How to Show/Hide Div onClick in JavaScript
-
Show and Hide an HTML
div
Using CSS -
Show and Hide an HTML
div
Using theonclick()
Function and JavaScript
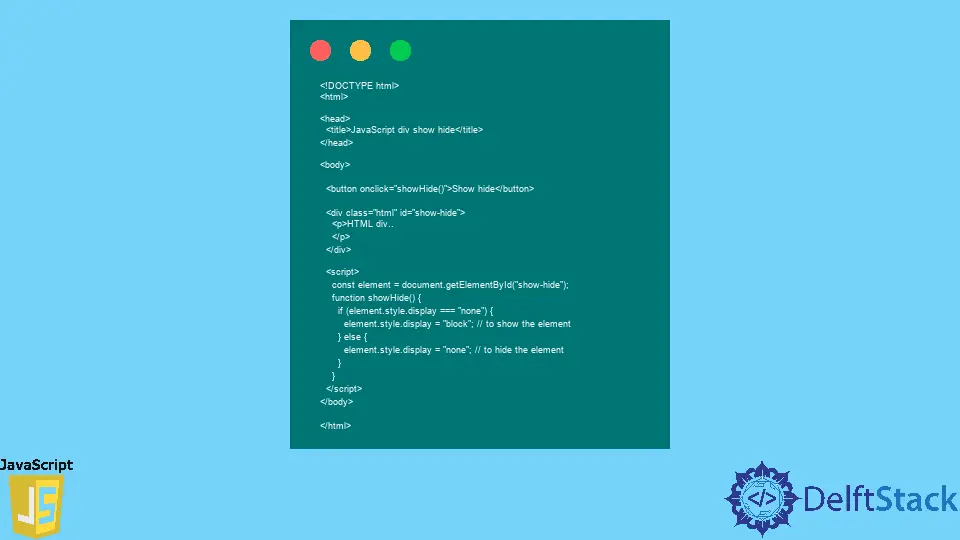
This article will discuss using CSS or the onclick()
function to show and hide an HTML div
in JavaScript.
Show and Hide an HTML div
Using CSS
A div
is a tag and element of HTML to define the section or divisions of a web page, and we can also add any content inside div
tags. A div
is used as a container of elements of HTML, and it can be assigned with CSS and customized with JavaScript.
We can toggle the div
section using CSS’s :target
keyword. The :target
keyword is linked with an HTML element which is the target element, and we can change its properties, and the properties will be applied to it when the element is clicked.
For example, we can show a specific div
when clicking a link. Let’s consider an example where we have three div
elements and want to show them when a specific link is clicked.
See the example code and output below.
<!DOCTYPE html>
<html>
<head>
<title>JavaScript div show hide</title>
<style>
#html-show-hide,
#css-show-hide,
#js-show-hide {
display: none;
}
#html-show-hide:target,
#css-show-hide:target,
#js-show-hide:target {
display: block;
}
</style>
</head>
<body>
<!--Nav-Bar-->
<a href="#html-show-hide">Show HTML</a>
<a href="#css-show-hide">Show CSS</a>
<a href="#js-show-hide">Show JS</a>
<!--HTML-->
<div class="html" id="html-show-hide">
<p class="text-html" id="htmlContent">HTML div..
</p>
</div>
<!--CSS-->
<div class="css" id="css-show-hide">
<p class="text-css" id="cssContent">CSS div..
</p>
</div>
<!--JS-->
<div class="js" id="js-show-hide">
<p class="text-js" id="jsContent">JS div..
</p>
</div>
</body>
</html>
Output:
In the above code, we have used the display
property of CSS to show and hide a div
element. If we want to hide the div
section, we have to set display
to none
and to show the section; we have to set display
to block
.
Inside the body
tag, we have created 3 div
elements and 3 links that will show a specific div
when we click on it. Each div
is attacked with a separate link using the href
attribute.
When we click on a link, the href
attribute will trigger the CSS attribute target
, which will change the property of the element whose name is attached to the target
attribute. In the above code, we have used the style
tag to write CSS inside the HTML file, but we can also create and link a separate CSS file.
In the above output, we can see only one div
, which is the CSS div
because we have clicked on the second link, and if we click on another link, the currently visible div
will hide, and the new div
will become visible on the page.
Show and Hide an HTML div
Using the onclick()
Function and JavaScript
In the above example, we have used the target
attribute of CSS to trigger the response of a click, but we can also use the onclick()
function, which will call a function specified inside the JavaScript file or the script
tag of HTML and the code inside the function will be executed.
<!DOCTYPE html>
<html>
<head>
<title>JavaScript div show hide</title>
</head>
<body>
<button onclick="showHide()">Show hide</button>
<div class="html" id="show-hide">
<p>HTML div..
</p>
</div>
<script>
const element = document.getElementById("show-hide");
function showHide() {
if (element.style.display === "none") {
element.style.display = "block"; // to show the element
} else {
element.style.display = "none"; // to hide the element
}
}
</script>
</body>
</html>
Output with show:
Output with hide:
In the above HTML code, we have created a div
section with the id show-hide
and a button to call the showHide()
function of JavaScript, which is present inside the script
tag. In the showHide()
function, we get the div
element using the document.getElementById()
function.
Firstly, we have used the if-else
statement to check the visibility of the div
using the style.display
attribute, and if the display
property is set to none
, we have to set it to block
, and if it is set to block
, we have to set it to none
. In the above output, we can see a button that will toggle the given div
.
If the div
is hidden, it will be visible when we press the button. If the div
is visible, it will hide when the button is pressed.
In the above code, we have used the script
tag to write the JavaScript code inside the HTML file, but we can also create and link a JavaScript file with the HTML file.