How to Check if Checkbox Is Checked With jQuery
-
Check if Checkbox Is Checked With Direct DOM Operations Using the
.get()
Method -
Check if Checkbox Is Checked With the
.prop()
Method -
Check if Checkbox Is Checked With the
.is()
Method - Common Use Cases for Checking if Checkbox Is Checked With jQuery
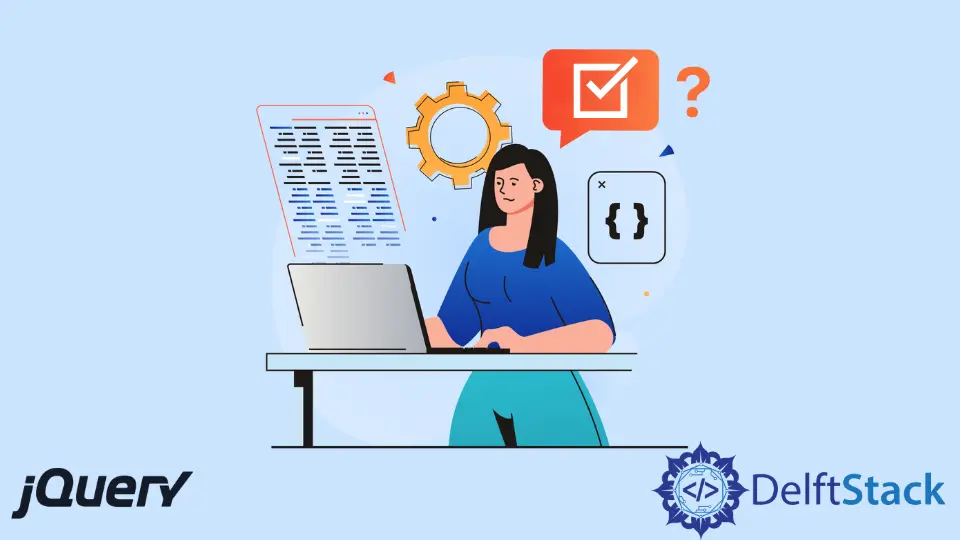
You will learn several methods to jQuery checkbox checked in this blog post. We will also discuss their performance metrics to let you choose the best method for each use case.
We will show useful shortcuts for common checkbox use cases. We also have a gotcha for a common error people make.
Check if Checkbox Is Checked With Direct DOM Operations Using the .get()
Method
jQuery lets us access the DOM node beneath each jQuery object with the .get()
method. We can then query this DOM node to directly access/set different properties.
$("button").on("click",function(){
$("p").html(`<p>
${$("input[name='checkboxArray']").get(0).checked?'The first box is checked':'The first box is not checked'}
// <p/>`);
});
You can see the working demo of this code below.
Let us break down this code. (Please see the attached HTML and CSS files to understand the code better.)
We select a button to trigger the check event and attach a callback on the click
event to it. In the callback, we display the check result by adding it as the text to a p
element; we use the jQuery .html()
method.
Note this jQuery method overwrites the earlier text, which is our desired use case here. We want a fresh HTML output for every new check.
The main check logic is in the line that we pass to the .html()
method. We first select all input elements with name = 'checkboxArray'
in a jQuery object.
Note that the selector only returns a jQuery object, and we do not have access to the underlying DOM node yet.
But we then chain the .get
method , like so - .get(0)
. We now have access to the first DOM node in the jQuery selected collection.
We will check if the first checkbox is checked, and so we access the first DOM node by passing in 0
as the argument to the .get()
method.
We now can directly access the .checked
property to jQuery to check if the checkbox is selected.
Pro Tip 1:
The .checked
property we access above is the JavaScript property attached to the DOM node (or, technically, the IDL property). It is different from the HTML checked
attribute that only displays the HTML element’s initial or default checked/unchecked value (technically the HTML content property).
We will look at this difference in detail later in this blog post.
Pro Tip 2:
Direct DOM operations are super fast. So, this method lets use jQuery to check if the checkbox is checked with superior performance.
Shortcut Check if Checkbox Is Checked With Direct DOM Operations Using the Dereferencing Operator
Each jQuery behaves like an array object. So, we can latch on to this behavior and reduce some code using the short array dereferencing operator []
instead of the .get
method.
$("button").on("click",function(){
$("p").html(`<p>
// ${$("input[name='checkboxArray']")[0].checked?'The first box is checked':'The first box is not checked'}
// <p/>`);
});
The code is the same as above except the use of the dereferencing operator: ... $("input[name = 'checkboxArray']")[0]...
.
Check if Checkbox Is Checked With the .prop()
Method
We can also use the jQuery .prop()
method to extract the underlying JavaScript properties from elements instead of directly accessing the DOM node.
$("button").on("click",function(){
$("p").html(`<p>
${$("input[name='checkboxArray']").prop('checked')?'The first box is checked':'The first box is not checked'}
<p/>`);
});
You can see the working demo of this code below.
Most of the code is similar to the above. Let us look at the line with the key difference, that is:
$("input[name='checkboxArray']").prop('checked')...
We extract the same .checked
property above, but use the jQuery .prop()
method here.
Pro Tip:
Why use jQuery .prop()
method? The jQuery .prop()
method is also a fast operation (compared to the selector-based method we see next).
It is good practice to query DOM element properties with JavaScript or JS-based libraries like jQuery.
Check if Checkbox Is Checked With the .is()
Method
We combine the :checked
selector with the .is()
method to use jQuery to check if the checkbox is checked.
The :checked
selector returns all selected or checked elements. For checkboxes, it means it selects those boxes that are checked.
$("some selector:checked");
// returns those elements that are checked
You can read more about the :checked
selector here.
The .is()
method matches a jQuery object against a selector and returns true if it matches that selector.
$("some jQuery object").is("some_selector");
//returns true if the jQuery object matches the selector
You can read more about the .is()
method here.
Let us combine the two into one code to check if the checkbox is checked with jQuery.
$("button").on("click",function(){
$("p").html(`<p>
${$("input[id='#first']").is(":checked")?'The first box is checked':'The first box is not checked'}
<p/>`);
});
Watch the demo of this method below.
The code to set up the event handler and the callback is similar to the earlier methods. The main change is in the implementation of the check, like so:
$("input[id = '#first']").is(":checked")...
The .is()
method returns true if the checkbox with the first id is checked because we pass the :checked
selector as the argument to it.
Pro Tip:
The is()
method does not make a new jQuery object or modify the original object in place. This behavior makes it ideal to use in callbacks and event handlers.
Common Use Cases for Checking if Checkbox Is Checked With jQuery
Now that we have seen the basic methods, let us build some ready-made quick scripts for common use cases.
Check if Checkbox Is Checked Using the Element’s ID
We can make the check using the element’s ID with the following code:
$("button").on("click",function(){
$("p").html(`<p>
${$("input[id='#second']").is(":checked")?'The second box is checked':'The secong box is not checked'}
<p/>`);
});
We select the checkbox with the ID second using the attribute equals selector, passing the id='#second'
option.
You can see the working demonstration below.
Check if Checkbox Is Checked Using Class
We can also select a group of checkboxes with a common class to see if they are checked. The code is pretty straightforward:
$("button").on("click",function(){
$("p").html(' ');
$("input[class='non-first']").each(function(){
$("p").append(`
${$(this).is(":checked")? 'The ' + $(this).attr("id") + ' box is checked':'The ' + $(this).attr("id") +' box is not checked'}
<br> `);
});
});
We select all the checkboxes with the non-first class and then iterate over each of them with the .each()
method. Finally, we run the check for each iteration and add the output to the p
element.
Note that we use the append()
method for this use case, as we do not want to overwrite the output for the previous iteration.
You can see the working demo below:
Uncheck the Checkbox if Checked With jQuery
We use the following snippet to uncheck a checkbox if checked with jQuery:
$("button").on("click",function(){
// To find the checked boxes by ID
let $checkedBox = $("input[id ='first']");
// To run the check and toggle if the checkbox is checked
if ($checkedBox.is(':checked')){
$checkedBox.prop("checked", false);
}
});
We use the set
form of the .prop()
method here.
$(some_jQuery_object).prop("checked",false);
// sets the "checked" property to false for the selected jQuery object
You can see the working demo of this snippet here:
Check if All Checkboxes Are Checked With jQuery
We can use the following boilerplate to find if all checkboxes in a given collection are checked.
$("button").on("click",function(){
let result = true;
// To find the list of checked boxes in an array of related checkboxes
let $checkedList = $("input[name='checkboxArray']");
// To iterate and do something with each checked box on the list above
// Here we display info related to them in the DOM
$checkedList.each(function(){
if (!($(this).is(":checked"))){
result = false;
}
});
// to display the number of checked boxes
$("p").html(`All checkboxes are ${result?'':'not'} checked`);
});
You can see the working demo of the snippet here:
Let us break down the logic for this code.
We attach the event handler like above and iterate over each checked box in the collection. We then run the .each()
function on each checkbox and change the result
variable to false if any checkboxes are not checked.
Finally, we output the result.
Check if Any Checkbox Is Checked in the Given Collection With jQuery
We can check if at least one of the checkboxes is checked with the following code:
$("button").on("click",function(){
let result = false;
// To find the list of checked boxes in an array of related checkboxes
let $checkedList = $("input[name='checkboxArray']");
// To iterate and do something with each checked box on the list above
// Here we display info related to them in the DOM
$checkedList.each(function(){
if ($(this).is(":checked")){
result = true;
}
});
// to display if any (at least) one of the checkboxes is checked
$("p").html(`At least one checkbox in the given collection is ${result?'':'not'} checked`);
});
Let us first see the method in action with the working demonstration below.
The logic is simple. We run the check on every checkbox in the collection, and we change the result
variable to true if even one of the checkboxes is checked.
Otherwise, we keep the result
variable’s value to false and output the message accordingly.
Disable Submit Button if Checkbox Is Unchecked With jQuery
We can write a simple snippet to disable a submit button if a specific checkbox is not checked.
$("button").on("click",function(){
// To find the specific checkbox
let $checkedBox = $("input[id='third']");
let $submitBtn = $("input[name='submitBtn']");
if ($($checkedBox).is(":checked")){
$submitBtn.prop("disabled",false);
}
else {
$submitBtn.prop("disabled",true);
}
});
You can see the output of this solution below:
The logic is simple. We determine if the checkbox is checked with the .is()
method and the :checked
selector, just like our methods above.
If the checkbox is checked, we set the disabled
property of the submitBtn
element to false; else, we set it to true.
Deprecated Use of the .attr()
Method for Checking if Checkbox Is Checked With jQuery
HTML attributes are different from DOM properties. Many times, properties match the HTML attribute, but not always.
Like in the case of checkboxes, the HTML checked
attribute is only the indicator of the element’s default or initial checked/unchecked state. It does not correspond with the checked
DOM property, which shows the element’s current checked/unchecked state.
The jQuery .attr()
method only reference HTML attributes. So, you should not use this method to find if the checkbox is selected.
You could do this with jQuery earlier, but the behavior changed in version 1.6 when the .prop()
method was added to access DOM properties explicitly and .attr()
was restricted to only HTML attributes.
You can read, in detail, about how jQuery handles attributes and properties differently here.