How to Add HTML to Div Using JavaScript
-
Use the
createElement()
Method to Adddiv
in HTML -
Use the
insertAdjacentHTML()
Method to Adddiv
Element
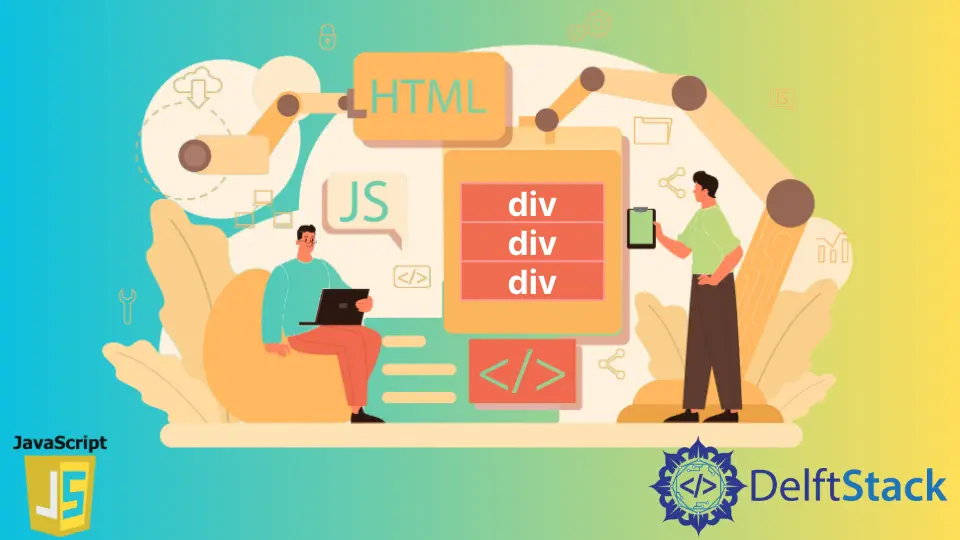
To create a div element from JavaScript, you must manipulate the DOM. The most commonly used and preferred way depends on the createElement()
method to create a div element.
We can also declare the necessary other elements inside the created div element. A less practiced method is the insertAdjacentHTML()
, which is often not supported by Firefox and Safari.
In the following examples, we will see the use of both methods. The instances will create a div
element under the main
element having a specific id
.
Use the createElement()
Method to Add div
in HTML
Here, we will first create a button
element to trigger a function that will create a new element. We will also set the main
element and an id=content
for our new div elements to be added to this segment.
In our JavaScript lines, we will create an instance for the div element that will initiate the createElement('div')
to set a value to the instance. In the innerHTML
, we will also define a command that will ensure us that our div
is created on function call.
Code Snippet - HTML:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>test</title>
</head>
<body>
<button onclick="add()">Make div</button>
<main id="content"></main>
</body>
</html>
Code Snippet - JavaScript:
function add() {
const div = document.createElement('div');
div.innerHTML = 'new div';
document.getElementById('content').appendChild(div);
}
Output:
With each click on the button, a new div
element with the content new div
is created. To be closely noticed is that the div
element maintains the hierarchy of parent and child elements.
As the new div
element is a child element of the main
element, thus the appenChild()
method is functioning.
Use the insertAdjacentHTML()
Method to Add div
Element
The insertAdjacentHTML()
refers to a specific position and the right format for adding the child div
element. In this case, we have this afterbegin
parameter that creates a div
right after the start, aka as a first child.
This loop will continue until it meets any condition to break.
Code Snippet - HTML:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>test</title>
</head>
<body>
<button onclick="add()">Make div</button>
<main id="content"></main>
</body>
</html>
Code Snippet - JavaScript:
function add() {
document.querySelector('#content')
.insertAdjacentHTML('afterbegin', `<div class="row">
<input type="text" name="name" value="Generated div" />
</div>`)
}
Output:
As it can be seen, right after the afterbegin
parameter, we have created the div
element explicitly. Each button press creates a new div
element relative to the previous div
element.
This method is similar and defined to be executed properly, but some major browsers do not have modifications to deal with it.