How to Truncate a String in JavaScript
-
Use
substr()
Method to Truncate a String in JavaScript -
Use
split()
andjoin()
Method to Truncate String in JavaScript
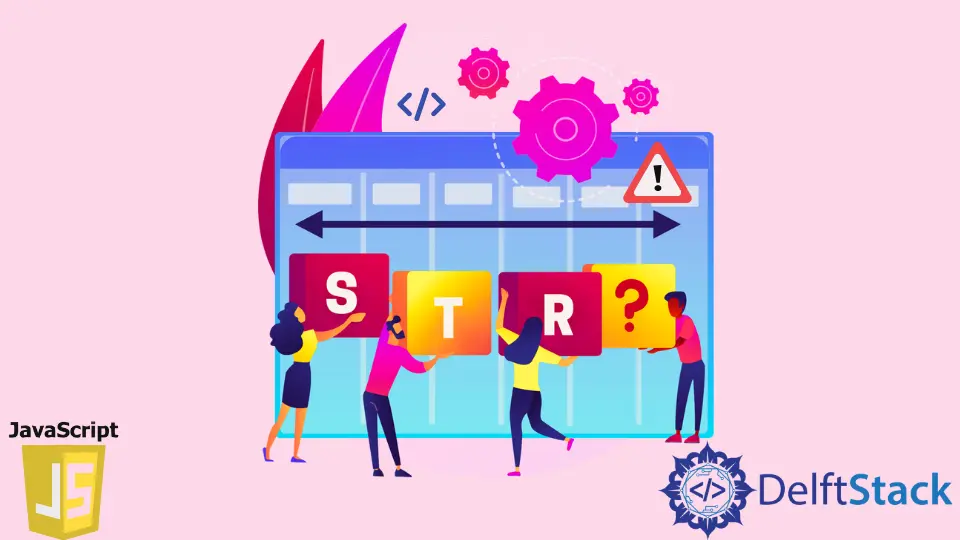
The floor()
, ceil()
, round()
, and trunc()
functions deal with numbers or any float numbers, but in the case of the string
data type, there is no direct function to truncate or round till a limit.
The common drive introduces the substr()
method that cuts a sentence, a.k.a string, up to a limit. This method draws out a substring from the stated string.
We will discuss the ways of using the mentioned JavaScript functions and methods.
Use substr()
Method to Truncate a String in JavaScript
The substr()
method takes two arguments to function the process.
The first parameter takes the start point (index
) from where the truncation will start, and the latter parameter is to set the limit till which index the truncation will stop.
It is to be mentioned that the truncation terminates before the limit
parameter. We will examine the line as we follow the instances.
Code Snippet:
function truncate(str, limit) {
if (str.length > limit) {
str = str.substr(0, limit);
} else {
return str;
}
return str;
}
console.log(truncate('Scooby-doo this crap', 10));
Output:
We noticed that the first parameter for the method was 0
while the limit was set to 10
. So, the output prints the characters up to the 9th
index.
If we only had one parameter for the method, it would infer as the start index and print the string until the end index. For the string we have in the example, if we have substr(10)
, we will get the outcome (whitespace)this crap
.
Use split()
and join()
Method to Truncate String in JavaScript
The manual way to truncate a string is by splitting a string into an array and getting the expected substring or truncated string. The example shows how a string is split
into an array and truncate
, join
and print
.
Code Snippet:
var str = 'I like to move it move it!';
str = str.split('');
var newStr = [];
var limit = 10;
for (var i = 0; i < limit; i++) {
newStr[i] = str[i];
}
newStr = newStr.join('');
console.log(newStr);
Output:
The str
object has a string stored, and we have reinitialized the str
to save the array form after applying the spilt()
method. We can calculate the array’s length to perform str.length
.
After that, we initiated a for
loop, setting the counter from the 0
index and ending until the limit value. You can select an index of preference as the start point of truncation.
The next task is to reallocate the str
array characters to the newStr
array before joining the newStr
and printing the console’s result. Our limit was set to index 10
, and the last printed character was the 9th
index.