Trigger Click Event in JavaScript
-
Trigger Click Event in JavaScript Using
click()
-
Trigger Click Event in JavaScript Using
addEventListener()
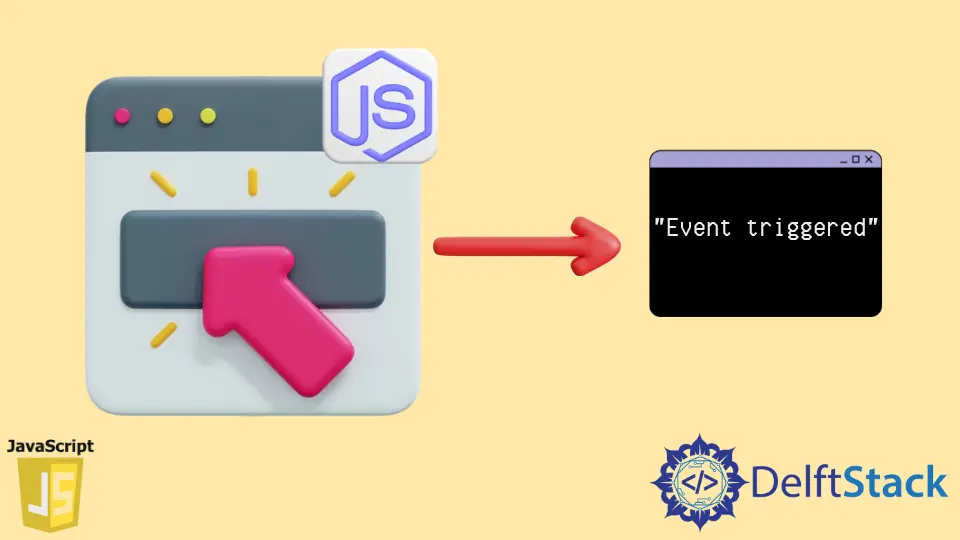
The window object is one of the most important objects used to communicate with the browser. It represents the browser’s window. All global variables and functions become members of the window object. The Window-Location object is used to get the URL of the current page and also to change the URL for the redirect.
Today’s article shows how to trigger a click event in JavaScript.
Trigger Click Event in JavaScript Using click()
This is a built-in event provided by JavaScript. An element receives the click event when pressed, and a key is released on the pointing device (eg, the left mouse button) while the pointer is within the element.
click()
is triggered after the down and up mouse events are triggered in that order. Further information on the click
function can be found in the documentation for the method click()
.
<button type="button" id="btn" onclick="openGoogleByMethod()">Open Google</button>
const link = document.getElementById('btn');
for (let i = 0; i < 5; i++) {
link.click();
}
function openGoogleByMethod() {
console.log('Event triggered')
}
We extract the element using the element ID in the example above and apply the Click event to an element. If you want to run an automated click event more than once, you can write the for loop and call target.click()
inside the for
loop. It automatically invokes the click of the button until the counter reaches its maximum value and outputs the Triggered event
5 times.
Output:
"Event triggered"
"Event triggered"
"Event triggered"
"Event triggered"
"Event triggered"
Trigger Click Event in JavaScript Using addEventListener()
This is an integral method provided by the JavaScript Event target interface. This method registers an event listener. Whenever the specified event is caught on the target, our configured function is called.
Syntax
target.addEventListener($type, $listener);
target.addEventListener($type, $listener, $options);
target.addEventListener($type, $listener, $useCapture);
$type
is a mandatory parameter that accepts only a string indicating the type of event to be monitored. It is a case-sensitive parameter. It supports various events likeclick
,keyboard
,input
,database
, etc.$listener
is a mandatory parameter. When an event of the specified type occurs, this parameter as an object receives a notification about it. This object must implement the EventListener interface or a JavaScript function.$options
is an optional parameter. This parameter specifies the properties of the event listener. Some properties arecapture
,once
,passive
, andsignal
.$useCapture
is an optional parameter. This parameter accepts Boolean values. Events of this type will be first sent to an EventTarget at the bottom of the DOM tree or not will be decided by this boolean value. If true, this event is first sent to the registered listener and then to EventTarget.
Further information on the addEventListener function can be found in the documentation for the method addEventListener()
.
<button type="button" id="btn" onclick="openGoogleByMethod()">Open Google</button>
const link = document.getElementById('btn');
link.addEventListener('click', e => {});
for (let i = 0; i < 5; i++) {
link.dispatchEvent(new Event('click'));
}
function openGoogleByMethod() {
console.log('Event triggered')
}
In the above example, we extract the element using the element ID and add a lister for the Click event on an element. If you want to run an automated click event more than once, you can type for loop and enter target.DispatchEvent (new Event('click'))
method inside the for loop. dispatchEvent
is the EventTarget
method that dispatches the event object followed by the event listener. This will automatically send the button click event until the counter reaches its maximum value and prints the Triggered Event
5 times.
Output:
"Event triggered"
"Event triggered"
"Event triggered"
"Event triggered"
"Event triggered"
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn