How to Splice String in JavaScript
-
Use
splice()
Method With thesplit()
Method in JavaScript -
Use
splice()
Method With Spread Operator in JavaScript
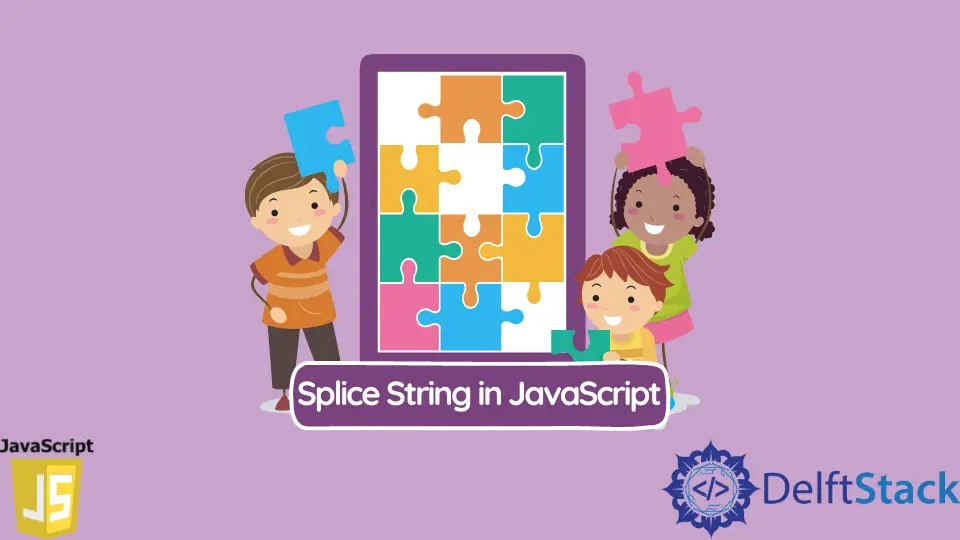
JavaScript has a similar method as splice()
that only lets accessing the element as an array. This method is slice()
, and this doesn’t perform anything other than accessing the string characters.
The splice()
effortlessly takes an argument for locating the index to be altered or removed and has a count
parameter that says how many characters will be removed.
Finally, a parameter that takes a string or number will be reallocated in the removal position. Here, we will see two examples of the splice()
method.
One will be splitting a string into an array using the split()
method. Another would be an example with the spread operator
.
Use splice()
Method With the split()
Method in JavaScript
We will split the string as an array in the following example with the split()
method.
Here, the splice()
method will take index
, count
, and add
arguments.
function spliceSplit(str, index, count, add) {
var array = str.split('');
array.splice(index, count, add);
return array.join('');
}
console.log(spliceSplit('I love you 3000', 11, 4, 42));
Output:
As you can see, the index
parameter points to the 11th
index, and the count
argument defines how many characters are to be removed.
The add
parameter will be set at the 11th index, and it is an optional case, so if you haven’t added it, the splice()
method will only remove characters based on index
and count
.
Use splice()
Method With Spread Operator in JavaScript
We will initiate a new object and store our string in an array form for this case. We will use the spread operator
here to convert the string to an array.
The rest of the mechanism of the splice()
method plays the same role. In the first example, we added a number, and here we will add a string.
var str = 'I\'m a happy soul in a happy world';
var len = str.length;
console.log(len);
var newStr = [...str];
newStr.splice(6, 5, 'sad');
newStr = newStr.join('');
console.log(newStr);
Output: