Nested for Loops JavaScript
- What are Nested Loops?
- Implementing Nested For Loops in JavaScript
- Performance Considerations
- Conclusion
- FAQ
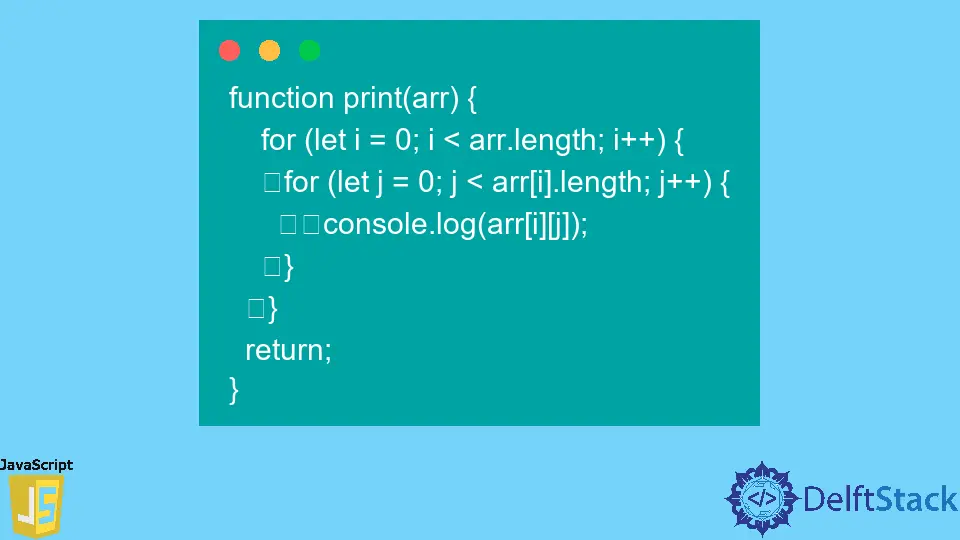
Understanding nested loops in JavaScript is crucial for any developer looking to enhance their programming skills. Nested loops allow you to iterate over complex data structures, such as multi-dimensional arrays, and perform operations that require multiple iterations.
This tutorial will walk you through the concept of nested loops, providing clear examples and explanations to ensure you grasp this essential programming concept. By the end of this article, you will be equipped with the knowledge to implement nested loops effectively in your JavaScript projects.
What are Nested Loops?
At its core, a nested loop is a loop inside another loop. In JavaScript, you can use any type of loop (for, while, or do…while) as a nested loop. The outer loop runs first, and for each iteration of the outer loop, the inner loop runs completely. This structure is particularly useful when dealing with multi-dimensional arrays or when you need to perform complex calculations that require multiple iterations.
For example, if you have a 2D array representing a grid, you can use nested loops to access each element of the grid. Let’s take a closer look at how to implement this in JavaScript.
Implementing Nested For Loops in JavaScript
Example 1: Iterating Over a 2D Array
Let’s say you have a 2D array that represents a grid of numbers. You want to print each number in the grid. Here’s how you can achieve this using nested for loops:
let grid = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
for (let i = 0; i < grid.length; i++) {
for (let j = 0; j < grid[i].length; j++) {
console.log(grid[i][j]);
}
}
Output:
1
2
3
4
5
6
7
8
9
In this example, the outer loop iterates through each row of the grid, while the inner loop iterates through each element in the current row. The result is that each number in the grid is printed to the console. This approach is efficient and straightforward, making it a popular choice for developers working with multi-dimensional arrays.
Example 2: Generating a Multiplication Table
Another practical application of nested loops is generating a multiplication table. This example will illustrate how you can create a simple multiplication table from 1 to 5 using nested for loops.
for (let i = 1; i <= 5; i++) {
let row = '';
for (let j = 1; j <= 5; j++) {
row += (i * j) + '\t';
}
console.log(row);
}
Output:
1 2 3 4 5
2 4 6 8 10
3 6 9 12 15
4 8 12 16 20
5 10 15 20 25
In this example, the outer loop represents the rows of the multiplication table, while the inner loop calculates the product of the current row and column indices. The result is a neatly formatted multiplication table printed to the console. This method is not only efficient but also demonstrates the power of nested loops in generating structured outputs.
Performance Considerations
While nested loops are powerful, they can also lead to performance issues, especially with large datasets. The time complexity of nested loops is typically O(n^2), meaning that the execution time increases exponentially with the size of the input. To mitigate performance concerns, consider the following strategies:
- Optimize Loop Conditions: Ensure that your loop conditions are as efficient as possible. Avoid unnecessary computations within the loop.
- Limit the Depth of Nesting: Try to keep the nesting to a minimum. If you find yourself nesting too deeply, it may be a sign that there’s a more efficient solution available.
- Use Built-In Methods: In many cases, JavaScript offers built-in methods (like
map
,filter
, andreduce
) that can replace the need for nested loops, improving both readability and performance.
By keeping these considerations in mind, you can effectively use nested loops without compromising the performance of your JavaScript applications.
Conclusion
Nested loops in JavaScript are a fundamental concept that every developer should understand. They allow for complex data manipulation and can be used in various applications, from iterating over multi-dimensional arrays to generating structured outputs like multiplication tables. While they are powerful, it’s essential to use them wisely to avoid performance pitfalls. By practicing with the examples provided, you can become proficient in implementing nested loops in your JavaScript projects.
FAQ
-
What is a nested loop in JavaScript?
A nested loop is a loop that exists within another loop, allowing for multiple iterations over data structures. -
When should I use nested loops?
Use nested loops when you need to iterate over multi-dimensional arrays or perform operations that require multiple iterations. -
Are nested loops efficient?
Nested loops can lead to performance issues with large datasets due to their time complexity. It’s essential to optimize them to avoid slow performance. -
Can I use other types of loops as nested loops?
Yes, you can use any type of loop (for, while, do…while) as a nested loop in JavaScript. -
How can I improve the performance of nested loops?
Optimize loop conditions, limit the depth of nesting, and consider using built-in JavaScript methods to improve performance.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn