How to Sort Array of Object by Property in JavaScript
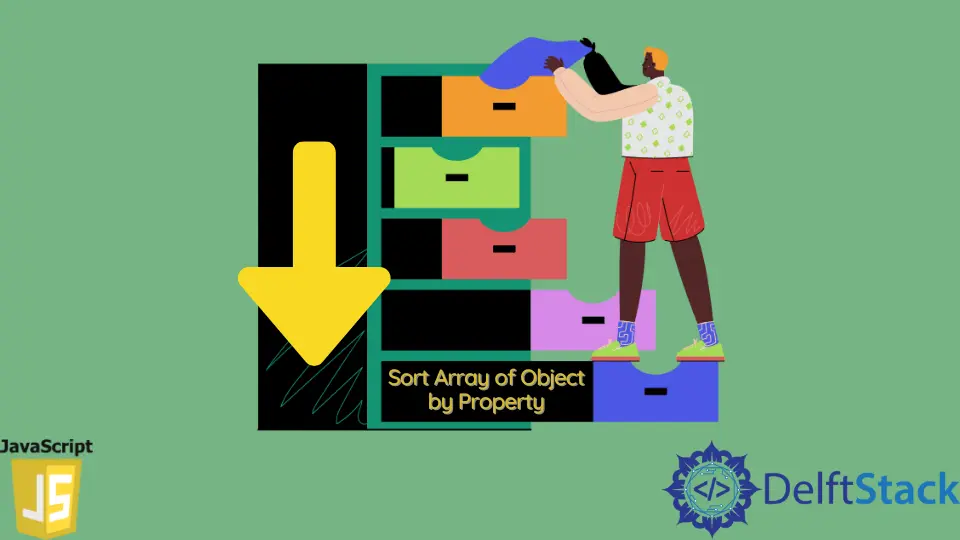
This article will discuss how to sort an array of objects based on the values of the object’s properties.
In JavaScript, we use the sort()
function to sort an array of objects. The sort()
function is used to sort the elements of an array alphabetically and not numerically. To get the items in reverse order, we may use the reverse()
method.
However, the traditional sort()
function may lag behind at times to compare an array of objects based on some property. So we can create a user-defined compare function to be used with the sort()
function. This method compares the properties of the items in an array.
The example below shows how to write your own comparison function.
let students = [
{fname: 'Rohan', lname: 'Dalal', age: 19},
{fname: 'Zain', lname: 'Ahmed', age: 17},
{fname: 'Anadi', lname: 'Malhotra', age: 19}
];
function compare_lname(a, b) {
if (a.lname.toLowerCase() < b.lname.toLowerCase()) {
return -1;
}
if (a.lname.toLowerCase() > b.lname.toLowerCase()) {
return 1;
}
return 0;
}
students.sort(compare_lname);
console.log(students)
Output:
[
{ fname: 'Zain', lname: 'Ahmed', age: 17 },
{ fname: 'Rohan', lname: 'Dalal', age: 19 },
{ fname: 'Anadi', lname: 'Malhotra', age: 19 }
]
In the above example, first, convert the lname
to lowercase and then we compare the names depending on the string comparison. It returns the sorted array of objects based on the lname
of the object. For descending order, we can replace the sort()
function with reverse()
.
If we are dealing with strings, we can eliminate the need to create a compare function and simply use the localeCompare()
function with the sort()
function to get the desired result.
See the code below.
let students = [
{fname: 'Rohan', lname: 'Dalal', age: 19},
{fname: 'Zain', lname: 'Ahmed', age: 21},
{fname: 'Anadi', lname: 'Malhotra', age: 16}
];
students.sort((a, b) => a.lname.localeCompare(b.lname));
console.log(students);
Output:
[
{ fname: 'Zain', lname: 'Ahmed', age: 21 },
{ fname: 'Rohan', lname: 'Dalal', age: 19 },
{ fname: 'Anadi', lname: 'Malhotra', age: 16 }
]
The localeCompare()
works only for strings. We cannot use this for numbers.
To get the sorted array based on some numerical property, we have to provide some compare function in the sort()
method because the sort()
method normally also does not work with numbers.
See the code below.
let students = [
{fname: 'Rohan', lname: 'Dalal', age: 19},
{fname: 'Zain', lname: 'Ahmed', age: 21},
{fname: 'Anadi', lname: 'Malhotra', age: 16}
];
students.sort((a, b) => {
return a.age - b.age;
});
console.log(students);
The above example compares the age of the objects and sorts them based on this age. The compare function is only a single line, so it is provided directly with the sort()
method. To get everything in descending order, use the reverse()
function.
Alternatively, we can also shift the order in the compare function to get the final output in descending order.
For example,
let students = [
{fname: 'Rohan', lname: 'Dalal', age: 19},
{fname: 'Zain', lname: 'Ahmed', age: 21},
{fname: 'Anadi', lname: 'Malhotra', age: 16}
];
students.sort((a, b) => {
return b.age - a.age;
});
console.log(students);
Output:
[
{ fname: 'Zain', lname: 'Ahmed', age: 21 },
{ fname: 'Rohan', lname: 'Dalal', age: 19 },
{ fname: 'Anadi', lname: 'Malhotra', age: 16 }
]
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript