How to Swap Array Elements in JavaScript
- Use Temporary Variable to Swap Array Elements in JavaScript
- Use ES6 Destructor Assignment to Swap Array Elements in JavaScript
- Use Bitwise XOR and Array Iteration to Swap Array Elements in JavaScript
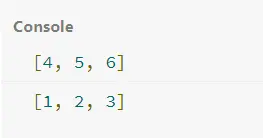
The traditional way of swapping two elements is to use a temp
variable. In the case of JavaScript, we can easily reassign the array objects to a variable set as an array object by default and make the process go further to transfer elements of two arrays for swap.
Again, the ES6 convention has brought a more efficient swapping for normal variables and objects. Another way is to use another traditional method of using a loop through the array elements and exchanging them, reading the indexes.
This way is too time-consuming and considers some extra lines of code.
Here, we will see all the preferable examples that will make the swap task more flexible. Let’s hop into the codebases!
Use Temporary Variable to Swap Array Elements in JavaScript
In the following example, we will take two arrays with corresponding elements. Our target here is to assign a new variable and transfer one of the arrays there.
Then reassign the other array to the array that has just shifted its content to the variable. And lastly, shift the variable contents to the last array we chose.
This is the basic operation for the temporary variable switching of array elements.
Code Snippet:
var x = [1, 2, 3];
var y = [4, 5, 6];
var a;
a = x;
x = y
y = a;
console.log(x)
console.log(y)
Output:
As you can see, the x
holds [1,2,3]
and y
holds [4,5,6]
. When the swapping is initiated, the variable a
takes the elements of x
, and the x
receives the elements of y
.
For the final step, y
appends the content of a
. The result infers a swapped outcome.
Use ES6 Destructor Assignment to Swap Array Elements in JavaScript
The ES6 destructor assignment swaps two arrays easier and requires only one line of code. It only needs to assign the arrays in square brackets and set the right side in a reverse form.
The pattern to set the destructor assignment is effortless if you look closely.
Code Snippet:
var x = [1, 3, 5];
var y = [2, 4, 6];
[x, y] = [y, x]
console.log(x)
console.log(y)
Output:
Use Bitwise XOR and Array Iteration to Swap Array Elements in JavaScript
Other than the two conventions mentioned above, there are multiple ways of swapping array elements. You can either swap using subtraction methods, bitwise XOR
operation, or the temporary variable rule by applying an iteration for the array.
Here, we have taken count of the XOR
operation to swap the elements of two arrays.
Code Snippet:
var x = [7, 42, 7];
var y = [4, 5, 6];
if (x.length == y.length) {
for (var i = 0; i < x.length; i++) {
x[i] = x[i] ^ y[i]
y[i] = x[i] ^ y[i]
x[i] = x[i] ^ y[i]
}
}
console.log(x)
console.log(y)
Output:
Let us consider the example, x[0] = 7
and y[0] = 4
. When we perform 7^4
, the corresponding bit pattern for 7
is 111
and 4
is 100
.
After the first XOR
, we get 011
, stored as x[0]
. For the next XOR
we have x[0] = 3 (011)
and y[0] = 100
, so the result, in this case, is stored in y[0]
is 111 = 7
.
And in x[0]
, we will get 111^011 = 100 (4)
. So, our first elements of the x
and y
array are swapped, and we can repeat this iteration for each indexed element.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript