How to Randomize or Shuffle an Array in JavaScript
- Shuffle an Array Depending on JavaScript Engine
- Measure the Randomness of Our Simple Algorithm
-
Shuffle an Array Using
Fisher-Yates
Shuffle Algorithm -
Shuffle an Array With the
Underscore.js
orLo-Dash
Library
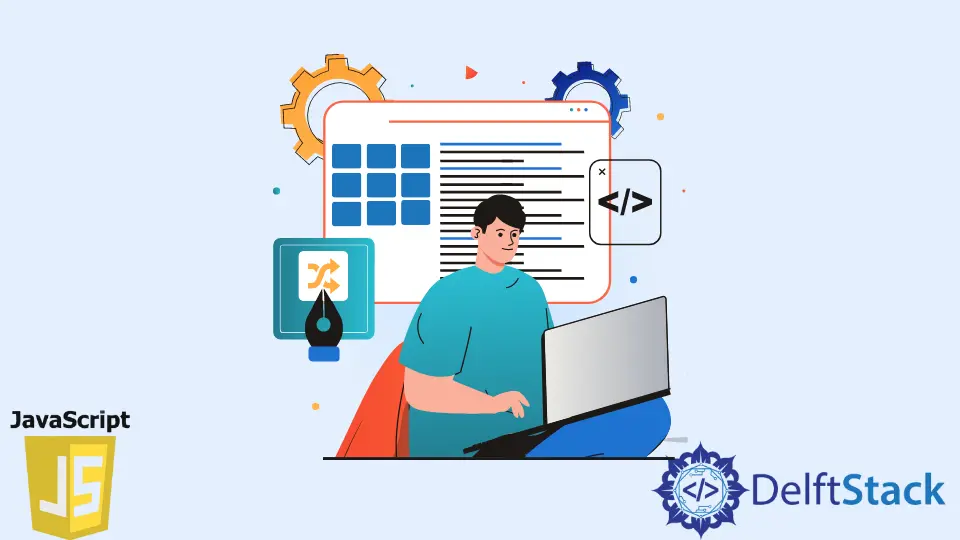
In this tutorial, we will learn how to shuffle or randomize an array in JavaScript; there are many ways to shuffle an array in JavaScriptwhether by implementing shuffling algorithms or using already existing shuffle functions in some libraries.
To shuffle an array is to arrange its element randomly, so it mainly depends on how you reorder or sort the array with a degree of randomness.
Let’s move forward and discover different ways to randomize or shuffle an array.
Shuffle an Array Depending on JavaScript Engine
Let’s start with implementing a simple array shuffling algorithm by sorting the array using array.sort()
but using some randomness generated by the equation Math.random() - 0.5
and -0.5
ensures that every time we call the algorithm, the random value can be positive or negative.
Let’s Implement that simple algorithm with the power of JavaScript engine and print the shuffled Array to console using Console.log()
:
function shuffleArray(inputArray) {
inputArray.sort(() => Math.random() - 0.5);
}
var demoArray = [1, 3, 5];
shuffleArray(demoArray);
console.log(demoArray);
Output:
[1, 5, 3]
Measure the Randomness of Our Simple Algorithm
The probability of that array’s permutations can be calculated to check how excellent and random our algorithm we have implemented.
Let’s see how we can measure its randomness.
- Create a dictionary that will count the appearance for all permutations.
- Create a loop that will run 1000000 times and every time will increase the count of the permutation formed
- Print the counts of all possible permutations and observe the probabilities between them.
This simple measuring algorithm can be implemented like the following:
function shuffleArray(inputArray) {
inputArray.sort(() => Math.random() - 0.5);
}
// counts of the appearances for all possible permutations
var countDic = {
'153': 0,
'135': 0,
'315': 0,
'351': 0,
'531': 0,
'513': 0,
};
// Creating the loop
for (var i = 0; i < 1000000; i++) {
var arr = [1, 5, 3];
shuffleArray(arr);
countDic[arr.join('')]++;
}
// Print the counts of all possible permutations
for (var key in countDic) {
console.log(`${key}: ${countDic[key]}`);
}
Output:
135: 62256
153: 375832
315: 62976
351: 311865
513: 124518
531: 62553
From the above output, we can see the bias clearly as 135
, 315
, and 531
appear much less than others and so similar counts to each other.
Shuffle an Array Using Fisher-Yates
Shuffle Algorithm
This simple algorithm based on the JavaScript engine is unreliable in the past section, but a great algorithm called Fisher-Yates
is better regarding its efficiency and reliability.
The idea behind the Fisher-Yates
Algorithm is to walk to the array in reverse order and swap each element with a random one before it. Fisher-Yates
is a simple but very efficient and fast algorithm.
Let’s implement Fisher-Yates
algorithm:
function fisherYatesShuffle(arr) {
for (var i = arr.length - 1; i > 0; i--) {
var j = Math.floor(Math.random() * (i + 1)); // random index
[arr[i], arr[j]] = [arr[j], arr[i]]; // swap
}
}
var tmpArray = [1, 3, 5];
fisherYatesShuffle(tmpArray);
console.log(tmpArray);
So let’s explain it step-by-step:
for(var i =array.length-1 ; i>0 ;i--)
a for loop that will walk on the array in a reverse order.Math.floor( Math.random() * (i + 1) )
Generating a random index that ranges between 0 and i.[arr[i],arr[j]]=[arr[j],arr[i]]
is swaping the elementsarr[i]
andarr[j]
with each other using theDestructuring Assignment
syntax.
Output:
(3) [3, 1, 5]
Now let’s test Fisher-Yates
the same we did before:
// counts of the appearances for all possible permutations
var countDic = {
'153': 0,
'135': 0,
'315': 0,
'351': 0,
'531': 0,
'513': 0,
};
// Creating the loop
for (var i = 0; i < 1000000; i++) {
var arr = [1, 5, 3];
fisherYatesShuffle(arr);
countDic[arr.join('')]++;
}
// Print the counts of all possible permutations
for (var key in countDic) {
console.log(`${key}: ${countDic[key]}`);
}
Output:
135: 166734
153: 166578
315: 166908
351: 166832
513: 166535
531: 166413
From the above output, you can see the big difference between Fisher-Yates
algorithm and the simple algorithm we implemented before and how reliable is Fisher-Yates
algorithm.
Shuffle an Array With the Underscore.js
or Lo-Dash
Library
The famous Underscore.js
library also provides a shuffle function that can directly randomize an array without the need to write your implementation of any algorithm.
Let’s see the following example of using the _.shuffle()
method.
First, We need to import the library using Cloudflare CDN
inside the HTML Template,
<script src="https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.17.15/lodash.min.js"></script>
Then we use the _.shuffle()
method like:
var tmpUnderscoreArray = [1, 3, 5];
resultArray = _.shuffle(tmpUnderscoreArray);
console.log(resultArray);
Output:
(3) [1, 5, 3]
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript