How to Sum Array of Objects in JavaScript
-
Method 1: Using the
reduce()
Function - Method 2: Using a forEach Loop
- Method 3: Using a for…of Loop
- Conclusion
- FAQ
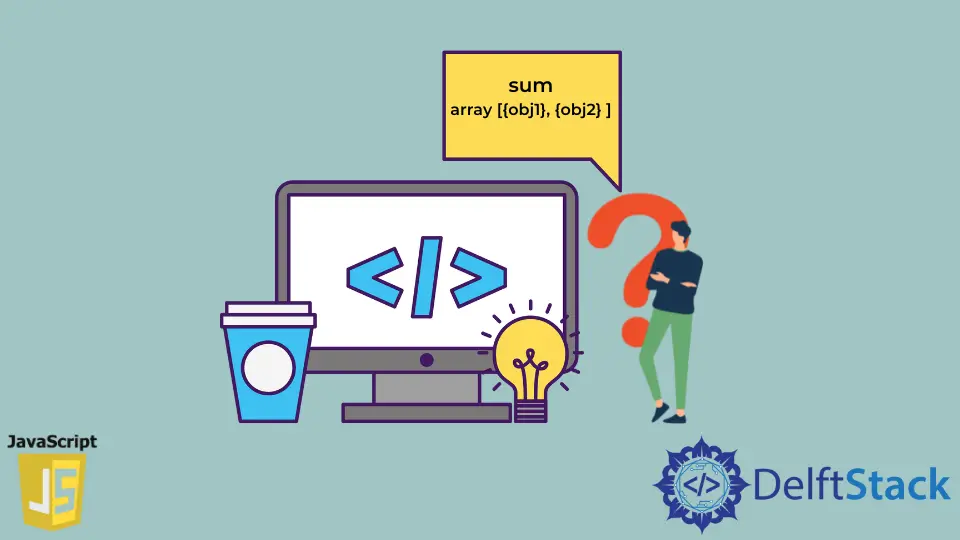
In the world of JavaScript, working with arrays of objects is a common task, especially when you’re dealing with data from APIs or databases. One frequent requirement is to sum specific properties within these objects. Whether you’re calculating total sales from an array of product objects or summing scores from a list of students, knowing how to efficiently sum values in an array of objects is essential.
This tutorial will guide you through various methods to achieve this using JavaScript, enhancing your coding skills and enabling you to handle data more effectively.
Method 1: Using the reduce()
Function
The reduce()
function is a powerful tool in JavaScript that allows you to accumulate values from an array. When summing properties from an array of objects, reduce()
is often the go-to method. Here’s how you can use it to sum a specific property, such as amount
, from an array of objects.
const data = [
{ id: 1, amount: 100 },
{ id: 2, amount: 200 },
{ id: 3, amount: 300 },
];
const totalAmount = data.reduce((accumulator, current) => accumulator + current.amount, 0);
console.log(totalAmount);
Output:
600
In this example, we have an array called data
, containing objects with an id
and an amount
. The reduce()
function iterates over each object, using an accumulator to keep a running total of the amount
property. The initial value of the accumulator is set to 0
. By the end of the iteration, totalAmount
holds the sum of all amount
values, which, in this case, is 600
.
Method 2: Using a forEach Loop
Another straightforward approach to summing values in an array of objects is by using a forEach
loop. This method is particularly useful if you prefer a more explicit iteration process. Here’s how you can implement it:
const data = [
{ id: 1, amount: 100 },
{ id: 2, amount: 200 },
{ id: 3, amount: 300 },
];
let totalAmount = 0;
data.forEach(item => {
totalAmount += item.amount;
});
console.log(totalAmount);
Output:
600
In this code, we declare a variable totalAmount
initialized to 0
. The forEach
method iterates through each object in the data
array. For each object, we add the amount
property to totalAmount
. By the end of the loop, totalAmount
contains the total sum of all amount
values, which again equals 600
. This method is clear and easy to understand, making it a good choice for those who prefer readability.
Method 3: Using a for…of Loop
If you prefer a more traditional approach, a for...of
loop can also be used to sum values in an array of objects. This method can be particularly handy when you want to have more control over the iteration process. Here’s how to do it:
const data = [
{ id: 1, amount: 100 },
{ id: 2, amount: 200 },
{ id: 3, amount: 300 },
];
let totalAmount = 0;
for (const item of data) {
totalAmount += item.amount;
}
console.log(totalAmount);
Output:
600
In this example, we again initialize totalAmount
to 0
. The for...of
loop iterates over each object in the data
array. For each object, we add the amount
property to totalAmount
. The final result is the same, with totalAmount
equaling 600
. This method is also straightforward and provides a clear structure for summing values.
Conclusion
Summing an array of objects in JavaScript is a fundamental skill that can significantly enhance your data manipulation capabilities. Whether you choose to use reduce()
, forEach
, or a for...of
loop, each method has its advantages and can be applied based on your coding style and requirements. By mastering these techniques, you’ll be well-equipped to handle various data-related tasks in your JavaScript projects. Keep practicing, and soon you’ll find yourself summing values in arrays with ease!
FAQ
-
What is the best method to sum an array of objects in JavaScript?
The best method depends on your preference;reduce()
is concise, whileforEach
andfor...of
are more explicit. -
Can I sum properties other than numbers in an array of objects?
No, you can only sum numerical properties. Other types will need conversion or different handling. -
Is it possible to sum multiple properties in an array of objects?
Yes, you can modify the summing logic to accumulate multiple properties within the same iteration. -
How can I sum values conditionally in an array of objects?
You can use methods likefilter()
before summing to conditionally include only certain objects. -
Are there performance implications for large arrays?
Yes, performance may vary based on the method used and the size of the array, withreduce()
generally being more efficient.