How to Remove All Event Listeners in JavaScript
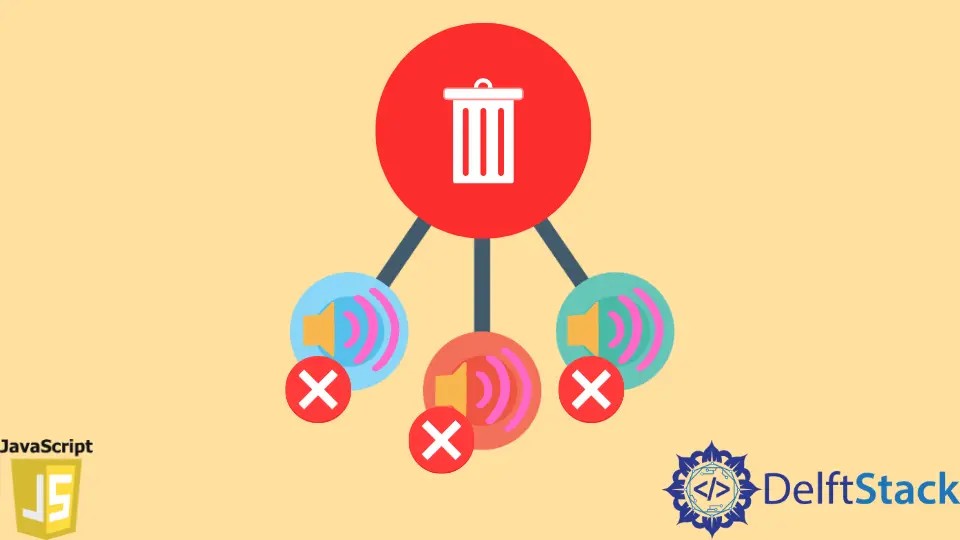
Today’s post will teach about removing all the event listeners in JavaScript.
Remove All Event Listeners in JavaScript
The addEventListener()
method of the EventTarget
interface configures a function to be called whenever the specified event is delivered to the target.
The addEventListener()
method works by adding a function or object that implements the EventListener
to the list of event listeners for the event type specified in the EventTarget
for which it is called.
If the function or object is already in the list of event listeners for that target, the function or object is not added a second time.
EventTarget
interface’s removeEventListener()
method removes an event listener that was previously registered from the target using EventTarget.addEventListener()
.
Calling removeEventListener()
with arguments that don’t identify an EventListener
currently registered in the EventTarget
has no effect.
The event will not dispatch if an EventListener
is removed from an EventTarget
while another listener on the target is processing an event. However, it can be repositioned.
Calling removeEventListener
is easy if only one or two listeners are registered for specific elements. What if more than 10 listeners are registered, and you want to remove all event listeners.
It will take effort; we will learn another method to perform this tedious operation to overcome this.
Let’s look at the example below.
<div>
<button id="grt-btn">Hello World!</button>
<button onclick="removeListeners()">Remove listeners</button>
</div>
document.getElementById('grt-btn').addEventListener(
'click', () => {console.log('firstClick')}, false);
document.getElementById('grt-btn').addEventListener(
'blur', () => {console.log('Blur event')}, false);
document.getElementById('grt-btn').addEventListener(
'focus', () => {console.log('focus event')}, false);
function removeListeners() {
const oldBtnElement = document.getElementById('grt-btn');
const newBtnElement = oldBtnElement.cloneNode(true);
oldBtnElement.parentNode.replaceChild(newBtnElement, oldBtnElement);
console.log('Removed all listners')
}
In the example above, we have defined 2 buttons. The first button is the one that will attach listeners to it.
The second button is the one that will remove listeners from the first button.
We have defined three events listeners; as soon as you click on the Hello World
button, it will trigger the focus
and click
events. Try to navigate to another tab; it will trigger the blur
event.
And finally, click on the Remove listeners
button, which will clone the Hello World
button. The final step is to replace the child node of the div
element with cloneNode
.
This will automatically remove all event listeners associated with the button earlier.
If you try to click on the Hello World
button again, it won’t trigger any events.
Output:
"focus event"
"firstClick"
"Blur event"
"focus event"
"Blur event"
"Removed all listeners"
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn