How to Sum of an Array in JavaScript
-
Use the
for
Loop to Sum an Array in a JavaScript Array -
Use the
reduce()
Method to Sum an Array in a JavaScript Array -
Use the
lodash
Library to Sum an Array in a JavaScript Array
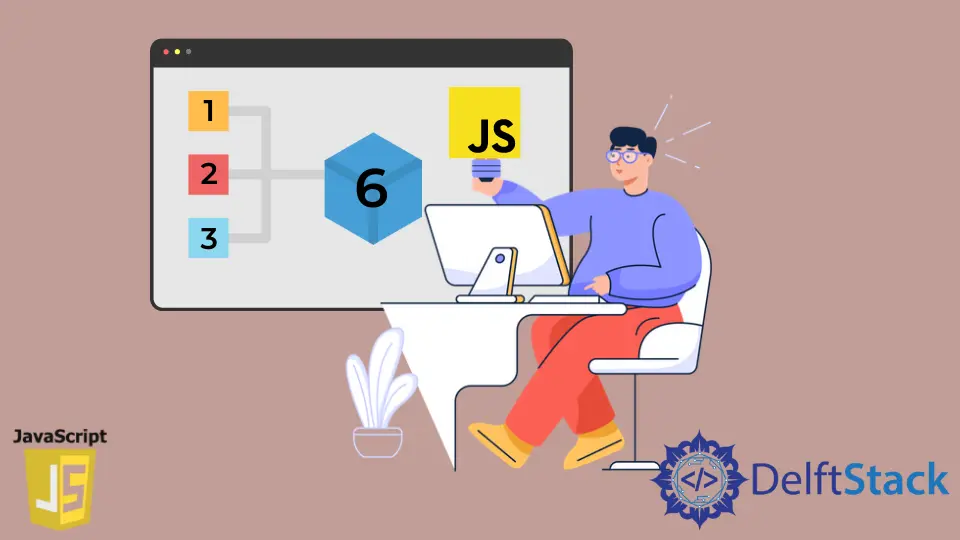
This tutorial teaches how to get the sum of an array of numbers in JavaScript.
Use the for
Loop to Sum an Array in a JavaScript Array
The for
loop is used to iterate an array. We can use it to add all the numbers in an array and store it in a variable.
const array = [1, 2, 3, 4];
let sum = 0;
for (let i = 0; i < array.length; i++) {
sum += array[i];
}
console.log(sum);
We initialize a variable sum
as 0
to store the result and use the for
loop to visit each element and add them to the sum of the array.
Use the reduce()
Method to Sum an Array in a JavaScript Array
The reduce()
method loops over the array and calls the reducer function to store the value of array computation by the function in an accumulator. An accumulator is a variable remembered throughout all iterations to store the accumulated results of looping through an array. We can use this to iterate through the array, add the element’s value to the accumulator and get the sum of the array.
const arr = [1, 2, 3, 4];
const reducer = (accumulator, curr) => accumulator + curr;
console.log(arr.reduce(reducer));
Use the lodash
Library to Sum an Array in a JavaScript Array
The lodash
library has a sum
method that can easily add the numbers present in an array.
var lodash = require('lodash');
var arr = [3, 6, 1, 5, 8];
var sum = lodash.sum(arr);
console.log(sum);
All the methods discussed above are compatible with all the major browsers.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedInRelated Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript