How to Implement Auto Scroll in JavaScript
-
Use
scrollBy()
WithsetInterval()
to Implement Auto Scroll in JavaScript -
Use
requestAnimationFrame
to Implement Auto Scroll in JavaScript - Use jQuery to Implement Auto Scroll in JavaScript
- Conclusion
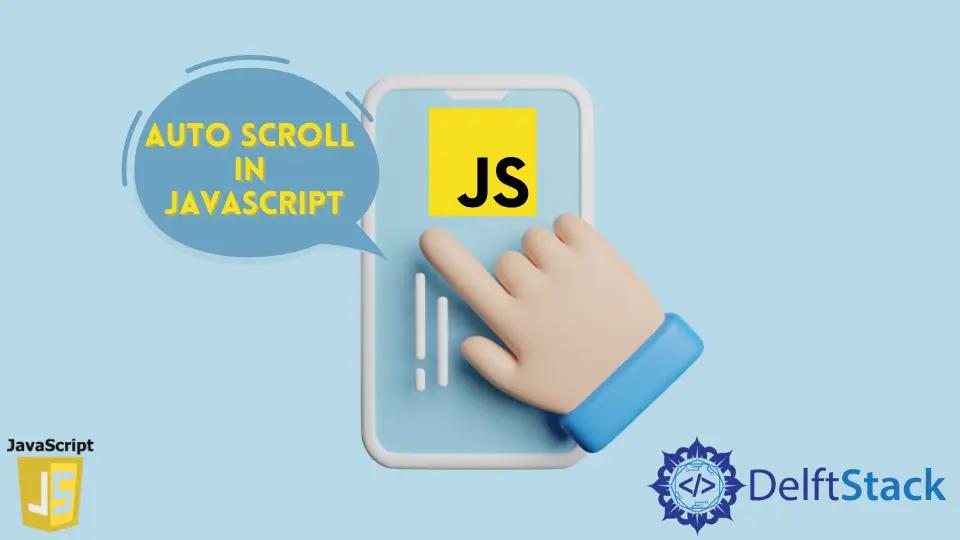
Auto-scrolling is a common feature in web applications that enhances the user experience by automatically moving the content within a webpage. It can be used for various purposes, such as displaying news feeds, social media updates, or long lists of items.
In this article, we’ll explore how to implement auto-scroll functionality in JavaScript.
Use scrollBy()
With setInterval()
to Implement Auto Scroll in JavaScript
In the JavaScript code below, the variables, scrollerID
, paused
, and interval
, are declared to manage the scrolling behavior on a webpage. Then, we defined a function called startScroll()
.
Within this function, the setInterval()
method is employed to repeatedly execute a specific action. This action involves scrolling the webpage by 2 pixels vertically using the window.scrollBy(0, 2)
method.
Additionally, startScroll()
contains logic to check if the user has reached the end of the page. This check is performed by comparing the sum of the viewport’s height and the current scroll position to the total height of the document.
If this condition is met, indicating that the user has scrolled to the bottom of the page, scrolling is halted.
Conversely, we also defined a function named stopScroll()
to clear the interval created by setInterval()
, effectively stopping the scrolling action initiated by startScroll()
.
To interact with these scrolling functionalities, an event listener is attached to the document.body
element, which listens for keypress events. Specifically, it listens for the Enter key (identified by its key code, 13).
When the Enter key is pressed, the code evaluates whether scrolling is currently paused (as determined by the value of the paused
variable). If scrolling is paused (paused == true
), the code initiates scrolling by invoking the startScroll()
function.
If scrolling is not paused, it halts the scrolling action by calling the stopScroll()
function. Crucially, the paused
variable is toggled accordingly, ensuring that the scrolling behavior can be controlled dynamically based on user input.
Let’s examine the code for further understanding.
HTML Code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<div id="one" style="height: 250px; background:pink">
<br>
  On page load the scrolling of the page will be enabled. <br>
  When pressed enter, the scroll will be halt. <br>
  And when pressed again it will scroll. <br>
  When the scroll meets the end of page, the scrolling function will stop. <br>
  And you can manually scroll up. An when needed press enter again to scroll to down. <br>
</div>
<div id="one" style="height: 250px; background:purple"></div>
<div id="one" style="height: 250px; background:cyan"></div>
<div id="one" style="height: 250px; background:lightgreen"></div>
</body>
</html>
JavaScript Code:
let scrollerID;
let paused = true;
let interval = 10;
function startScroll() {
let id = setInterval(function() {
window.scrollBy(0, 2);
if ((window.innerHeight + window.scrollY) >= document.body.offsetHeight) {
// end of page?
stopScroll();
}
}, interval);
return id;
}
function stopScroll() {
clearInterval(scrollerID);
}
document.body.addEventListener('keypress', function(event) {
if (event.which == 13 || event.keyCode == 13) {
// 'Enter' key
if (paused == true) {
scrollerID = startScroll();
paused = false;
} else {
stopScroll();
paused = true;
}
}
}, true);
Output:
As we can see, the page stops scrolling as it reaches the end of the page. And when manually scrolled to the top again, it reassures to play the scroller again.
Thus, the auto-scroll behavior can be implemented.
Use requestAnimationFrame
to Implement Auto Scroll in JavaScript
We can also implement auto-scrolling in JavaScript using the requestAnimationFrame
method. This is an efficient method for creating smooth animations and updates in web applications and is often used for tasks like smooth scrolling, animations, and other visual updates.
Here’s a complete working HTML and JavaScript code example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Auto-Scrolling Example</title>
<style>
/* Optional styles for demonstration */
body {
height: 2000px; /* Create enough content to scroll through */
}
.scroll-indicator {
position: fixed;
bottom: 20px;
right: 20px;
}
</style>
</head>
<body>
<!-- Content to scroll through -->
<div style="padding: 20px;">
<h1>Welcome to Auto-Scrolling Example</h1>
<br>
  On page load, the scrolling of the page will be enabled. <br>
<!-- Add more content here -->
</div>
<!-- Scroll to Top Indicator -->
<div class="scroll-indicator">
<a href="#" id="scrollToTop">Scroll to Top</a>
</div>
<script>
// JavaScript for auto-scrolling using requestAnimationFrame
let scrollPosition = 0;
const scrollSpeed = 2; // Adjust scroll speed as needed
let animationId; // Store the animation frame ID
function autoScroll() {
scrollPosition += scrollSpeed;
window.scrollTo(0, scrollPosition);
if (scrollPosition < document.body.scrollHeight) {
animationId = requestAnimationFrame(autoScroll);
}
}
animationId = requestAnimationFrame(autoScroll);
// Scroll to top when clicking the "Scroll to Top" link
document.getElementById('scrollToTop').addEventListener('click', function (e) {
e.preventDefault();
cancelAnimationFrame(animationId); // Stop the auto-scrolling
window.scrollTo({ top: 0, behavior: 'smooth' }); // Smoothly scroll to the top
});
</script>
</body>
</html>
In this example, we created a simple HTML document with a large amount of content to scroll through. We then included a Scroll to Top
link at the bottom of the page to demonstrate smooth scrolling.
The JavaScript code initiates auto-scrolling using the requestAnimationFrame
method. The autoScroll
function continuously updates the scroll position and uses requestAnimationFrame
to create a smooth scrolling effect.
When the Scroll to Top
link is clicked, we stop the auto-scrolling by canceling the animation frame with cancelAnimationFrame
. Then, we smoothly scroll to the top of the page using the window.scrollTo
method with the behavior: 'smooth'
option.
Use jQuery to Implement Auto Scroll in JavaScript
In addition to the methods discussed above, we can also implement auto-scrolling using jQuery. Below is a complete working HTML and JavaScript code example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Auto-Scrolling Example with jQuery</title>
<style>
/* Optional styles for demonstration */
body {
height: 2000px; /* Create enough content to scroll through */
}
.scroll-indicator {
position: fixed;
bottom: 20px;
right: 20px;
}
</style>
<!-- Include jQuery library (you can also use a CDN) -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<!-- Content to scroll through -->
<div style="padding: 20px;">
<h1>Welcome to Auto-Scrolling Example with jQuery</h1>
<br>
  On page load, the scrolling of the page will be enabled. <br>
<!-- Add more content here -->
</div>
<!-- Scroll to Top Indicator -->
<div class="scroll-indicator">
<a href="#" id="scrollToTop">Scroll to Top</a>
</div>
<script>
// JavaScript for auto-scrolling using jQuery
$(document).ready(function () {
// Function to enable auto-scrolling
function startAutoScroll() {
const scrollSpeed = 2; // Adjust scroll speed as needed
const $window = $(window);
const $document = $(document);
// Scroll function
function scroll() {
const currentScrollTop = $window.scrollTop();
$window.scrollTop(currentScrollTop + scrollSpeed);
// Continue scrolling until the end of the document is reached
if (currentScrollTop + $window.height() < $document.height()) {
requestAnimationFrame(scroll);
}
}
// Start scrolling
scroll();
}
// Start auto-scrolling when the page loads
startAutoScroll();
// Scroll to top when clicking the "Scroll to Top" link
$('#scrollToTop').click(function (e) {
e.preventDefault();
$('html, body').animate({
scrollTop: 0
}, 1000); // Adjust duration as needed
});
});
</script>
</body>
</html>
Within the JavaScript code, there is a function named startAutoScroll()
, which is responsible for enabling the auto-scrolling behavior. It operates by continuously scrolling down the web page at a rate determined by the scrollSpeed
variable.
This scrolling action continues until the bottom of the web page is reached.
There is also the scroll()
function that is nested within startAutoScroll()
. It calculates the current vertical scroll position of the page, incrementally increases it by the specified scrollSpeed
, and utilizes the requestAnimationFrame
method to sustain the scrolling action until the end of the web page is reached.
The initiation of auto-scrolling occurs automatically when the web page loads, thanks to the startAutoScroll()
function.
Additionally, an event handler is established for the Scroll to Top
link identified by #scrollToTop
. When this link is clicked, it triggers a smooth scrolling animation that smoothly navigates the web page back to the top. This animation lasts for a duration of 1000 milliseconds, which is equivalent to 1 second.
Conclusion
In summary, implementing auto-scroll functionality in JavaScript can be achieved using either scrollBy()
with setInterval()
, requestAnimationFrame
, or jQuery. The choice between these methods depends on your specific requirements and browser compatibility considerations.
With the right implementation, auto-scrolling can enhance user engagement and improve the user experience on your website.