How to Strip Non-Numeric Characters From String in JavaScript
-
Regex
in JavaScript -
the
replace()
Method in JavaScript -
Using the
replace()
Method to Remove All the Non-Numeric Characters From a String in JavaScript -
the
match()
Method in JavaScript -
the
join()
Method in JavaScript
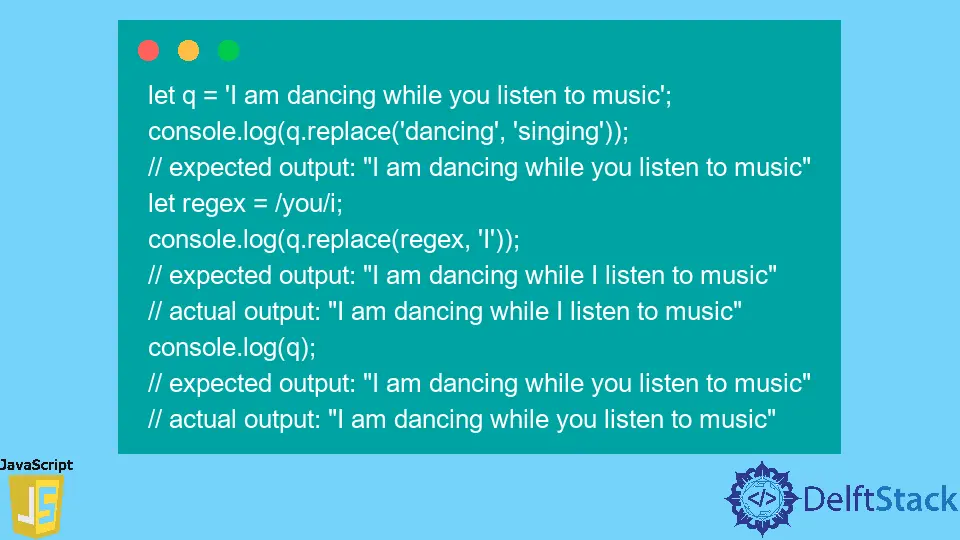
This article describes how they can remove non-numeric characters from a string variable. This article will explain the working of Regex and how it is used.
Regex
in JavaScript
Regex
stands for Regular expressions. Regex
are objects in JavaScript that consist of some special patterns that can help us match several string character combinations.
They can also break or split some characters. There are two different approaches to writing Regex in your program.
Syntax 1:
let re = /ab+c/;
Syntax 2:
let re = new RegExp('ab+c');
We use the constructor
function in this code segment for the expression. The expression can be changed during the runtime and compiled at the runtime.
the replace()
Method in JavaScript
The replace()
function creates a new string from the existing string where the matching patterns are stored. The original string remains the same and can be accessed after making a new string.
Example:
let q = 'I am dancing while you listen to music';
console.log(q.replace('dancing', 'singing'));
// expected output: "I am dancing while you listen to music"
let regex = /you/i;
console.log(q.replace(regex, 'I'));
// expected output: "I am dancing while I listen to music"
// actual output: "I am dancing while I listen to music"
console.log(q);
// expected output: "I am dancing while you listen to music"
// actual output: "I am dancing while you listen to music"
The output can be seen using this link or this image.
We defined a string variable naming q
. We replaced two words, dancing
and you
, with singing
and I
.
Then we displayed the resultant string, and the output is also shown with the code as comments.
Using the replace()
Method to Remove All the Non-Numeric Characters From a String in JavaScript
We will use the replace()
function on our string and only keep the integer values and remove the non-integer values.
Example:
let og = '-123.456abcdefgfijkl';
let updated = og.replace(/[^\d.-]/g, '');
console.log(updated);
// expected output: "-123.456"
For the output of this code segment, please click on the link, or you can see the image below for the output.
We used two string variables. The first one had some non-numeric characters that we wanted to separate, so we created another variable and used the replace()
method.
\d
is used for matching integers. However, /g
tells the expression to keep working after the first replacement.
the match()
Method in JavaScript
The match()
function matches the string with some Regular Expression and returns the string that matches with the Regular Expression. The original string remains unchanged.
However, this new string can be accessed and used anytime during the program execution. The string returned will be returned in an array.
Example:
var og = '-123.456abcdefgfijkl';
var updated = og.match(/\d/g);
console.log(updated);
The output of the code mentioned above segment can be accessed using this link.
the join()
Method in JavaScript
The join()
method combines the strings into one string. The join()
function joins two or more strings one after the other to look like a single string.
We have used this function on the output generated from the Match function, which will give us the joined single string.
Example:
var og = '-123.456abcdefgfijkl';
var updated = og.match(/[\d.-]/g);
var joined = updated.join('');
console.log(joined);
The output of the code mentioned above segment can be accessed using this link or in the image below.