How to Sort Strings in JavaScript
-
Sort JavaScript Strings Using the
sort()
Method -
Sort JavaScript Strings Using the
localeCompare()
Method
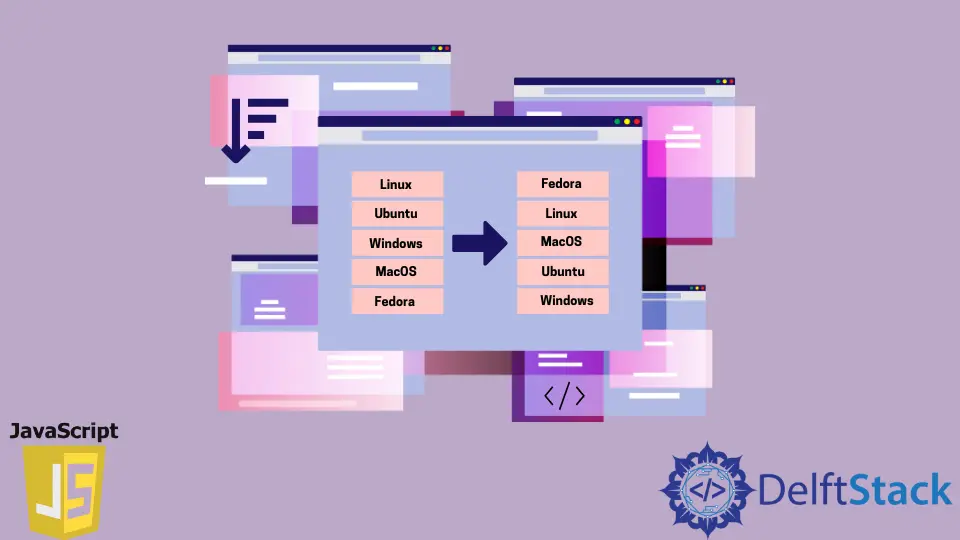
A JavaScript string contains zero or more quoted characters used to store and manipulate text.
When it comes to strings in JavaScript, there are some useful built-in methods to help us work with and manipulate them. This article shows you how to sort an array of strings using the built-in JavaScript methods.
JavaScript has two methods, sort
and localeCompare
, that return an ordered array of strings by mutating the original array of strings.
Sort JavaScript Strings Using the sort()
Method
The sort()
method is a built-in method provided by JavaScript that sorts the elements of an array.
This sorting is done by converting an element to strings. And based on that, it will compare their sequences of UTF-16
code units values.
The standard order is always ascending. This method mutates the original array.
Syntax:
sort()
sort((firstEl, secondEl) => {...})
sort(compareFn)
sort(function compareFn(firstEl, secondEl) {
...
})
The parameters firstEl
and secondEl
represent two elements of an array that needs to be compared and sorted. compareFn
is an optional function that defines the custom sorting function. The return value of this method is that it returns sorted array in-place, which means it mutates the original array and stores the result in the original array.
For more information, check out this documentation for the sort()
method.
const osItems = ['Linux', 'Ubuntu', 'Windows', 'MacOS', 'Fedora'];
osItems.sort();
console.log(osItems);
If we call sort()
, this will sort the original array osItems
according to UTF-16
code units, and it will store the result back into the osItems
array. When you execute the above code, it will give you the below output.
Output:
["Fedora", "Linux", "MacOS", "Ubuntu", "Windows"]
Sort JavaScript Strings Using the localeCompare()
Method
The localeCompare()
method is a built-in method provided by JavaScript.
This method checks whether a reference string comes before or after, matches the specified string in the sort order, and returns the number based on that. The actual result may differ if you pass the other locale.
Syntax:
localeCompare(compareString)
localeCompare(compareString, locales)
localeCompare(compareString, locales, options)
compareString
is a required parameter which is a string against which the reference string is compared.
locales
represent the language whose formatting conventions should be used, and it’s a completely optional parameter. Based on the comparison, it will return either 1
if reference string occurs after comparing string, -1
if reference string occurs before comparing string, and 0
if both the strings are equivalent.
For more information, check out this documentation for the localeCompare()
method.
The only advantage of using the localeCompare
method inside the sort
method is that it allows sorting the non-ASCII characters, like é and è. localeCompare
method’s locales and options parameter makes it more accurate.
const osItems = ['Linux', 'Ubuntu', 'Windows', 'MacOS', 'Fedora'];
osItems.sort((a, b) => a.localeCompare(b, 'en'));
console.log(osItems);
If we call a.localeCompare(b, 'en')
, this will compare the a
(reference string) from the original array, in this case osItems
, with b
(comparing string) using English locale. The interesting part here is that it only returns -1
, 1
, and 0
, and then the sort method will sort these strings.
When you execute the code block above, it will give you the following output.
Output:
["Fedora", "Linux", "MacOS", "Ubuntu", "Windows"]
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn