How to Remove Spaces From a String in JavaScript
-
Use
replace()
to Only Replace White Space in JavaScript String -
Use
replace()
to Replace All Spaces in JavaScript String -
Use
split()
andjoin()
Methods to Remove Spaces From a JavaScript String
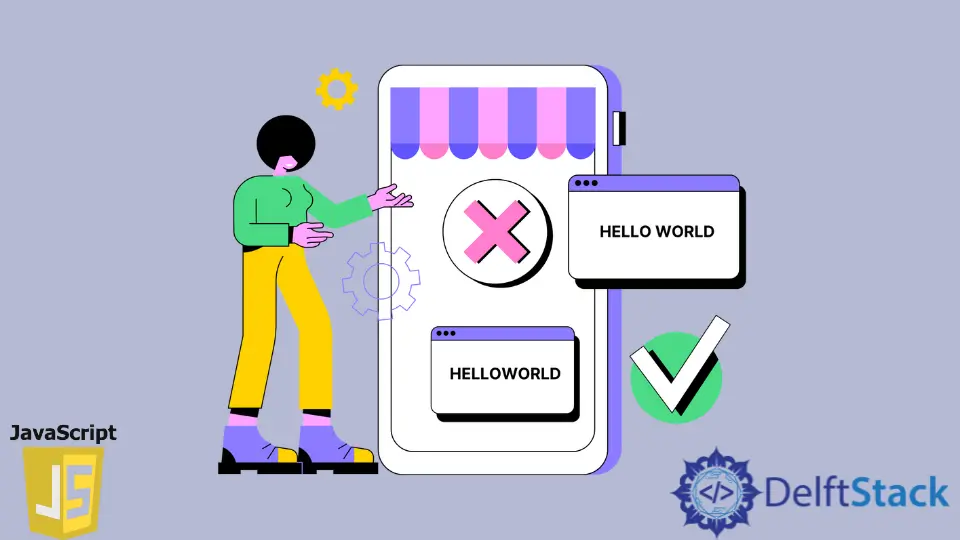
This article will introduce different ways to remove spaces from a string, especially how to remove tabs and line breaks. Every method below will have a code example, which you can run on your machine.
The replace()
method takes a specified value or a regular expression and replaces them in a given string. It returns a new string in which the specified values are replaced.
Use replace()
to Only Replace White Space in JavaScript String
string.replace(/ /g, '')
The regular expression contains a whitespace character (" "
), and the global property. It will search every space in a string and replace them with an empty string, as given in the second parameter.
Example
<!DOCTYPE html>
<html>
<head>
<title>
Remove spaces from a string using JavaScript
</title>
</head>
<body>
<h2>
Remove spaces from a string using JavaScript
</h2>
<p>
Original string is:
site/ delft stack .com/
</p>
<p>
New Sentence is:
<span class="outputString"></span>
</p>
<button onclick="removeSpacesFromString()">
Clean Spaces
</button>
<script type="text/javascript">
const removeSpacesFromString = () => {
let text1 =
"site/ delft stack .com/";
let text2 =
text1.replace(/ /g, "");
document.querySelector('.outputString').textContent
= text2;
}
</script>
</body>
</html>
Output:
Use replace()
to Replace All Spaces in JavaScript String
string.replace(/\s+/g, '')
The regular expression pattern \s
refers to any whitespace symbol: spaces, tabs, and line breaks.
Example:
<script type="text/javascript">
const removeSpacesFromString = () => {
let text1 =
"site/ delft stack .com/";
let text2 =
text1.replace(/\s+/g, '');
document.querySelector('.outputString').textContent
= text2;
}
</script>
Use split()
and join()
Methods to Remove Spaces From a JavaScript String
The split()
method splits a string into an array and returns the new array.
The join()
method returns a new string by concatenating all of the elements in an array.
We will use the split
method to split the string into an array with the white space character " "
as the delimiter, and then use the join
method to convert the array into a string. It removes the white spaces using these two methods.
Example:
<html>
<head>
<title>
Remove spaces from a string using JavaScript
</title>
</head>
<body>
<h2>
Remove spaces from a string using JavaScript
</h2>
<p>
Original string is:
site/ delft stack .com/
</p>
<p>
New Sentence is:
<span class="outputString"></span>
</p>
<button onclick="removeSpacesFromString()">
Clean Spaces
</button>
<script type="text/javascript">
const removeSpacesFromString = () => {
let text1 =
"site/ delft stack .com/";
let text2 =
text1.split(" ").join("");
document.querySelector('.outputString').textContent
= text2;
}
</script>
</body>
</html>