How to Transpose an Array in JavaScript
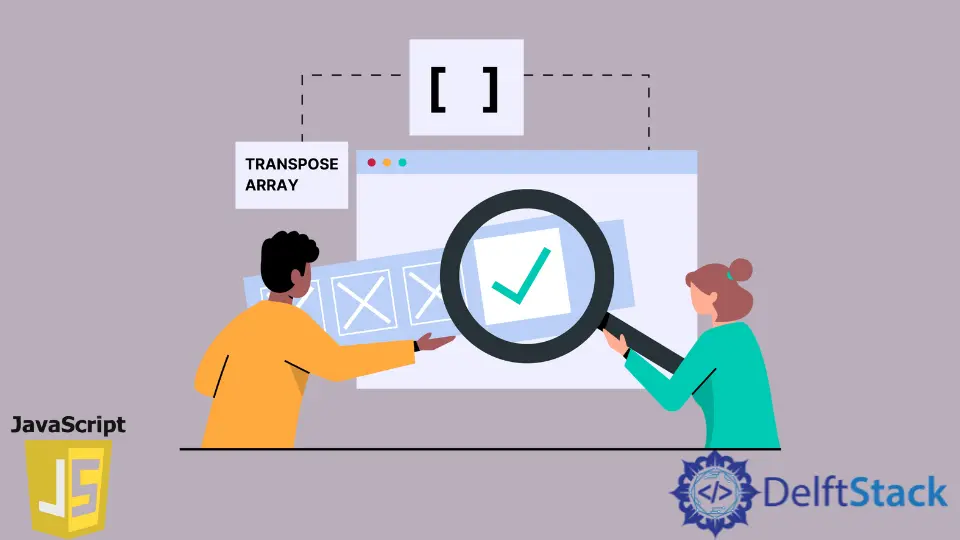
Like in many other programming languages, objects in JavaScript can be compared to objects in real life.
In JavaScript, an object is an independent entity with properties and a type. For example, a bike is an object with color, design, model number, make, etc.
Arrays are special types of objects whose keys are numerically predefined. Any JavaScript object can be converted to an array.
Arrays must use integers instead of strings as element indices. This article explains how to transpose an array in JavaScript.
What is Transposing of a Matrix
Transposing a matrix is one of the most common ways of transforming matrices into matrix concepts through linear algebra. The transpose of a matrix is acquired by changing the rows to columns and the columns to rows for a given matrix.
It is particularly useful in applications where inverses and adjoints of matrices are to be obtained.
The 2-dimensional array is a collection of elements that have a common name and are organized as an array of rows and columns. The 2-dimensional array is an array of arrays, so let’s create an array of one-dimensional array objects.
Use the map()
Method to Transpose an Array in JavaScript
The map()
method creates a new array due to calling a provided function for each element of the calling array. The map calls a provided callbackFn
function once for each element of an array in order and creates a new array from the results.
The callbackFn
is only called for array indexes that have assigned values (including undefined ones); it’s not called due to missing array elements, i.e., indices that have never been configured and indices that have been removed.
Syntax:
// Arrow function
map((element, index) => {/* Perform operation */})
// Callback function
map(callbackFn, thisArg)
// Inline callback function
map(function(element, index, array) { /* Perform operation */ }, thisArg)
The callbackFn
is a function called for each array element. Each time callbackFn
is executed, the return value is added to newArray
.
The callbackFn
function accepts the three arguments.
- An
element
is a required parameter representing the current element processed in the array. - An
index
is an optional parameter representing the current index of the element processed in the array. - An
array
is an optional parameter representing the called array map.
A thisArg
is an optional parameter representing the value when executing callbackFn
. This parameter returns a new array where each element of the array is the output of the callback function.
You can find more information about the map in the documentation for map()
.
Let’s take an example of OS Array; we will create a 2-dimensional array of OS and transpose them to the new array.
Example:
const osArray = [
['Linux', 'Windows', 'MacOS'],
['Linux', 'Windows', 'MacOS'],
['Linux', 'Windows', 'MacOS'],
];
const transposedOSArray =
osArray[0].map((_, colIndex) => osArray.map(row => row[colIndex]));
console.log(transposedOSArray);
We have defined a two-dimensional array; we use the map
function with element and column index. Each element is again iterated using a nested map
function and returned with the element of that particular index inside a row.
Running the code above in any browser prints something like this.
Output:
[['Linux', 'Linux', 'Linux'], ['Windows', 'Windows', 'Windows'], ['MacOS', 'MacOS', 'MacOS']]
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedInRelated Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript