The setInterval Loop in JavaScript
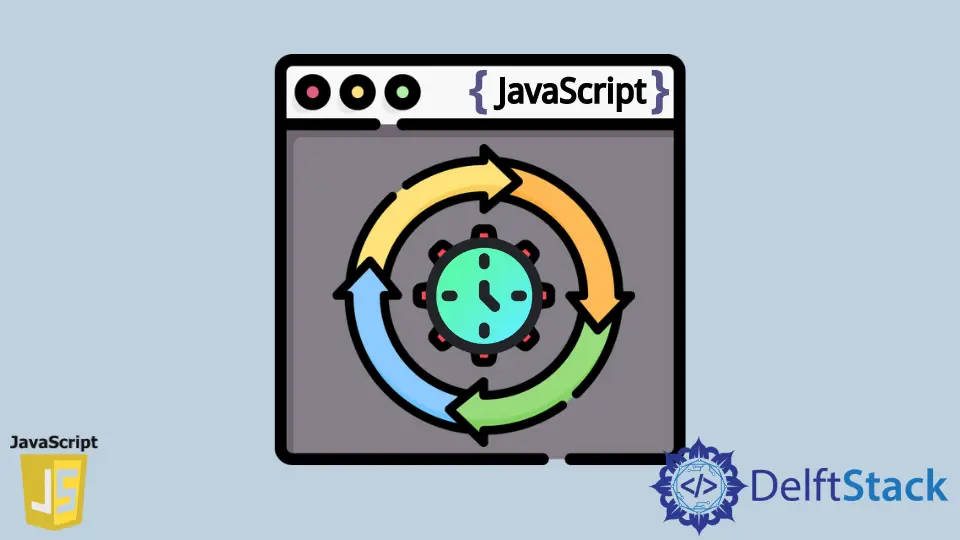
There is always a ready-made method available in JavaScript to accomplish most tasks; converting one data type to another, setting an interval loop, updating objects or arrays, recognizing keyboard input events, and much more.
In line with this, there are some useful methods to help us work with timers in JavaScript. And this article will guide you to use an interval loop in JavaScript.
JavaScript provides two timer functions to allow code to run without blocking. It provides the functions setTimeout()
and setInterval()
, which execute the specified expression or the specified function after a certain time interval.
Set Interval With Loop Time in JavaScript Using setInterval()
The method setInterval()
is provided by JavaScript. This method is offered in the worker
and window
interfaces, repeatedly calling a function or executing a code section. This code runs with a fixed time interval between each call.
The sole difference between the setInterval
and setTimeout
function is that prior calls the function repeatedly with a delay between each call whereas later executes a function after a delay. If you want your function to be called once after the specified delay, use setTimeout()
.
Syntax:
setInterval(function [, delay, arg1, arg2, ...]);
function
is a required parameter that specifies the function to be performed after the time has elapsed.
code
is a required parameter; if the user does not submit the function, the user can pass a string that is an alternative to the function. delay
is an optional parameter.
This parameter accepts the numeric value that serves as a timer in milliseconds before the specified code or function is executed. If no value is passed, 0
is the default value that causes execution.
arg1, ..., argN
is an optional parameter. The values of the function can be passed as an extra argument when the function is passed.
For more information, read the documentation of setInterval()
method.
let counter = 0;
const i = setInterval(function() {
console.log(counter);
counter++;
if (counter === 5) {
clearInterval(i);
}
}, 200);
In the code above, we set the counter to 0 and update it within the setInterval
function until it reaches the desired value. As soon as the desired value is reached, you can delete the interval. This setInterval()
method returns a positive value and a unique intervalID
that helps identify the timer. This value can be used for clearInterval()
. This clearInterval()
method cancels the repeated action (timeout) previously set by setInterval()
.
Output:
0
1
2
3
4
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn