How to Disable Checkboxes in jQuery
-
Disable Checkboxes Using the
.attr()
Method in jQuery -
Disable Checkboxes Using the
.prop()
Method in jQuery
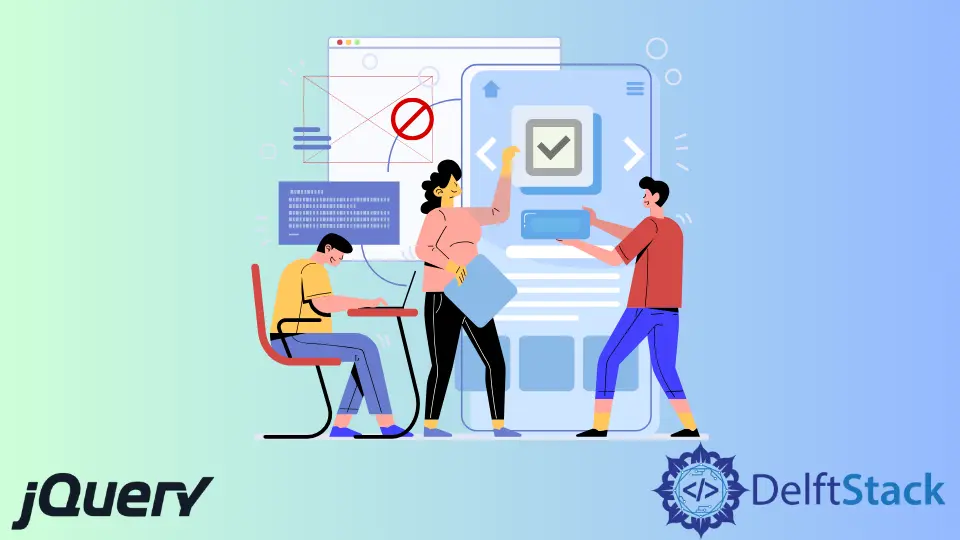
To disable checkboxes in jQuery, you can use the jQuery .attr()
and prop()
methods.
This article teaches you how to do it using two detailed examples.
Disable Checkboxes Using the .attr()
Method in jQuery
To disable checkboxes in your HTML form, you can use the attr()
method to add a disabled
attribute to each checkbox. To enable them, you can attach a click event to another checkbox.
So, when you check it, the remaining checkboxes will become enabled. At the same time, when you uncheck it, it’ll return to a disabled
state.
The following code shows you how to do it, and the results follow.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-jQuery-disable-checkboxes</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
form {
outline: 2px solid #1a1a1a;
width: 50%;
margin: 0 auto;
padding: 0.5em;
}
label {
display: block;
cursor: pointer;
font-size: 1.2em;
}
[disabled] {
cursor: not-allowed;
}
label[style^="opacity: 0.4"] {
cursor: not-allowed;
}
label:first-child:hover {
background-color: #aaa;
}
</style>
</head>
<body>
<main>
<form id="inventors-list">
<label>
<input type="checkbox" id="disable-all-checkboxes">Enable all options
</label>
<label>
<input type="checkbox" class="inventor"> Thomas Edison
</label>
<label>
<input type="checkbox" class="inventor"> Albert Einstein
</label>
<label>
<input type="checkbox" class="inventor"> Ernest Rutherford
</label>
</form>
</main>
<script>
$(function() {
enable_all_checkboxes();
$("#disable-all-checkboxes").click(enable_all_checkboxes);
});
function enable_all_checkboxes() {
if (this.checked) {
$("input.inventor").removeAttr("disabled");
$("input.inventor").parent().css('opacity', '')
} else {
$("input.inventor").attr("disabled", true);
$("input.inventor").parent().css('opacity', '0.4')
}
}
</script>
</body>
</html>
Output:
Disable Checkboxes Using the .prop()
Method in jQuery
The prop()
method is another way to disable checkboxes using jQuery. This means you’ll not use the disabled
attribute, as shown in the first example.
In the following code, we have four checkboxes, and three are disabled by default. You’ll enable the remaining ones when you click on the first checkbox.
They’ll return to a disabled
state if you click it the second time.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-disable-checkboxes</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
form {
outline: 2px solid #1a1a1a;
width: 50%;
margin: 0 auto;
padding: 0.5em;
}
label {
display: block;
cursor: pointer;
font-size: 1.2em;
}
[disabled] {
cursor: not-allowed;
}
label:first-child:hover {
background-color: #aaa;
}
</style>
</head>
<body>
<main>
<form id="algorithm-list">
<label>
<input type="checkbox" id="disable-all-checkboxes">Enable all options
</label>
<label>
<input type="checkbox" class="algo"> Binary search
</label>
<label>
<input type="checkbox" class="algo"> Merge Sort
</label>
<label>
<input type="checkbox" class="algo"> Linked List
</label>
</form>
</main>
<script>
$(function() {
enable_all_checkboxes();
$("#disable-all-checkboxes").click(enable_all_checkboxes);
});
function enable_all_checkboxes() {
$("input.algo").prop("disabled", !this.checked);
}
</script>
</body>
</html>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn