How to Scroll to the Top of a Page in JavaScript
-
Scroll to the Top of a Page Using Vanilla JS
window.scrollTo()
Method in JavaScript -
Scroll to the Top of a Page Using an Animated Vanilla JS - New Form of the
window.scrollTo()
Method -
Scroll to the Top of a Page Using the jQuery
scrollTop()
Method -
Scroll to the Top of a Page by Animating With the jQuery
animate()
Method
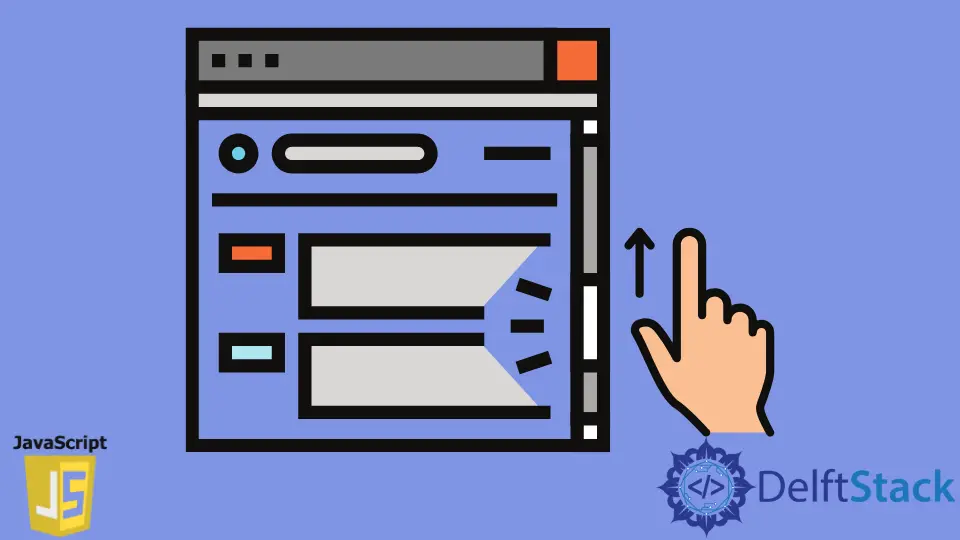
It is crucial to have a UI element for long web pages to let users scroll to the top of the page JavaScript.
In this tutorial, you will find several JavaScript methods to scroll to the top.
We will use the vanilla JavaScript scrollTo
method. We will also show how to use the jQuery scrollTop()
method and scrollTop
property to scroll to the top (read ahead to find out the benefit of using jQuery).
We will also give bonus tricks: a clever one-line pure HTML shortcut and a custom vanilla JS animation to scroll to the top of a page.
Scroll to the Top of a Page Using Vanilla JS window.scrollTo()
Method in JavaScript
You can use the vanilla JS window.scrollTo()
method to scroll to the top of the page in JavaScript. Using the window.scrollTo()
method, we can scroll to any specified set of x- and y-coordinates in the document.
The old form of this method works like the following:
window.scrollTo(x - coordinate, y - coordinate);
We can use it to scroll to the top of a page in JavaScript by passing in (0,0)
as the arguments for the coordinates.
let scrollTopBtn = document.getElementById('top');
scrollTopBtn.addEventListener('click', function() {
window.scrollTo(0, 0);
});
(Please see the attached HTML and CSS files to follow along easily with the code.)
You can see the demo of this code below:
Scroll to the Top of a Page Using an Animated Vanilla JS - New Form of the window.scrollTo()
Method
The above solutions get the job done, but it does not make for good UX.
The window.scrollTo()
method also has a new form that lets us animate the scrolling motion. You can improve the UX and user engagement metrics with this nice little animated effect.
The new form works like the following:
window.scrollTo(options)
// options is a JSON objectwith
// top: the y-coordinate to scroll to
// left : the x-coordinate to scroll to
// behavior : an animated effect with vrious string values like 'smooth',
// 'slow' etc.
We can animate scrolling to the top of the page with vanilla JavaScript using this form of this method.
let scrollTopBtn = document.getElementById('top');
scrollTopBtn.addEventListener('click', function() {
window.scrollTo({top: 0, behavior: 'smooth'});
});
(Please refer to the attached HTML and CSS files for better understanding.)
The code is straightforward. We attached an event listener to a button to trigger the scroll top function.
The callback implemented the window.scrollTo()
method in its new form. We passed top:0
to scroll to the top, and we passed behavior: 'smooth'
to have a nice even animation effect.
You can see the working demo here:
Read in detail about the vanilla JS window.scrollTo()
method here.
Scroll to the Top of a Page Using the jQuery scrollTop()
Method
You can also scroll to the top of a page with the jQuery scrollTop()
method.
$("#top").on("click",function(){
$(window).scrollTop(0);
});
The logic is simple. We attached an event handler to listen for the click
event on our scroll to the top button.
The callback executed the $(window).scrollTop(0)
method and scrolled to the top of the page because we passed 0
as its argument.
You can see the working demo here:
Scroll to the Top of a Page by Animating With the jQuery animate()
Method
Like the vanilla JS solution above, we can animate scrolling to the top of the page with jQuery to improve the UX.
$("#top").on("click",function(){
$([document.documentElement,document.body]).animate({
scrollTop:0
},1000)
});
Let us dive into the logic here.
The callback to the event listener first selected the document.documentElement
(the root element ) or the document.body
. We need to pick both to ensure cross-browser compatibility.
(Hint: This is one reason why we use jQuery. More on that a bit later.)
We then ran the animate()
method on our selected object and changed the scrollTop
property to reach a 0
value (the page top.)
Finally, we pass the animation duration as the second argument to the callback. We passed 1000 milliseconds here, but you can choose any time span you like.
Here is the working output of this method:
Pro Tip: We use jQuery to ensure better cross-browser compatibility. Sometimes, UI can render weirdly in different browsers, and it can be tough to troubleshoot.
As jQuery is a professional front-end library, it has the advantage of ironing out such cross-browser incompatibility issues. So, if your vanilla JS solutions break in some browsers, you can instead use the jQuery version of the method.
One-Line Clever Pure HTML Scroll to the Top of the Page Hack
You can also scroll to the top of the page (without animation) with a single-line HTML hack.
<div>
<a href="#">To Top</a>
</div>
Here is the demo of the output.
Use the Old scrollTop
Property to Scroll to the Top of the Page
Some browsers have issues with the vanilla JS scrollTo()
method that we used above. For example, many users report that MS Edge does not always work well with this method,
So, you can instead use the older way with the scrollTop
property. The scrollTop
property sets the pixels you want to scroll an element vertically.
But, a special case of this property is when you apply it to the root or html
element, it moves the entire document.
Read about the scrollTop
property in detail here.
We can scroll to the top of a page with this property.
let scrollTopBtn = document.getElementById('top');
scrollTopBtn.addEventListener('click', function() {
document.getElementsByTagName('html')[0].scrollTop = 0;
});
The code is simple. The callback selected the html
element and set its scrollTop
property to 0
.
You can see the working demo below:
Custom Scroll to the Top of the Page Using JavaScript Animation Script
Finally, we have a nice little script to devise your custom scroll to top JavaScript animation. We use the setInteval()
and clearInterval()
functions to execute the animation effect.
let scrollTopBtn = document.getElementById('top');
scrollTopBtn.addEventListener('click', function() {
let customAnimScroll = window.setInterval(function() {
let topOffset = window.pageYOffset;
if (topOffset > 0) {
window.scrollTo({top: topOffset - 20});
} else {
clearInterval(customAnimScroll);
}
}, 20);
});
Let us break down the logic here.
We executed a setInterval()
function on the window
object inside the callback to the event handler on the scroll to top button. The setInterval
function ran the callback we passed to it repeatedly after a specific duration.
It also returned an ID which we stored to start/stop/modify that particular instance of the setInterval()
method. So, we ran the setInterval()
method and stored its ID in the customAnimScroll
variable.
The callback to the setInterval()
function stored the window
object’s pageYOffset
value in the topOffset
variable. This value is the number of pixels that the window
object is scrolled down.
Finally, we checked if the topOffset
value is greater than 0 (meaning that the window
is scrolled down by some pixels). If yes, we move the window
up by 20 pixels with the scrollTo
method we saw above in its new form.
Here, we chose to move 20 pixels up for every cycle, but you can choose any other number.
If the topOffset
value is not greater than 0, we have scrolled to the top of the window
. In this case, we ended the setInterval()
function by passing its ID we stored earlier in the customAnimScroll
variable to the clearInterval()
method.
You can see the working demo here: