How to Insert Variable Into String in JavaScript
- Use Template Literals to Insert Variable Into String in JavaScript
- Use Basic Formatting to Insert Variable Into String in JavaScript
-
Use
sprintf()
to Insert Variable Into String in JavaScript - Conclusion
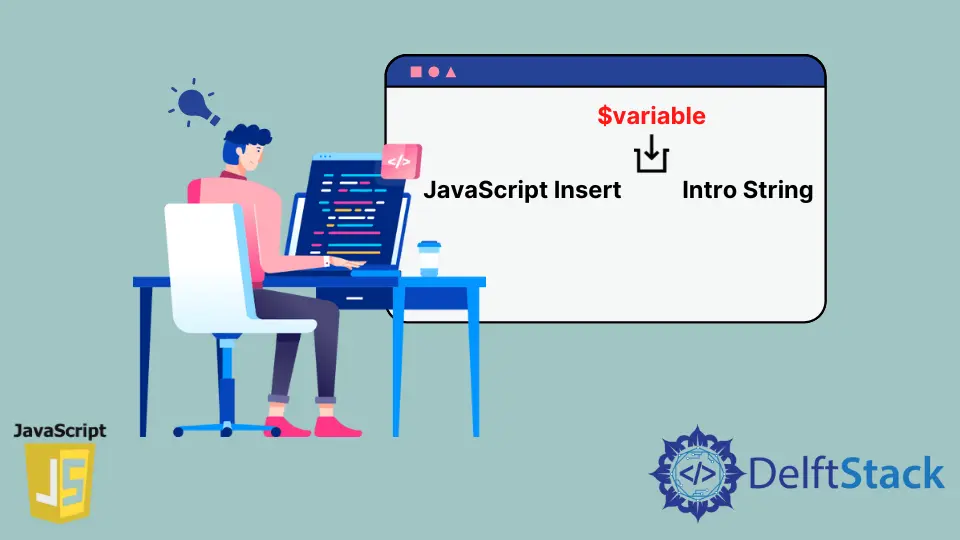
This article will introduce how to insert variable(s) into a string in JavaScript.
Use Template Literals to Insert Variable Into String in JavaScript
The concept of template literals has been around for a while in JavaScript. They were earlier known as template strings. Template literals
was introduced in ES6. It offers an easier way to embed variables in a given sentence than having to use cumbersome string concatenations. Instead of using quotes (double quotes or single quotes), we use template literals defined with the backticks (` character in keyboard). We place the string in the backticks and embed our variables in the sentence. Following are the usages of template literals.
Just including a string in a set of backticks by itself makes it a string value. It has the same functionality as holding the string text in double-quotes "
. The following sample code depicts a simple string used as a template literal.
console.log(`Hello World`);
Output:
Hello World
We have a normal string ready. If we have to display the value of a variable in this string, we need to embed variables. While embedding, we wrap the variable in curly braces with a $
sign preceding it. The syntax for embedding the variable value is ${variableName}
. The following piece of code has the eggCount
variable embedded in a usual string, with this entire content is encapsulated in backticks.
let eggCount = 20;
console.log(`On easter we decorted ${eggCount}` easter eggs);
Output:
On easter we decorted 20 easter eggs
Embedding Expressions Involving Variables
Unlike the mere string concatenation, with template literals, we can include expressions with more than one variable, similar to coding in JavaScript with the template literals. If we need to do certain operations like summing, getting substrings, including dates, etc., all these functionalities and much more can be embedded into a string with template literals. The following code set has a variety of use cases for doing so.
let a = 5;
b = 10;
console.log(`Sum of ${a} and ${b} is ${a + b}`);
Output:
Sum of 5 and 10 is 15
Another example involving date is as follows. Here we do not use any variables, but the expressions included in template literals work for themselves. It is the strings with javascript in continuation with having to use the double quotes or backticks over and over again as we would do with concatenating sentences:
console.log(`The date today is ${new Date().getDate()}-${
new Date().getMonth() + 1}-${new Date().getFullYear()}`);
Output:
The date today is 7-4-2021
Remarks
- Template literals offer one of the easiest ways to embed variables in a sentence.
- It also offers support for including expressions directly in the string and leave the compiler to resolve the calculations at run time.
- We can now easily include single quotes and double quotes in a string without using escape characters.
- Template literals make coding simple, concise, neat, and readable.
- The value of template literals can be assigned to a new variable. It can be considered as a new string holding the values of your variables.
Use Basic Formatting to Insert Variable Into String in JavaScript
Another way of neatly inserting the variable values into our string is by using the basic formatting supported in JavaScript. Using the JavaScript console.log()
, we can avoid concatenation and add placeholders in the targetted string. The values to be assigned are passed as arguments as shown below.
let a = 5;
b = 10;
console.log('Sum of %d and %d is %d.', a, b, (a + b));
Output:
Sum of 5 and 10 is 15.
This resolution of variables into values works only with console.log()
. Hence, we cannot use it for assigning to a different variable, and we can not use it to display the new resolved string in HTML UI.
Remarks
- We can use this method while logging with the
console.log()
function. Hence, this method will not work for assigning values to a variable. Therefore, it may not be worthy of scenarios other than logging. - While using the basic formatting, placeholders used in the string should be corresponding to the type of data passed as a parameter. For instance, for displaying a numeric value, the placeholder in the sentence should be
%d
for string data type it is%s
.
let op1 = 5, op2 = 10, operation = 'Sum';
console.log('%s of %d and %d is %d.', operation, op1, op2, (op1 + op2));
Output:
Sum of 5 and 10 is 15.
If we forget to consider the type of parameter passed, there is a type mismatch. JavaScript will try to typecast the variable, and it will add the resultant value to the string. The following code explains this phenomenon.
let op1 = 5, op2 = 10, operation = 'Sum';
console.log('%s of %d and %d is %d.', op1, op2, (op1 + op2), operation);
Output:
5 of 10 and 15 is NaN.
op1
is of the Number data type, and we are using the %s
to inject it into the final string. The %s
will auto type-cast the number to a string data type. This conversion goes smoothly. But for the operation
variable, the value is assigned to a placeholder that comes next in the sequence. This placeholder is %d
. Hence, here a string data type is being converted to a number. The conversion does not happen. Hence the result returned is NaN
, which we see as the end word of the output.
We can use expressions in the parameters, as we saw in the case of (op1 + op2)
. The javascript understands and resolves it as a sum accordingly.
In this case, we can not use Double quotes in the final string without using the escape characters. However, we can use single quotes if we are encapsulating the entire structure in double-quotes. See the following code.
let op1 = 5, op2 = 10, operation = 'Sum';
console.log('\'%s\' of %d and %d is %d.', operation, op1, op2, (op1 + op2));
Output:
'Sum' of 5 and 10 is 15.
Use sprintf()
to Insert Variable Into String in JavaScript
Before Template Literals
in ES6 of JavaScript, the development community followed the feature-rich library of sprintf.js
. The library has a method called sprintf()
. sprintf.js
is an open-source library for JavaScript. It has its implementations for Node.js
and browsers. For setup and configurations, you can visit their git-hub page. sprintf()
is used to include the variables in a string without using the string concatenation. The usage is as follows.
let sprintf = require('sprintf-js').sprintf;
let op1 = 5, op2 = 10, operation = 'Sum';
sprintf('%s of %d and %d is %d.', operation, op1, op2, (op1 + op2));
Output:
Sum of 5 and 10 is 15.
It has various useful special features as follows.
sprintf()
function allows to specify the variables in a different order.- Like the functionality of template literals,
sprintf()
resolves expressions encapsulated within strings. - It has support for various formats. Other than the standard string and number, the
sprintf-js
library supports boolean, binary formats etc., for outputting the variable values to a string. - Moreover, you can specify the arguments as an array using the
vsprintf()
method provided by the library. - It also allows passing object directly in the second argument and resolves the variables automatically.
There are many more features that this library offers. More details can be found in the Read.me
file specified in their GitHub link.
Conclusion
When it comes to including the variable values to your string without going for the tedious string concatenations using the +
and double quotes, your best option will be to go for Template Literals, the feature with backticks. If we require inserting values in the output string only for logging purposes, the console.log()
function of JavaScript is useful. It has the formatting options included by default. The sprintf.js
library is feature-rich and dominates the space as it offers much more than the Template Literals when it comes to embedding the variable values. But that comes at a small cost of getting familiarized with the library.