How to Zip Two Arrays in JavaScript
-
Use
map()
Method to Zip Two Arrays in JavaScript -
Use
Array.from()
Method to Zip Two Arrays in JavaScript -
Use
Array.prototype.fill()
Method to Zip Two Arrays
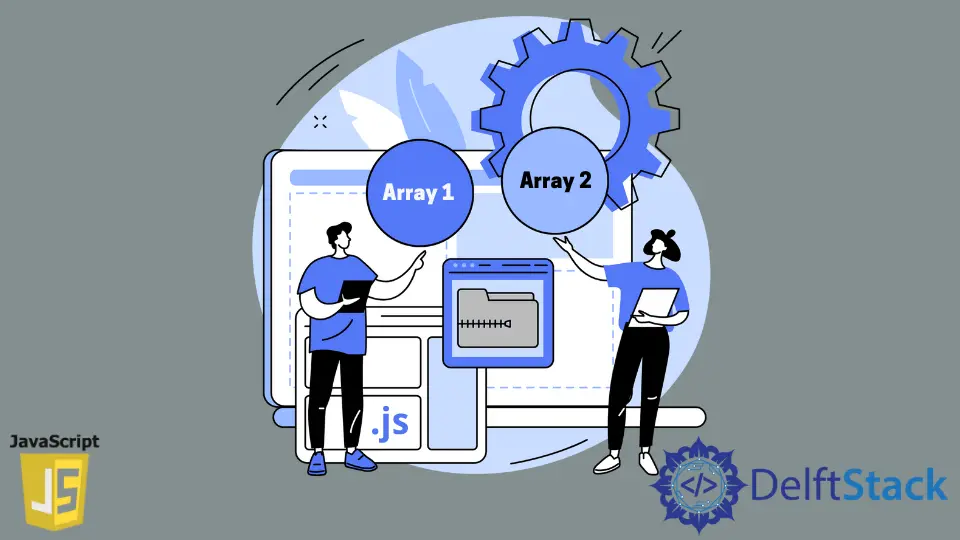
JavaScript has the map()
method to zip two arrays of a certain length. But if the corresponding two array does not match each other’s length, then the minimum lengthen array prints undefined
as its match.
Here along with the map()
method, we will see a demonstration of the Array.form()
method and also the Array.prototype.fill()
method, which has an explicit declaration of what to do when array lengths do not match.
Use map()
Method to Zip Two Arrays in JavaScript
The map()
function takes a call-back function that will call the x
array elements and map them with the elements of the y
array. This simple way of zipping is effortless to grasp as well.
Code Snippet:
var x = [1, 2, 3];
var y = ['10', '20', '30'];
var z = x.map(function(e, i) {
return [e, y[i]];
});
console.log(z);
Output:
Use Array.from()
Method to Zip Two Arrays in JavaScript
In the instance for the Array.from()
method, we will have two arrays and pass them in an arrow function. The function will first match the length and then map the elements from two different arrays. Also, when any element is found missing, the corresponding map will print undefined
for that.
Code Snippet:
var x = [1, 2, 3, 4];
var y = ['10', '20', '30'];
var zip = (x, y) =>
Array.from(Array(Math.max(x.length, y.length)), (_, i) => [x[i], y[i]]);
console.log(zip(x, y));
Output:
Use Array.prototype.fill()
Method to Zip Two Arrays
The method Array.prototype.fill()
works similar to the Array.from()
method and outputs as expected.
Code Snippet:
var x = [1, 2, 3];
var y = ['10', '20', '30', '40'];
const zip = (x, y) =>
Array(Math.max(x.length, y.length)).fill().map((_, i) => [x[i], y[i]]);
console.log(zip(x, y));
Output:
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript