How to Toggle Checkboxes on and Off in jQuery
-
Toggle Checkboxes Using the
.prop()
Method -
Toggle Checkboxes Using the
.each()
Method -
Toggle Checkboxes Using the
.trigger()
Method
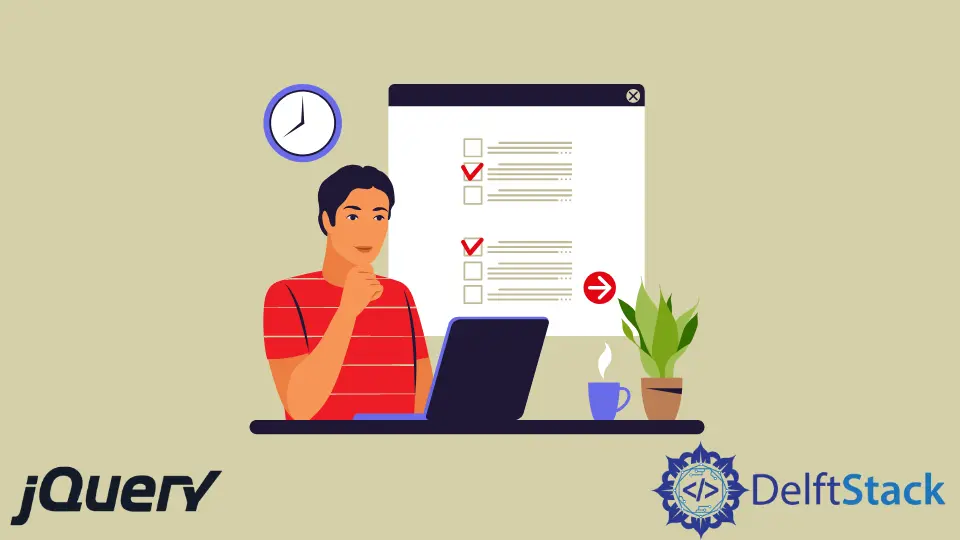
This article explains three methods that’ll let you toggle multiple checkboxes at once using jQuery. The three methods will use three methods available in jQuery; these are .prop()
, .each()
, and .trigger()
.
Toggle Checkboxes Using the .prop()
Method
In jQuery, you can use the .prop()
method to set the properties of HTML elements. Here, you can use it on the checkboxes in your code to alternate their state between checked
and unchecked
.
The next example shows you how it’s done; in it, we have an HTML and jQuery code. The HTML contains checkboxes, and clicking on the first one checks them all.
We added a click
event to the first checkbox in the jQuery code. Afterward, we manipulate the checked
property of the remaining checkboxes.
The first time you check the first checkbox, all checkboxes get checked. If you uncheck it, the remaining checkboxes return to their default state; unchecked
.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-toggle-checkboxes</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
form {
outline: 2px solid #1a1a1a;
width: 50%;
margin: 0 auto;
padding: 0.5em;
}
label {
display: block;
cursor: pointer;
font-size: 1.2em;
}
label:first-child:hover {
background-color: #aaa;
}
</style>
</head>
<body>
<main>
<form id="form">
<label>
<input type="checkbox" id="select-lang"> Select all languages
</label>
<label>
<input type="checkbox" class="tech"> Cascading Style Sheets
</label>
<label>
<input type="checkbox" class="tech"> Hypertext Markup Language
</label>
</form>
</main>
<script>
$(document).ready(function() {
$("#select-lang").click(function() {
let check_boxes = $("input.tech");
// Swap the "prop" attribute each time the
// user clicks the "select-lang" checkbox.
check_boxes.prop("checked", !check_boxes.prop("checked"));
});
});
</script>
</body>
</html>
Output:
Toggle Checkboxes Using the .each()
Method
The.each()
method to toggle checkboxes works like what we showed with .prop()
. But, there is a difference; we use a function to iterate over each checkbox.
During this iteration, we changed the state of the checkboxes from checked
to unchecked
. The following code shows you how it’s done.
Also, we’ve updated the HTML from the first example; this time, the checkboxes contain the name of countries.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-toggle-checkboxes</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
form {
outline: 2px solid #1a1a1a;
width: 50%;
margin: 0 auto;
padding: 0.5em;
}
label {
display: block;
cursor: pointer;
font-size: 1.2em;
}
label:first-child:hover {
background-color: #aaa;
}
</style>
</head>
<body>
<main>
<form id="form">
<label>
<input type="checkbox" id="select-country"> Select all countries
</label>
<label>
<input type="checkbox" class="country"> Georgia
</label>
<label>
<input type="checkbox" class="country"> Turkmenistan
</label>
<label>
<input type="checkbox" class="country"> Azerbaijan
</label>
</form>
</main>
<script>
$(document).ready(function() {
$("#select-country").click(function() {
// Use the ".each()" API to iterate over the
// countries and alternate them between "checked"
// and "unchecked".
$('input.country').each(function () {
this.checked = !this.checked;
});
});
});
</script>
</body>
</html>
Output:
Toggle Checkboxes Using the .trigger()
Method
Using the .trigger()
method to toggle checkboxes works differently compared to using .prop()
and .each()
. This time, we use the .trigger()
method to execute a click function on all checkboxes when you click the first checkbox.
Another way is to call the .click()
method on each checkbox; both works fine.
But we left that as a comment in the code, and you can try it out at a later time. The HTML remains the same, but the checkboxes now contain the name of top websites on the internet.
You’ll find all these in the code block below, and the output in a browser follows.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>03-toggle-checkboxes</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
form {
outline: 2px solid #1a1a1a;
width: 50%;
margin: 0 auto;
padding: 0.5em;
}
label {
display: block;
cursor: pointer;
font-size: 1.2em;
}
label:first-child:hover {
background-color: #aaa;
}
</style>
</head>
<body>
<main>
<form id="form">
<label>
<input type="checkbox" id="select-websites"> Select all websites
</label>
<label>
<input type="checkbox" class="website"> Facebook
</label>
<label>
<input type="checkbox" class="website"> Amazon
</label>
<label>
<input type="checkbox" class="website"> Apple
</label>
<label>
<input type="checkbox" class="website"> Netflix
</label>
<label>
<input type="checkbox" class="website"> Google
</label>
</form>
</main>
<script>
$(document).ready(function() {
$("#select-websites").click(function() {
// Use the ".trigger()"" API to execute the
// click function on each checkbox
$('input.website').trigger('click');
// Another option is to execute the "click"
// API directly on the checkboxes
// $('input.website').click();
});
});
</script>
</body>
</html>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn