3D matrix of zeros
ZeroMatrix = zeros(1, 2, 3);
ZeroMatrix
In this example, we explore how to create a matrix of zeros with the same dimensions as an existing matrix (originalMatrix
). By utilizing the size()
function, we retrieve the size information and pass it to the zeros()
function.
The output reveals the creation of a new matrix filled with zeros but sharing the dimensions of originalMatrix
.
Output:
originalMatrix =
1 2
3 6
ZeroMatrix =
0 0
0 0
This example demonstrates how to create a matrix of zeros with dimensions matching an existing matrix.
Example 5: Specifying Data Type
% Creating a matrix of zeros with a specified data type
originalMatrix = int16([1 2 3 6]);
ZeroMatrix = zeros(size(originalMatrix), 'like', originalMatrix);
originalMatrix
ZeroMatrix
This example illustrates the like
property within the zeros()
function, allowing us to create a matrix of zeros with the same data type as another matrix (originalMatrix
). By using int16
as the data type, the resulting ZeroMatrix
maintains consistency.
Output:
originalMatrix =
1×4 int16 row vector
1 2 3 6
ZeroMatrix =
1×4 int16 row vector
0 0 0 0
This example highlights how to maintain consistent data types when creating matrices using the zeros()
function.
Example 6: Specifying Data Type Directly
% Creating a matrix of zeros with a specified data type
originalMatrix = [1 2 3 6];
ZeroMatrix = zeros(size(originalMatrix), 'int8');
originalMatrix
ZeroMatrix
In this final example, we showcase an alternative approach to create a vector of zeros. The colon
operator generates indices (1 through 10), and the zeros()
function is employed to initialize a vector of zeros with the same size as the indices.
Output:
ZeroVector =
0 0 0 0 0 0 0 0 0 0
This example provides an alternative approach for creating arrays of zeros using the colon operator and the zeros()
function.
The zeros()
function in MATLAB provides a flexible and efficient way to create arrays filled with zeros. Whether you need a simple matrix, a multi-dimensional array, or a specific data type, the zeros()
function is a valuable tool for array initialization in MATLAB.
Create Sparse Arrays of Zeros in MATLAB Using the sparse()
Function
In addition to the zeros()
function, MATLAB offers another powerful tool for generating arrays of zeros with a specialized purpose - the sparse()
function.
The sparse()
function is particularly useful for dealing with large matrices that are predominantly filled with zeros. It creates a sparse matrix, a data structure that only stores non-zero elements, resulting in significant memory savings and computational efficiency.
The basic syntax is as follows:
S = sparse(i, j, s, m, n);
Where:
i
: Vector of row indices for the non-zero elements.j
: Vector of column indices for the non-zero elements.s
: Vector of the non-zero elements.m
: Number of rows in the matrix.n
: Number of columns in the matrix.
In the context of creating an array of zeros, the s
parameter is not explicitly required, as we are interested in generating a matrix filled with zeros. The i
and j
vectors specify the positions of the non-zero elements, and m
and n
define the size of the matrix.
Example 1: Creating a Sparse Matrix
% Creating a sparse 3x3 matrix of zeros
SparseMatrix = sparse(3, 3);
SparseMatrix
In this example, we illustrate the fundamental use of the sparse()
function to create a sparse matrix filled with zeros. The code SparseMatrix = sparse(3, 3)
generates a sparse 3x3 matrix efficiently representing only the zeros.
The resulting SparseMatrix
is displayed, showcasing the memory-efficient sparse representation.
Output:
SparseMatrix =
All zero sparse: 3×3
Example 2: Creating a Sparse Vector
% Creating a sparse row vector of zeros
SparseVector = sparse(1, 5);
SparseVector
Here, we demonstrate creating a sparse row vector using sparse(1, 5)
. The resulting SparseVector
efficiently represents a row vector with zeros, storing only the information about the non-zero elements.
This is particularly useful for large vectors with mostly zero elements.
Output:
SparseVector =
(1,1) 0 (1,2) 0 (1,3) 0 (1,4) 0 (1,5) 0
Example 3: Creating a Sparse Matrix With Non-Zero Elements
% Creating a sparse 4x4 matrix with some non-zero elements
SparseNonZero = sparse([1, 3, 2], [2, 4, 3], [5, 8, 1], 4, 4);
SparseNonZero
In this example, we showcase the ability of the sparse()
function to handle matrices with non-zero elements. The input vectors [1, 3, 2]
, [2, 4, 3]
, and [5, 8, 1]
represent the row indices, column indices, and values, respectively.
The resulting SparseNonZero
matrix efficiently represents only the provided non-zero elements.
Output:
SparseNonZero =
Compressed Column Sparse (rows = 4, cols = 4, nnz = 3 [19%])
(1, 2) -> 5
(2, 3) -> 1
(3, 4) -> 8
Example 4: Creating a Sparse Matrix From a Dense Matrix
% Creating a sparse matrix from a dense matrix
DenseMatrix = eye(3);
SparseFromDense = sparse(DenseMatrix);
SparseFromDense