How to Set Session Variable in JavaScript
-
localStorage
vssessionStorage
in JavaScript -
Set Variable in
localStorage
andsessionStorage
Using JavaScript
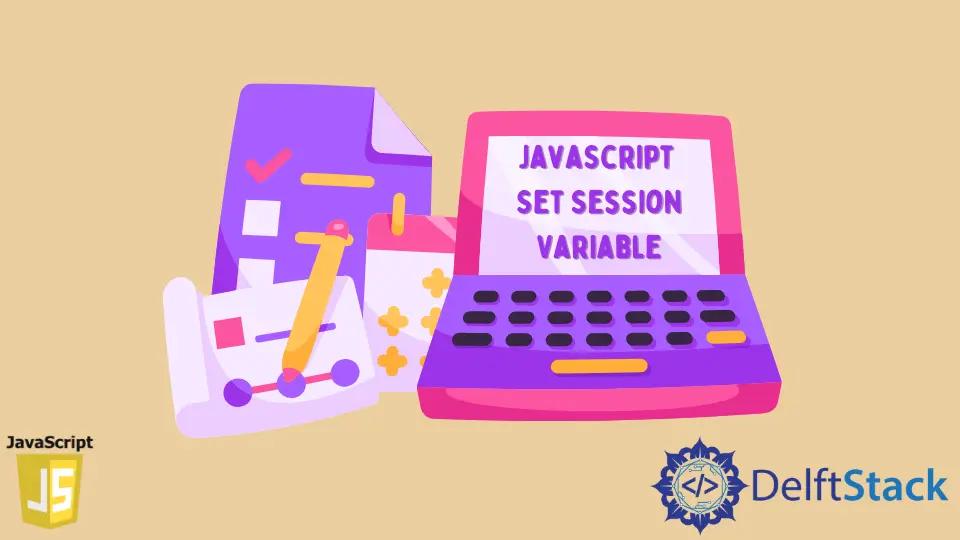
Our motive is to learn about sessions and set session variables in JavaScript. A session is a part of server-side storage where the data is stored and accessed depending on the unique id of each user.
For instance, whenever the user interacts with the website, it creates a new session object for that particular user and assigns them a cookie.
This cookie is checked whenever the user request from the exact client again and sent to the server for further processing and updating the session information that is already stored. The sessions get cleared as soon as the user clears the cookies.
You may have a question why is it important to use sessions?
The stateless HTTP protocol does not track any information about responses & requests, which means there is no relation among multiple requests by the one user (same user). The sessions are used to solve this issue.
For example, we have a form with multiple pages. Page 1, Page 2, and Page 3 are about personal information, education details, and family information, respectively.
The application can only be submitted once the user fills all three pages. In this scenario, we may need to store the submitted data from Page 1&2 into a session.
Once the user submits Page 3, we can get that page 3 from the HTTP request and the remaining Page 1&2 from the session. It is how sessions are used.
Now we understand sessions and know that the server maintains them. It is impossible to assign session values directly using JavaScript, but we (as web developers) want to use session storage locally to save, retrieve, update data.
We can use localStorage
and sessionStorage
.
localStorage
vs sessionStorage
in JavaScript
The localStorage
object allows storing data in key-value pairs in the browser with no expiration. Even after closing the tab or browser, the data can be used for upcoming sessions (future sessions).
The sessionStorage
object is similar to localStorage
, but it stores data only for one session. The data is lost when the tab/browser is closed or refreshed.
Following is a brief comparison among both of these, but you can find more about it here.
Local Storage | Session Storage | |
---|---|---|
Capacity | 10 MB | 5 MB |
Browsers | HTML 5 | HTML 5 |
Accessible From | Any Window | Same Tab |
Expires | Never | On Tab Close |
Storage Location | Browser Only | Browser Only |
Sent With Requests | No | No |
Set Variable in localStorage
and sessionStorage
Using JavaScript
The methods are the same for storing and retrieving data in localStorage
and sessionStorage
. These methods are listed below.
setItem(key, value)
stores key-value pairs.getItem(key)
retrieves the value via key.removeItem(key)
removes both the key and value.clear()
deletes the entire storage and clears everything.key(index)
returns the key on a particular position.length
tells the number of stored items.
Never use these storage mechanisms to store confidential information.
JavaScript Code to Practice For localStorage
:
localStorage.setItem('fname', 'Bob');
localStorage.setItem('lname', 'Thomas');
console.log(localStorage.getItem('fname'));
console.log(localStorage.getItem('lname'));
// returns null
console.log(localStorage.getItem('firstname'));
// removes the item with key and value
localStorage.removeItem('fname');
// clears the entire storage
localStorage.clear()
Output:
JavaScript Code to Practice For sessionStorage
:
sessionStorage.setItem('fname', 'Bob');
sessionStorage.setItem('lname', 'Thomas');
console.log(sessionStorage.getItem('fname'));
console.log(sessionStorage.getItem('lname'));
// returns null
console.log(sessionStorage.getItem('firstname'));
// removes the item with key and value
sessionStorage.removeItem('fname');
// clears the entire storage
sessionStorage.clear()
Output:
Related Article - JavaScript Variable
- How to Access the Session Variable in JavaScript
- How to Check Undefined and Null Variable in JavaScript
- How to Mask Variable Value in JavaScript
- Why Global Variables Give Undefined Values in JavaScript
- How to Declare Multiple Variables in a Single Line in JavaScript
- How to Declare Multiple Variables in JavaScript