How to Write a Multiline String in JavaScript
- Method 1: Using Template Literals
- Method 2: String Concatenation
- Method 3: Using Escape Characters
- Conclusion
- FAQ
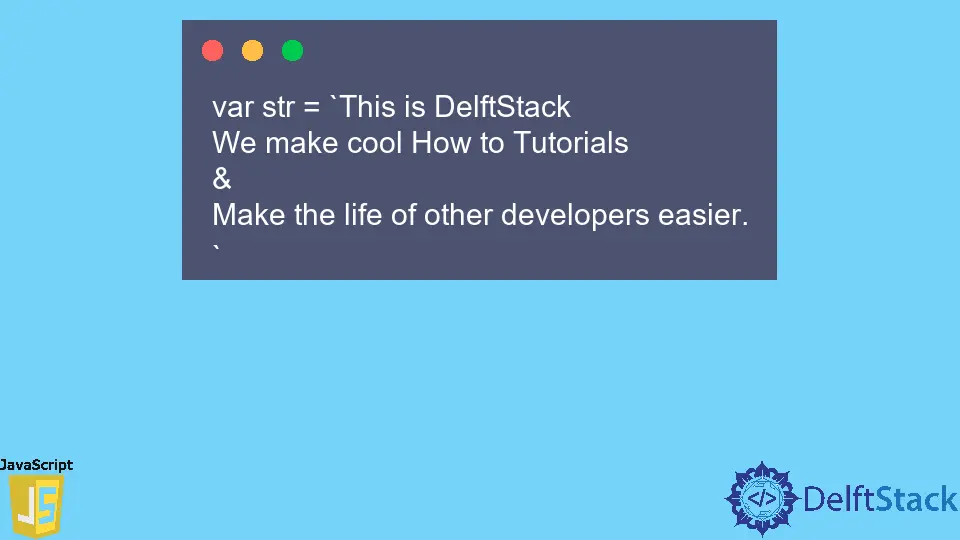
Creating multiline strings in JavaScript can be a bit tricky if you’re not familiar with the various methods available.
In this tutorial, we will explore different approaches to writing multiline strings in JavaScript. Whether you are a beginner or an experienced developer, understanding how to effectively manage multiline strings can enhance your coding efficiency and readability. We will cover template literals, string concatenation, and the traditional method using escape characters. By the end of this guide, you will be equipped with the knowledge to choose the best method for your specific needs.
Method 1: Using Template Literals
One of the most straightforward and modern ways to create multiline strings in JavaScript is by using template literals. Introduced in ES6, template literals allow you to embed expressions and create strings that span multiple lines without any additional syntax. To create a template literal, you simply use backticks (`) instead of single or double quotes.
Here’s how you can write a multiline string using template literals:
const multilineString = `This is a string
that spans multiple lines
using template literals.`;
console.log(multilineString);
Output:
This is a string
that spans multiple lines
using template literals.
Template literals not only allow for multiline strings but also enable string interpolation, making them incredibly versatile. You can easily include variables and expressions within the string by wrapping them in ${}
. This feature enhances readability and keeps your code clean. Additionally, template literals maintain the formatting of your strings, which is especially useful for generating HTML or other formatted text.
Method 2: String Concatenation
Before the advent of template literals, developers primarily relied on string concatenation to create multiline strings. This method involves using the +
operator to combine multiple strings into one. While this approach is less elegant than using template literals, it is still widely used, especially in older codebases.
Here’s an example of how to create a multiline string using string concatenation:
const multilineString = 'This is a string ' +
'that spans multiple lines ' +
'using string concatenation.';
console.log(multilineString);
Output:
This is a string that spans multiple lines using string concatenation.
In this example, each line of the string is enclosed in single quotes and concatenated using the +
operator. While this method works, it can become cumbersome and hard to read, especially when dealing with longer strings. It also requires careful attention to spacing, as you need to manually add spaces where necessary. Despite its drawbacks, string concatenation is still a valid approach and can be useful in certain scenarios.
Method 3: Using Escape Characters
Another traditional method for creating multiline strings in JavaScript is by using escape characters. This involves using the backslash (\
) at the end of a line to indicate that the string continues on the next line. While this method is less common today due to the availability of template literals, it can still be useful in specific situations.
Here’s how you can create a multiline string using escape characters:
const multilineString = 'This is a string \
that spans multiple lines \
using escape characters.';
console.log(multilineString);
Output:
This is a string that spans multiple lines using escape characters.
In this example, the backslash at the end of each line tells JavaScript to ignore the newline character and continue the string on the next line. While this method works, it can make the code less readable, especially with longer strings. It also requires careful placement of the backslash, and forgetting it can lead to syntax errors. Therefore, while using escape characters is a viable option, it is generally recommended to use template literals or string concatenation for better clarity and maintainability.
Conclusion
In conclusion, writing multiline strings in JavaScript can be accomplished through various methods, each with its advantages and disadvantages. Template literals are the most modern and versatile option, allowing for clear and concise multiline strings. String concatenation remains a valid approach, especially for older codebases, while escape characters offer a traditional method that is less commonly used today. By understanding these different techniques, you can choose the best approach for your coding needs and improve your overall coding efficiency.
FAQ
-
What are template literals in JavaScript?
Template literals are a feature introduced in ES6 that allow you to create strings using backticks and support multiline strings and string interpolation. -
Is string concatenation still relevant in JavaScript?
Yes, string concatenation is still relevant, especially in older codebases. However, template literals are generally preferred for new code due to their enhanced readability.
-
Can I use escape characters for multiline strings in JavaScript?
Yes, you can use escape characters to create multiline strings, but this method is less common and can reduce code readability. -
What is the best method for writing multiline strings?
The best method depends on your specific use case. For modern applications, template literals are recommended due to their simplicity and flexibility. -
Are there any performance differences between these methods?
In most cases, the performance differences are negligible. However, template literals may offer slight advantages in readability and maintainability, which can lead to better long-term performance in code quality.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn