How to Smooth Scroll in JavaScript
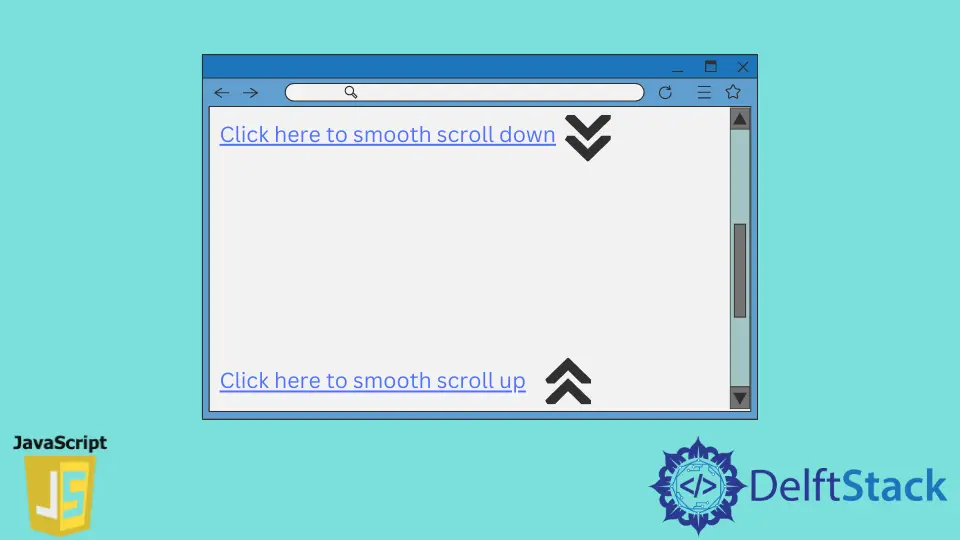
This article will discuss how to use the smooth scroll feature in JavaScript. For the example, we will make events that will call the scrolling function.
To enhance the navigation experience within the page, we use smooth scrolling, which is a user interface pattern. It is the change of position animatedly within the scrolling box of the page.
There are multiple ways to create and implement that scrolling pattern with the help of JavaScript, jQuery, and any other plugin, but right now, we will use JavaScript with jQuery solution for this feature.
Smooth Scroll in JavaScript
In JavaScript, we can call a function on click event that will change the current position of a webpage and will animatedly navigate to another position.
There is a default method animate()
in jQuery to pass the object from its containing position with scrollTop
, milliseconds as a timer, and a call back function called after scrolling is completed.
Example code:
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script>
$(document).ready(function(){
// smooth scrolling function on anchor tag
$("a").on('click', function(event) {
// if this.hash contain value
if (this.hash !== "") {
//default anchor click prevention
event.preventDefault();
// save hash
var myHash = this.hash;
// jQuery animate() method to call scroll
// 1200 milliseconds takes to scroll to the desired area
$('html, body').animate({
scrollTop: $(myHash).offset().top
}, 1200, function(){
// when done scroll add # to URL
window.location.hash = myHash;
});
} // End if
});
});
</script>
<style>
#section_top {
height: 600px;
border-style: solid;
border-width: 1px;
padding:5px
}
#section_down {
height: 600px;
border-style: solid;
border-width: 1px;
padding:5px
}
</style>
</head>
<body>
<h1>Delftstack learning</h1>
<h4>Smooth scrolling using JavaScript.</h4>
<div class="main" id="section_top">
<h2>Top Section</h2>
<a href="#section_down">Click here to Smooth Scroll to down Section</a>
</div>
<div class="main" id="section_down">
<h2>Down Section</h2>
<a href="#section_top">Click here to Smooth Scroll to top Section</a>
</div>
</body>
</html>
In the above HTML source code, we have created multiple sections using <div>
elements of HTML and CSS. We have defined IDs for the sections to change the position and implement styling.
We have created an anchor tag in both sections and defined href
to that element. At the head of the HTML page, we have included a jQuery link to use the jQuery default method animate()
.
We have called the scrolling function on click of the anchor tag in the script tag. Inside the scrolling function, we must check the hash value; if it has a value, then continue the execution.
We have prevented the default behavior of the anchor tag and saved the current hash. We have called the jQuery function animate()
with 1200 milliseconds timer and desired scroll position.
You can save the above source with an HTML extension and open it in any browser to see the result of smooth scrolling.