How to Scroll to Bottom of a Div in JavaScript
-
Use
scrollTop
andscrollHeight
to Scroll to Bottom of a<div>
in JavaScript -
Use jQuery to Scroll to Bottom of a
<div>
in JavaScript -
Use jQuery
.animate()
to Scroll to Bottom of a<div>
in JavaScript -
Use
element.scroll()
to Scroll to Bottom of a<div>
in JavaScript -
Use
element.scrollIntoView()
to Scroll to Bottom of a<div>
in JavaScript - Conclusion
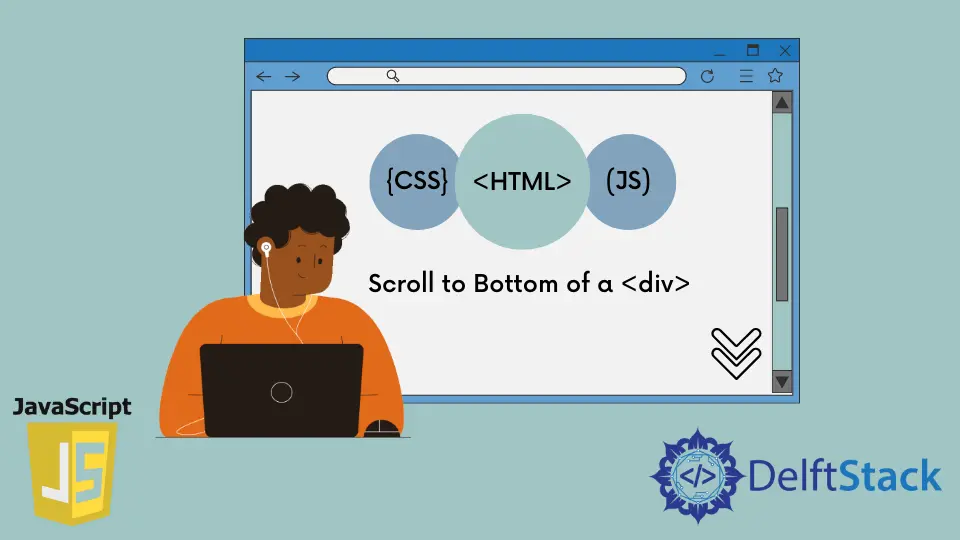
Scrolling to the bottom of a <div>
element using JavaScript is a crucial functionality in web development. This action is often required when dealing with dynamic content or chat applications, where new messages are added, and the user needs to be automatically scrolled to the latest message.
In this process, JavaScript provides several methods and techniques to accomplish this task efficiently. These methods include manipulating properties like scrollTop
, utilizing the scrollIntoView()
function, leveraging jQuery for animation, and employing smooth scroll behavior available in modern browsers.
In this article, we will explore each of these methods in detail, providing example code and explanations for each. By the end of this guide, you will have a comprehensive understanding of how to implement scrolling to the bottom of a <div>
element in your web applications.
Use scrollTop
and scrollHeight
to Scroll to Bottom of a <div>
in JavaScript
The scrollTop
property of an element represents the number of pixels by which the content of the element is scrolled vertically. When set, it determines the vertical scroll position.
Syntax:
element.scrollTop
element
: The DOM element you want to get or set the vertical scroll position of.
The scrollHeight
property returns the entire height of an element’s content, including any content that is not currently visible due to scrolling. It is a read-only property.
Syntax:
element.scrollHeight
element
: The DOM element for which you want to get the total height of the content (including any content not currently visible due to scrolling).
A combination of scrollTop
and scrollHeight
can cause an element to scroll to the bottom because scrollTop
determines the number of pixels for a vertical scroll. In contrast, scrollHeight
is the element’s height (visible and non-visible parts).
Therefore, for an element, when scrollTop
equals scrollHeight
, the browser scrolls up. This allows us to see the bottom of the element.
However, this method requires that the element should be scrollable. You can achieve this when the element has a child element that causes a vertical overflow.
Meanwhile, you can control the overflow with overflow-y: scroll
. This means that without overflow-y: scroll
, this method will not work.
For example, in the next code block, the HTML element with an ID of scroll-to-bottom
has a height of 300
pixels. Meanwhile, the child element has a height of 400
pixels.
As a result, the child element causes an overflow in its parent element. However, we’ve controlled the overflow with overflow-y: scroll
in the CSS.
<head>
<meta charset="utf-8">
<title>Scroll to bottom - 1 (scrollTop and scrollHeight)</title>
<style type="text/css">
#scroll-to-bottom {
border: 5px solid #1a1a1a;
padding: 2em;
width: 50%;
margin: 0 auto;
height: 300px;
overflow-y: scroll;
}
.content {
height: 400px;
}
p {
font-size: 100px;
}
</style>
</head>
<body>
<div id="scroll-to-bottom">
<div class="content">
<p>This is a dummy text</p>
</div>
</div>
<script>
let scroll_to_bottom = document.getElementById('scroll-to-bottom');
scroll_to_bottom.scrollTop = scroll_to_bottom.scrollHeight;
</script>
</body>
Output:
This code is an HTML document that includes a <div>
element with the id scroll-to-bottom
. This <div>
has a child element with the class content
, which contains a <p>
element with the text "This is a dummy text"
. The CSS styles define the appearance and layout of these elements.
Here’s a breakdown of the code:
- HTML Structure:
- There is a
<div>
element with the idscroll-to-bottom
. This is the container element that we want to scroll. - Inside the
scroll-to-bottom
<div>
, there is another<div>
element with the classcontent
. This is the content that we want to scroll within the container. - Inside the
content
<div>
, there is a<p>
element with the text"This is a dummy text"
.
- There is a
- CSS Styles:
- The CSS styles define the appearance of the elements. Notably, the
#scroll-to-bottom
element has a fixed height of300
pixels andoverflow-y
set toscroll
, which means it will have a vertical scrollbar if the content overflows.
- The CSS styles define the appearance of the elements. Notably, the
- Script:
- The script at the end of the HTML document uses JavaScript to scroll the
#scroll-to-bottom
element to its bottom. It does this by setting thescrollTop
property of the element to itsscrollHeight
. This ensures that the content is scrolled all the way down.
- The script at the end of the HTML document uses JavaScript to scroll the
Use jQuery to Scroll to Bottom of a <div>
in JavaScript
jQuery is a fast, small, and feature-rich JavaScript library. It simplifies many common tasks like DOM manipulation, event handling, and AJAX calls. One of its strengths is its ability to achieve complex tasks with concise code.
jQuery provides the scrollTop()
method to get or set the vertical scrollbar position for an element. The scroll bar in question will be for a set of matched elements.
When used in conjunction with scrollHeight
, it becomes a powerful tool for scrolling to the bottom of a <div>
.
scrollTop()
Method Syntax (in jQuery):
$(selector).scrollTop(value)
selector
: A jQuery selector that identifies the element you want to manipulate the scroll position of.value
(optional): An optional parameter representing the vertical scroll position in pixels that you want to set for the element.
Example Usage:
$('#myDiv').scrollTop(100); // Set the scroll position to 100 pixels
scrollHeight
Property Syntax (in jQuery):
$(selector)[0].scrollHeight
selector
: A jQuery selector that identifies the element you want to get the total height of the content (including any content not currently visible due to scrolling).
Example Usage:
let totalHeight = $('#myDiv')[0].scrollHeight; // Get the total height of the content
In the example below, we’ve selected an element with the jQuery selector. At the same time, we’ve used the element scrollTop
to select the element and its scrollHeight
.
You’ll note from the code that we’ve used [0]
to get the DOM element. As a result, when you load the code in your web browser, the <div>
will scroll to the bottom.
What’s more, in the JavaScript code, we’ve provided variants of this approach. However, we’ve left them as comments.
As a final note, this approach also requires that the element be scrollable.
<head>
<meta charset="utf-8">
<title>Scroll to bottom - 2 (jQuery)</title>
<style type="text/css">
#scroll-to-bottom {
border: 5px solid #1a1a1a;
padding: 2em;
width: 50%;
margin: 0 auto;
height: 300px;
overflow-y: scroll;
}
.content {
height: 400px;
}
p {
font-size: 100px;
}
</style>
</head>
<body>
<div id="scroll-to-bottom">
<div class="content">
<p>This is a dummy text</p>
</div>
</div>
<script
src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"
integrity="sha512-894YE6QWD5I59HgZOGReFYm4dnWc1Qt5NtvYSaNcOP+u1T9qYdvdihz0PPSiiqn/+/3e7Jo4EaG7TubfWGUrMQ=="
crossorigin="anonymous"
referrerpolicy="no-referrer"
>
</script>
<script>
$('#scroll-to-bottom').scrollTop($('#scroll-to-bottom')[0].scrollHeight);
// The following code does the same thing:
// $('#scroll-to-bottom').scrollTop(function() {
// return this.scrollHeight;
// });
// let scroll_to_bottom = $("#scroll-to-bottom");
// scroll_to_bottom.scrollTop(scroll_to_bottom.prop("scrollHeight"));
</script>
</body>
Output:
The above HTML document demonstrates a scenario where a <div>
element with the id scroll-to-bottom
is styled to have a fixed height with a scrollable content area. The jQuery library is included to facilitate dynamic scrolling.
Here’s a detailed breakdown of the code:
- HTML Structure:
- There is a
<div>
element with the idscroll-to-bottom
. This is the container element that we want to scroll. - Inside the
scroll-to-bottom
<div>
, there is another<div>
element with the classcontent
. This is the content that we want to scroll within the container. - Inside the
content
<div>
, there is a<p>
element with the text"This is a dummy text"
.
- There is a
- CSS Styles:
- The CSS styles define the appearance of the elements. Notably, the
#scroll-to-bottom
element has a fixed height of300
pixels andoverflow-y
set toscroll
, which means it will have a vertical scrollbar if the content overflows.
- The CSS styles define the appearance of the elements. Notably, the
- JavaScript (jQuery):
- The jQuery library is included from a CDN using a
<script>
tag. - The script following the jQuery inclusion is using jQuery to scroll the
#scroll-to-bottom
element to its bottom. It does this by setting thescrollTop
property of the element to itsscrollHeight
. This ensures that the content is scrolled all the way down.
- The jQuery library is included from a CDN using a
Overall, this code demonstrates how jQuery can be used to dynamically scroll to the bottom of a <div>
element, ensuring that the latest content is visible to the user.
Use jQuery .animate()
to Scroll to Bottom of a <div>
in JavaScript
jQuery’s .animate()
function is a powerful tool for creating animations and transitions in web applications.
It allows you to smoothly change CSS properties over a specified duration, creating visually engaging effects. Therefore, you can create a smooth scroll effect.
What we mean by “smooth” is that the scroll-to-bottom effect will appeal to the users. However, you’ll need scrollTop
and scrollHeight
to pull this off.
The syntax of jQuery’s .animate()
function is as follows:
$(selector).animate(styles, options);
selector
: A jQuery selector that identifies the element(s) to be animated.styles
: An object or a set of CSS properties and values that you want to animate.options
(optional): An object containing additional settings for the animation, such as duration, easing, and callback functions.
In the example below, we aim to keep the code clean because we use the .animate()
API. So, we’ll wrap all our code in a function.
The inner working of the function is as follows:
- Get the element’s ID using the jQuery selector.
- Attach the
.animate()
API to this element. - Pass an object as the first argument of the
.animate()
API. In this object, do the following:- Create an object property whose name is
scrollTop
. - Set
scrollTop
value toelement.prop("scrollHeight")
.
- Create an object property whose name is
- Set the second argument of the
.animate()
API as the animation duration.
Afterward, we call this function on the element’s HTML ID. Meanwhile, the element should be scrollable with CSS overflow-y: scroll
.
<head>
<meta charset="utf-8">
<title>Scroll to bottom - 3 (jQuery smooth)</title>
<style type="text/css">
#scroll-to-bottom {
border: 5px solid #1a1a1a;
padding: 2em;
width: 50%;
margin: 0 auto;
height: 300px;
overflow-y: scroll;
}
.content {
height: 400px;
}
p {
font-size: 100px;
}
</style>
</head>
<body>
<div id="scroll-to-bottom">
<div class="content">
<p>This is a dummy text</p>
</div>
</div>
<script
src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"
integrity="sha512-894YE6QWD5I59HgZOGReFYm4dnWc1Qt5NtvYSaNcOP+u1T9qYdvdihz0PPSiiqn/+/3e7Jo4EaG7TubfWGUrMQ=="
crossorigin="anonymous"
referrerpolicy="no-referrer"
>
</script>
<script>
const smoothScroll = (id) => {
const element = $(`#${id}`);
element.stop().animate({
scrollTop: element.prop("scrollHeight")
}, 500);
}
smoothScroll('scroll-to-bottom');
</script>
</body>
Output:
The above code sets up a scenario where the content inside the #scroll-to-bottom
<div>
will be smoothly scrolled to the bottom when the page loads. The smoothScroll
function provides a reusable way to implement this scrolling behavior for other elements by passing their respective IDs as arguments.
Use element.scroll()
to Scroll to Bottom of a <div>
in JavaScript
The element.scroll()
method is a native JavaScript method that allows you to scroll an element to a specified position. It can be used to scroll both horizontally and vertically.
In the context of scrolling to the bottom of a <div>
, we will focus on vertical scrolling.
The method takes two arguments: left
and top
. We will set left
to 0
and top
to a value representing the desired vertical position.
Syntax:
element.scroll(options);
element
: The DOM element you want to scroll.options
(optional): An object containing the scroll options, which can include thetop
andleft
properties to specify the scroll positions.
You can use element.scroll()
to scroll to the bottom of an element. What element.scroll()
requires is a set of coordinates.
We’ll set the coordinates to the top of the element in this case. Also, if you’d like, you can make it a smooth scrolling.
To scroll to the bottom with element.scroll()
, set its first argument to an object. Afterward, the first property of this object should be a top
property.
This property should have a value of element.scrollHeight
. Also, set the second argument of element.scroll()
to behavior: "smooth"
for a smooth scroll.
We’ve used element.scroll()
on the element in the following code. As a result, when you run the code in your browser, the <div>
will scroll to the bottom.
In addition, the element should be scrollable via CSS overflow-y: scroll
.
<head>
<meta charset="utf-8">
<title>Scroll to bottom - 4 (Element.scroll())</title>
<style type="text/css">
#scroll-to-bottom {
border: 5px solid #1a1a1a;
padding: 2em;
width: 50%;
margin: 0 auto;
height: 300px;
overflow-y: scroll;
}
.content {
height: 400px;
}
p {
font-size: 100px;
}
</style>
</head>
<body>
<div id="scroll-to-bottom">
<div class="content">
<p>This is a dummy text</p>
</div>
</div>
<script>
let scroll_to_bottom = document.getElementById('scroll-to-bottom');
function scrollBottom(element) {
element.scroll({ top: element.scrollHeight, behavior: "smooth"})
}
scrollBottom(scroll_to_bottom);
</script>
</body>
Output:
The above HTML document sets up a scrollable <div>
with the ID scroll-to-bottom
containing a large text.
The JavaScript code defines a function scrollBottom(element)
which smoothly scrolls the specified element to its bottom using the element.scroll()
method with a "smooth"
animation behavior. When the page loads, this function is called, ensuring that the content is fully visible.
Use element.scrollIntoView()
to Scroll to Bottom of a <div>
in JavaScript
The element.scrollIntoView()
method is a native JavaScript method that scrolls the element into view, aligning it either at the top or bottom of the viewport.
In the context of scrolling to the bottom of a <div>
, we will use the parameter false
to align the element at the bottom of the viewport.
Syntax:
element.scrollIntoView([options]);
element
: The DOM element you want to scroll into view.options
(optional): An object containing options for customizing the scrolling behavior.
The element.scrollIntoView()
method will scroll an element to be visible to the user. As a result, you see the overflow content hidden from sight. At the same time, the bottom of the element.
Unlike previous examples in this article, you’ll need a single <div>
element. This <div>
element should have some content.
Also, you don’t need to set a height for the <div>
because, with scrollIntoView
, a defined height could prevent a scroll to the bottom.
In the following code, we’ve called scrollIntoView()
on the <div>
element. Also, we’ve set its argument to false
. This is what is required to scroll the element to the bottom.
<head>
<meta charset="utf-8">
<title>Scroll to bottom - 5 (scrollIntoView())</title>
<style type="text/css">
body {
padding: 1em;
}
div {
border: 5px solid #1a1a1a;
padding: 2em;
width: 50%;
margin: 0 auto;
}
p {
font-size: 100px;
}
</style>
</head>
<body>
<div id="scroll-to-bottom">
<p>This is a dummy text</p>
</div>
<script type="text/javascript">
let scroll_to_bottom = document.getElementById('scroll-to-bottom');
scroll_to_bottom.scrollIntoView(false);
// scroll_to_bottom.scrollIntoView({ behavior: 'smooth', block: 'end'});
</script>
</body>
Output:
The above HTML document creates a simple webpage with a centered <div>
element containing a large text paragraph. The <div>
has a border and padding for styling.
In the JavaScript section, the scroll_to_bottom
variable is assigned the reference to the <div>
element with the ID scroll-to-bottom
.
The line scroll_to_bottom.scrollIntoView(false);
utilizes the scrollIntoView()
method to immediately scroll the element into view, aligning it at the bottom of the viewport. This means the content within the <div>
becomes immediately visible without the need for manual scrolling.
Conclusion
This article explores various methods to scroll to the bottom of a <div>
element using JavaScript. It covers five distinct techniques: scrollTop
and scrollHeight
, jQuery’s scrollTop()
, jQuery’s .animate()
, element.scroll()
, and element.scrollIntoView()
.
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedInRelated Article - JavaScript Div
- How to Show/Hide Div onClick in JavaScript
- How to Reload DIV in JavaScript
- How to Scroll to Top of Div in JavaScript
- How to Add HTML to Div Using JavaScript