How to Get Subset of an Array in JavaScript
-
Extract the Subset of Array Elements From an Array Using
slice()
in JavaScript -
Extract the Subset of Array Elements From an Array With
splice()
in JavaScript
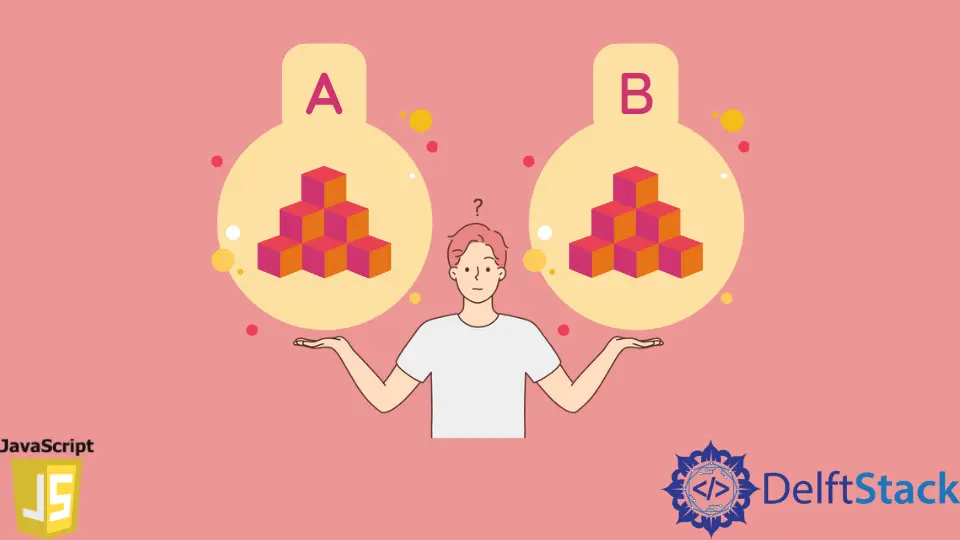
Arrays are an important part of a programming language because they contain many ordered elements.
All of these items are accessed through an index. In JavaScript, arrays are regular objects containing the value at the specified key, a numeric key.
Arrays are JavaScript objects with a fixed numeric key and dynamic values ​​containing any amount of data in a single variable.
An array can be one-dimensional or multi-dimensional. The JavaScript array can store anything like direct values or store JavaScript objects.
Unlike other languages, JS arrays can hold different data types at different indices of the same array. We will learn how to extract some of the elements or subsets of an array in JavaScript.
Extract the Subset of Array Elements From an Array Using slice()
in JavaScript
The slice()
method is a built-in method provided by JavaScript. This method cuts the array in two places.
This cut occurs by taking two inputs, the start index and the end index. And based on that, the part of the array between the indices will be returned.
If only the starting index is specified, it returns to the last element. The advantage of using slice
over splice
is that the original array is not mutated
as it is in a splice.
Syntax:
slice()
slice(start)
slice(start, end)
Each element present in the start
and end
indexes (including the starting element and stopping an element before the end) is inserted into the new array.
The end index is completely an optional parameter. See the slice()
method documentation for more information.
const inputArray = [1, 2, 3, 4, 5];
const outputArray1 = inputArray.slice(0, 1);
const outputArray2 = inputArray.slice(1, 3);
const outputArray3 = inputArray.slice(-2);
console.log(outputArray1);
console.log(outputArray2);
console.log(outputArray3);
When we call slice(0, 1)
the element 1
is copied from the initial array, inputArray
to outputArray1
.
If the starting index is larger than the length of an array, it will appear empty and also, the empty array will be returned as output.
Interestingly, the input parameter is considered an offset from the end of the sequence
if you specify a negative index. Once you run the above code in any browser, it’ll print something like this.
Output:
[1]
[2, 3]
[4, 5]
Extract the Subset of Array Elements From an Array With splice()
in JavaScript
The splice()
method affects or modifies the contents of an array. This is done by removing, replacing existing items, and adding new items in their place.
Syntax:
splice(start)
splice(start, deleteCount)
splice(start, deleteCount, item1)
splice(start, deleteCount, item1, item2, itemN)
The JavaScript splice method takes three input parameters, out of which the first one is start
.
The required parameter specifies an array’s starting position/index to modify the array. If greater than the length of an array, the start is set to the array’s length.
In this case, no elements are removed, but the method behaves like an addition function, adding as many elements as [n*] elements are provided.
If negative, start with that many elements at the end of the array.
The second parameter is deleteCount
, an optional parameter. This parameter specifies the number of elements of the array to get rid of from the start.
If deleteCount
is omitted or its value is equal to or greater than array.length - start
, all elements from the beginning to the end of the array are deleted.
0 or negative, no elements are deleted. In this case, you need to enter a minimum of one new item.
The third parameter is itemN...
, an optional parameter. This parameter specifies the elements to add to the array, starting at the beginning.
If you do not specify an item, splice()
only eliminates elements of the array.
This method returns a replacement array that contains the deleted items. An array of n elements is returned if only n elements are deleted.
If no element is deleted, an empty array is returned. See the splice
method documentation for more information.
const fruitsArray = ['Kiwi', 'Orange', 'Apple', 'Banana'];
console.log(fruitsArray, fruitsArray.splice(0, 2));
In the above example, we have specified the various styles of fruits. Once you extract the citrus fruit, it will affect the original fruitsArray
array and return an array.
That does not contain the name of the citrus fruit. Once you run the above code in any browser, it’ll print something like this.
Output:
["Apple", "Banana"], ["Kiwi", "Orange"]
To check the complete working code, click here.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedInRelated Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript