Static Variables in JavaScript
- Use the Property of a Function to Create Static Variables in JavaScript
- Use IIFE (Immediately Invoked Function Expression) to Create Static Variables in JavaScript
-
Use the
arguments.callee
to Create Static Variables in JavaScript - Use Constructors to Create Static Variables in JavaScript
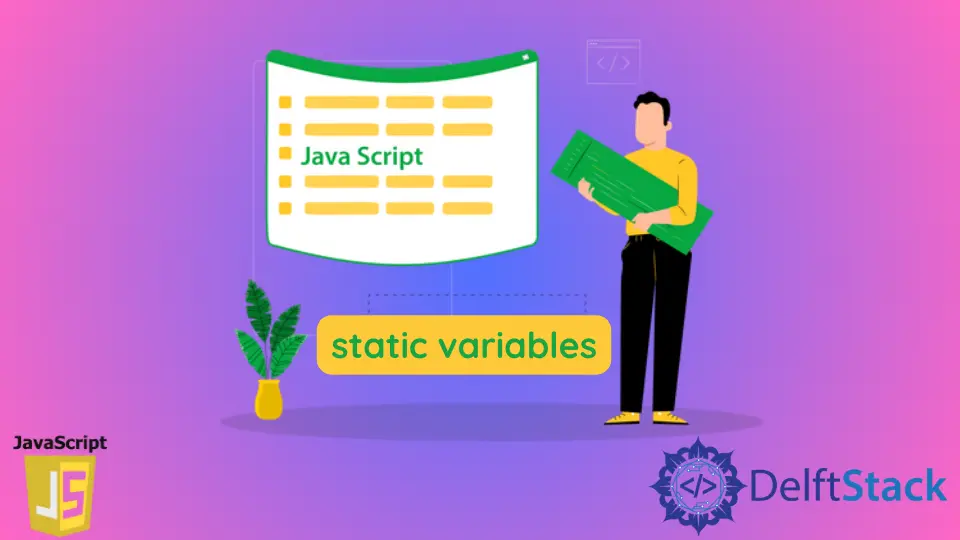
This tutorial introduces how to create static variables in JavaScript. Static variables are variables, typically used in functions, that maintain their value between function calls. The static
keyword helps to define a static property or method of a class.
Use the Property of a Function to Create Static Variables in JavaScript
Functions in JavaScript are objects and can have properties. So, we can create static variables by declaring the function’s properties. They maintain their values like global variables and can’t be modified outside the functions, making them much tidier than global variables.
function getId() {
if (typeof getId.counter == 'undefined') {
getId.counter = 0;
}
alert(++getId.counter);
}
Calling the above function multiple times increases the counter’s value and can’t be accessed outside function like global variables stopping all the mess.
Use IIFE (Immediately Invoked Function Expression) to Create Static Variables in JavaScript
IIFE are functions that are executed as soon as they are defined. It consists of two parts:
- An anonymous function with a lexical scope enclosed by the grouping operator
()
. - Function expression that is directly interpreted by JavaScript.
var incr = (function() {
var i = 1;
return function() {
return i++;
}
})();
incr(); // returns 1
incr(); // returns 2
Every time the function is called, the counter i
increases by one. i
is inaccessible outside because it is a function’s property, just like a typical static variable inside a class.
Use the arguments.callee
to Create Static Variables in JavaScript
We can use arguments.callee
to store static variables in JavaScript. It refers to the function being currently executed, and we can attach properties to it directly as we would to a function object.
function() {
arguments.callee.myStaticVar = arguments.callee.myStaticVar || 1;
arguments.callee.myStaticVar++;
alert(arguments.callee.myStaticVar);
}
This method is pretty similar to method 1, the only difference being instead of directly attaching properties, we are using arguments.callee
to add properties to the currently executing function.
Use Constructors to Create Static Variables in JavaScript
This method is the equivalent version of strongly-typed object-oriented languages like C++
/Java
/C#
. We try to assign variables to a whole type and not all instances.
function delftClass() {
var privateVariable = 'foo';
this.publicVariable = 'bar';
this.privilegedMethod = function() {
alert(privateVariable);
};
}
delftClass.prototype.publicMethod = function() {
alert(this.publicVariable);
};
delftClass.staticProperty = 'baz';
var myInstance = new delftClass();
Here we create the construction function delftClass
and then assign a static property that has nothing to do with the created instance. JavaScript treats functions as objects, so being an object, we can assign properties to a function. All the instances will share the static variable.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedInRelated Article - JavaScript Variable
- How to Access the Session Variable in JavaScript
- How to Check Undefined and Null Variable in JavaScript
- How to Mask Variable Value in JavaScript
- Why Global Variables Give Undefined Values in JavaScript
- How to Declare Multiple Variables in a Single Line in JavaScript
- How to Declare Multiple Variables in JavaScript