How to Scroll to Top of Div in JavaScript
-
Use the
scrollIntoView()
Method to Scroll to the Top of adiv
in JavaScript -
Use the
scrollTo()
Method to Scroll to the Top of adiv
in JavaScript -
Use the
scrollTop
Property to Scroll to the Top of adiv
in JavaScript - Conclusion
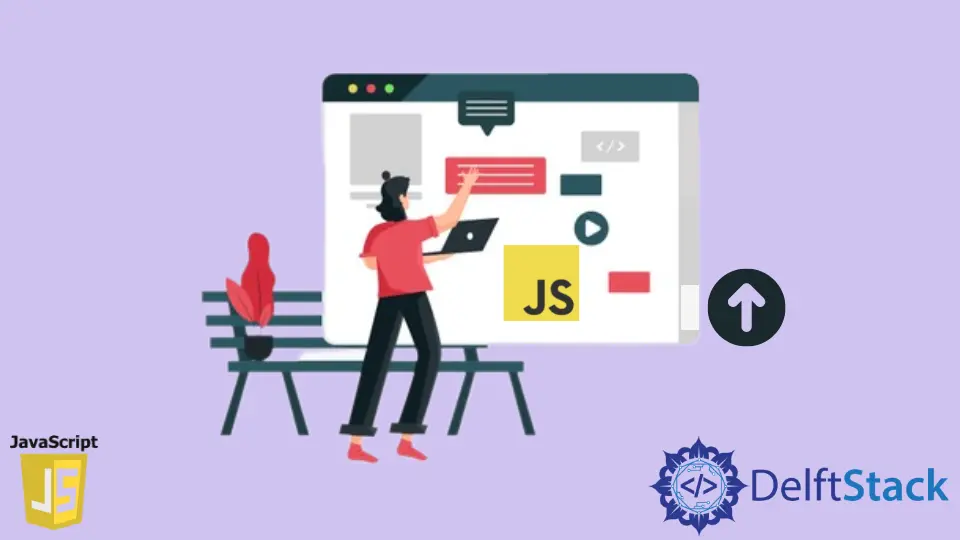
Scrolling to the top of a specific div
in a web page is a common requirement in web development. JavaScript provides several methods to achieve this functionality, including the scrollIntoView()
method, the scrollTo()
method, and the scrollTop
property.
In this article, we’ll explore how to utilize these methods to smoothly scroll to the top of a div
element.
Use the scrollIntoView()
Method to Scroll to the Top of a div
in JavaScript
The scrollIntoView()
method is available for DOM elements in modern web browsers. It’s used to scroll the container element (e.g., div
, section
, body
) so that the current element becomes visible within the viewport.
This method accepts an optional options
parameter, allowing you to customize the scrolling behavior. However, for our purpose of scrolling to the top of a div
, we won’t delve into the optional parameters.
To scroll to the top of a div
using scrollIntoView()
, you first need to obtain a reference to the div
element you want to scroll to the top.
This can be done using document.getElementById()
, document.querySelector()
, or similar methods.
var myDiv =
document.getElementById('myDiv'); // Replace 'myDiv' with your div's ID
Then, call the scrollIntoView()
method on the div
element to scroll it into view.
myDiv.scrollIntoView({behavior: 'smooth'}, true);
By calling scrollIntoView()
on the div
element, the browser will smoothly scroll the page to ensure the top of the div
is visible within its parent container.
Let’s put the above steps together in a practical example:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>JS Bin</title>
<style>
#top{
height:200px;
background:pink;
}
#buff{
height:800px;
}
</style>
</head>
<body>
<div id="top"></div>
<div id="buff"></div>
<a href="javascript: scroll()">Jump to top of page</a>
<script>
function scroll(){
var top = document.getElementById("top").scrollIntoView({behavior: 'smooth'}, true);
}
</script>
</body>
</html>
Output:
Here, the HTML includes two div
elements with IDs top
and buff
, each styled using CSS for height and background. Additionally, an anchor (<a>
) element acts as a link to a JavaScript function named scroll()
.
When the link is clicked, the scroll()
function is invoked, utilizing the scrollIntoView()
method on the top
element. This method, configured with {behavior: 'smooth'}
for smooth scrolling, effectively scrolls the page to align the top of the top
element with the top of the viewport.
The JavaScript function combines with the HTML structure to achieve the desired scrolling behavior, enhancing the user experience by smoothly navigating to the specified portion of the webpage.
Use the scrollTo()
Method to Scroll to the Top of a div
in JavaScript
We can also use the scrollTo()
method in JavaScript to control the scroll position of a webpage. It allows us to specify the x and y coordinates to which the viewport should be scrolled.
When using the scrollTo()
method to scroll to the top of a div
, we essentially want to reset the current viewport position to have the div
at the top of the visible area. The scrollTo()
method takes the x and y coordinates as parameters, representing the new position for the viewport.
To scroll to the top of a div
, we’ll set both the x and y coordinates to 0
. This will bring the top of the div
to the top of the viewport, ensuring it is visible to the user.
Here’s a simple example demonstrating how to use the scrollTo()
method to scroll to the top of a div
when a link is clicked:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>JS Bin</title>
<style>
#top{
height:200px;
background:orange;
}
#buff{
height:800px;
}
</style>
</head>
<body>
<div id="top"></div>
<div id="buff"></div>
<a href="javascript: scroll()">Jump to top of page</a>
<script>
function scroll(){
var top = document.getElementById("top")
window.scrollTo(0,0);
}
</script>
</body>
</html>
Output:
As we can see, in the example code, we defined two div
elements within the HTML, each styled with specific heights and backgrounds.
The crucial functionality is triggered by a link labeled Jump to top of page
. This link calls a JavaScript function named scroll()
upon being clicked.
Inside this function, the scrollTo()
method is employed with parameters (0, 0)
to reset the viewport’s position, effectively scrolling to the top of the div
with the ID top
.
This seamlessly ensures that the top of the designated div
becomes the topmost visible area on the screen, enhancing user experience and navigation within the webpage.
Use the scrollTop
Property to Scroll to the Top of a div
in JavaScript
The scrollTop
property is used to get or set the number of pixels that an element’s content is scrolled vertically. By setting this property to 0, we can scroll to the top of the element.
Here’s how you can scroll back to the top of a scrollable div
using the scrollTop
property in JavaScript:
First, you need to access the scrollable div
in your HTML using JavaScript. You can achieve this by using the getElementById
method or any other suitable method to select the desired element.
const scrollableDiv = document.getElementById('yourScrollableDivId');
Replace 'yourScrollableDivId'
with the actual ID of your scrollable div
.
Next, set the scrollTop
property of the scrollable div
to 0. This will scroll the content of the div
to the top.
scrollableDiv.scrollTop = 0;
Take a look at the example below:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Scroll to Top Example</title>
<style>
#top {
height: 200px;
background: red;
}
#buff {
height: 800px;
overflow-y: scroll;
}
</style>
</head>
<body>
<div id="top"></div>
<div id="buff"></div>
<a href="#" onclick="scrollToTop()">Jump to top of page</a>
<script>
function scrollToTop() {
const scrollableDiv = document.getElementById("buff");
scrollableDiv.scrollTop = 0;
}
</script>
</body>
</html>
Output:
This code demonstrates a straightforward example of implementing a “scroll to top” functionality on a webpage using HTML, CSS, and JavaScript.
In the HTML body, two div
elements are defined: one representing the top of the page and another acting as a buffer with a designated height, enabling vertical scrolling. A link is also created, prompting the user to Jump to top of page
.
The critical functionality is driven by a JavaScript function, scrollToTop()
, linked to the link element. When this link is clicked, the JavaScript function is executed, utilizing the element with the ID buff
to scroll to the top of the page by setting the scrollTop
property to 0.
Essentially, clicking the link triggers the scrolling action, providing a convenient way for users to navigate back to the top of the webpage.
Conclusion
Scrolling to the top of a specific div
in JavaScript is achievable through various methods like scrollIntoView()
, scrollTo()
, and the property scrollTop
. Depending on your requirements and preferences, you can choose the most suitable method to provide a smooth scrolling experience to users.
Implement these techniques in your web development projects to enhance user interaction and navigation within your web pages.
Related Article - JavaScript Div
- How to Show/Hide Div onClick in JavaScript
- How to Reload DIV in JavaScript
- How to Scroll to Bottom of a Div in JavaScript
- How to Add HTML to Div Using JavaScript