How to Remove Item From Array by Value in JavaScript
-
Remove an Item From an Array by Value Using the
splice()
Function in JavaScript -
Remove an Item From an Array by Value Using the
filter()
Function in JavaScript
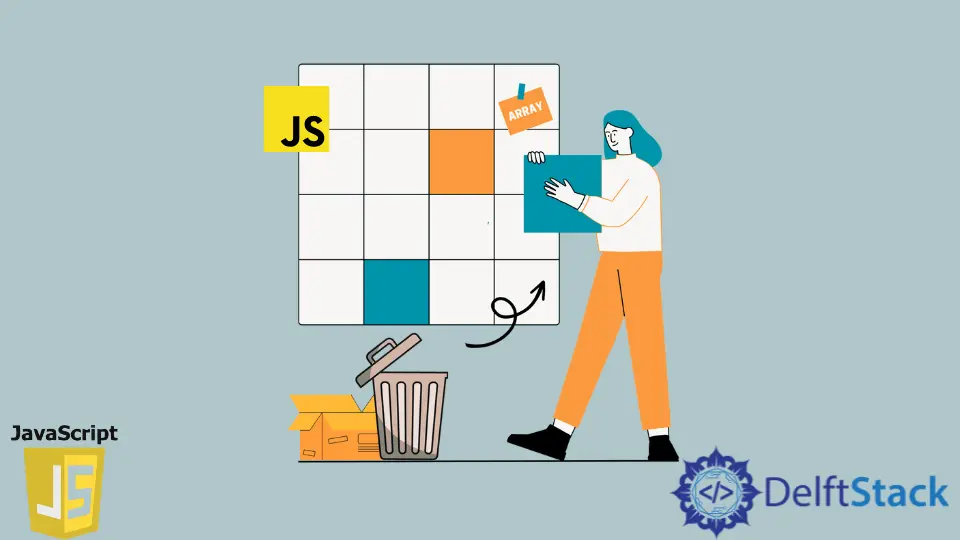
This tutorial will discuss how to remove an item from an array by value using the splice()
and filter()
functions in JavaScript.
Remove an Item From an Array by Value Using the splice()
Function in JavaScript
To remove an item from an array by value, we can use the splice()
function in JavaScript. The splice()
function adds or removes an item from an array using the index. To remove an item from a given array by value, you need to get the index of that value by using the indexOf()
function and then use the splice()
function to remove the value from the array using its index. For example, let’s create an array with three string values and remove one string value using the splice()
and indexOf()
function. See the code below.
var myArray = ['one', 'two', 'three'];
var myIndex = myArray.indexOf('two');
if (myIndex !== -1) {
myArray.splice(myIndex, 1);
}
console.log(myArray)
Output:
["one", "three"]
In the above code, we are using an if
statement to check if the value is present in the array or not. If the value is present, its index will not be -1; otherwise, it will be -1. The indexOf()
function returns the index of the given value, and if it’s not present in the array, the function will return -1. If the value is present in the array, we will use the splice()
function to remove 1 value present at the myIndex
. We can also remove more than one value from the array by defining it as a second argument in the splice()
function. The console.log()
function will show the new array after the item is removed from the array on the console.
Remove an Item From an Array by Value Using the filter()
Function in JavaScript
To remove an item from an array by value, we can use the filter()
function in JavaScript. The filter()
function is used to filter values from a given array by applying a function defined inside the filter()
function on each value of the array. In our case, we will define a function inside the filter()
function, which will return all the values to accept the value we want to remove from the array, and the result will be stored in a new array. For example, let’s create an array with three string values, and then using the filter()
function, we will create another array that will have all the values of the first array except the value that we want to remove. In this way, our original array will not be changed. See the code below.
var myArray = ['one', 'two', 'three'];
var newArray = myArray.filter(function(f) {
return f !== 'two'
})
console.log(newArray)
Output:
["one", "three"]
In the above code, we removed the value two
from the array and saved the result in the variable newArray
, which will be shown on the console.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript