How to Remove Index From Array in JavaScript
-
Use the
splice()
Function to Remove a Specific Element From JavaScript Array -
Use
Array.filter()
to Remove a Specific Element From JavaScript Array -
Use the
Underscore.js
Library to Remove a Specific Element From JavaScript Array -
Use the
Lodash
Library to Remove a Specific Element From JavaScript Array
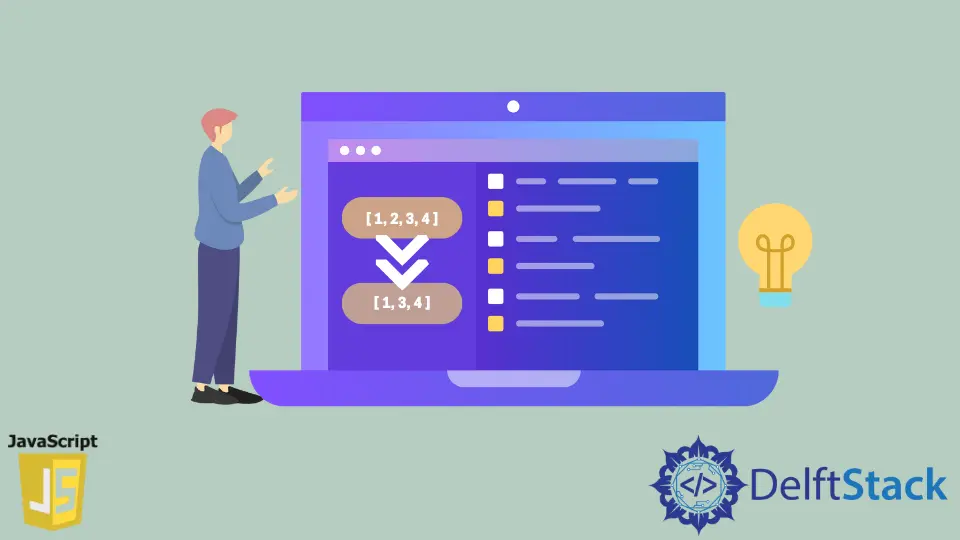
This tutorial teaches how to remove a specific element from an array in JavaScript.
Use the splice()
Function to Remove a Specific Element From JavaScript Array
The splice()
method can modify the array’s content by adding/removing elements. It takes the following 3 arguments :
index
: An integer value specifying the position to add/remove elements. We can even specify an index from the back of the array by using negative indices.howmany
: It is an optional parameter. It specifies how many items will be removed from the array. If it is set to0
, then no items are removed.item1, item2, ... ,itemx
: The items to be added to the array.
const array = [1, 2, 3, 4, 5];
const index = array.indexOf(3);
if (index > -1) {
array.splice(index, 1);
}
console.log(array);
Output:
[1, 2, 4, 5]
In the above code, we first find the index of the element we want to remove and then use the splice()
method to remove the array element.
Use Array.filter()
to Remove a Specific Element From JavaScript Array
The filter
methods loop through the array and filter out elements satisfying a specific given condition. We can use it to remove the target element and keep the rest of them. It helps us to remove multiple elements at the same time.
var toRemove = 1;
var arr = [1, 2, 3, 4, 5];
arr = arr.filter(function(item) {
return item !== toRemove
});
console.log(arr)
Output:
[2, 3, 4, 5]
We use the filter
function to keep every element that is not equal to the element to be removed and assign the newly formed array to the original array.
Use the Underscore.js
Library to Remove a Specific Element From JavaScript Array
Underscore.js
is a very helpful library that provides us a lot of useful functions without extending any of the built-in objects. To remove target elements from a JavaScript array, we have to use the without()
function. This function returns a copy of the array with all copies of the target element removed.
const arr = [1, 2, 1, 0, 3, 1, 4];
arr = _.without(arr, 0, 1);
console.log(arr);
Output:
[2, 3, 4]
In the above code, we pass the array and the elements to be removed 0
and 1
to the without
function. It returns a new array with these elements removed, which we store again in arr
.
Use the Lodash
Library to Remove a Specific Element From JavaScript Array
Lodash
is a great library that allows us to import only the functions we need and not the complete library. It has a function named remove()
, which can remove a specific element from the array. This function takes the array and a function to check for conditions matching the element to be removed from the array.
var arr = [1, 2, 3, 4];
var greater = _.remove(arr, function(n) {
return n > 2;
});
console.log(arr)
Output:
[1,2]
In the above code, we pass the array and a function checking if elements are greater than 2
to the lodash
library’s remove
function. It removes all elements greater than 2
from the array.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedInRelated Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript