How to Read File Line by Line in JavaScript
- Use Plain JavaScript to Read a Local File Line by Line in JavaScript
-
Use Node.js
readline
Module to Read a Local File in JavaScript -
Use the
line-reader
Module in Node.js to Read a Local File in JavaScript
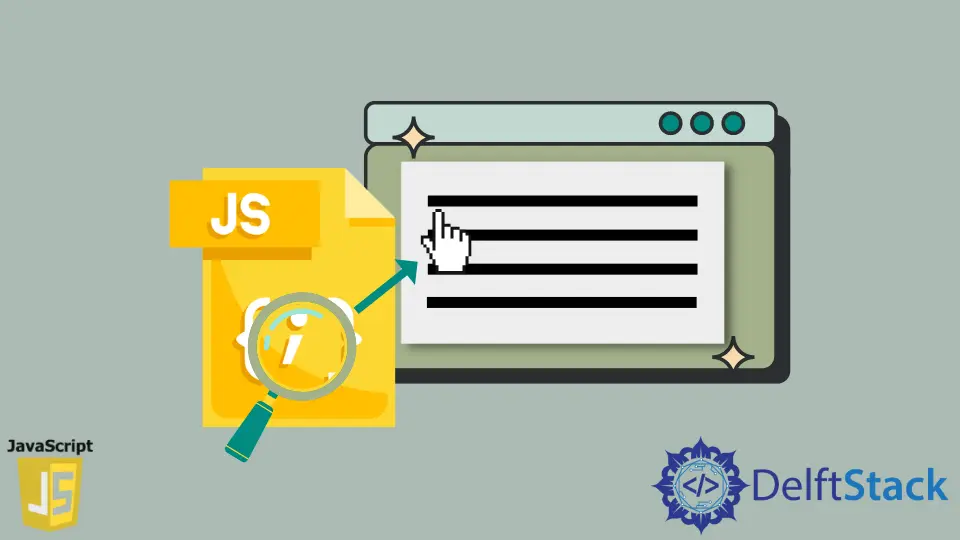
This article will introduce ways to read through the file using JavaScript using Vanilla JS and JavaScript framework Node.js.
Use Plain JavaScript to Read a Local File Line by Line in JavaScript
We can create a simple JavaScript function to read the local file put in as the HTML Input.
We can use the input
HTML tag to upload the file, and the function FileReader()
to read the content from the file line by line with the use of function
Code Example:
<input type="file" name="file" id="file" />
document.getElementById('file').onchange = function() {
var file = this.files[0];
var reader = new FileReader();
reader.onload = function(progressEvent) {
console.log(this.result);
};
reader.readAsText(file);
};
Here, the input field is selected by the getElementById
method, which will trigger the function whenever it is changed (whenever a file is selected). We create a new instance of object FileReader()
. When the instance reader.onload
is triggered, a function with parameter progressEvent
is called, and we can print the entire content of the file on console as console.log(this.result)
.
We can extend the function to read the content of the file line by line, as shown below.
document.getElementById('file').onchange = function() {
var file = this.files[0];
var reader = new FileReader();
reader.onload = function(progressEvent) {
var fileContentArray = this.result.split(/\r\n|\n/);
for (var line = 0; line < lines.length - 1; line++) {
console.log(line + ' --> ' + lines[line]);
}
};
reader.readAsText(file);
};
The extended snippet from the previous method uses split()
to split the content read by the result variable and store it into an array fileContentArray
variable. Then for
loop is used to loop through each line of the fileContentArray
variable and print the file contents line by line.
For testing purposes, we create a demo.txt
file that contains the following content.
Line 1
Line 2
Line 3
Line 5
Now, we can see that the file has five lines, and on uploading the file from the HTML Input element, we can see the file’s content as in the output below.
Output:
1 --> Line 1
2 --> Line 2
3 --> Line 3
4 -->
5 --> Line 5
We can ignore content 1 -->
as it was to visualize the file’s line number.
Use Node.js readline
Module to Read a Local File in JavaScript
We should make sure we have Node installed to use this method. We can check that by typing node -v
in the terminal or command prompt. We can now use the readline
module to read the file’s content easily. We create a file, app.js,
and on the first line of the file app.js
, we import the module as shown below.
const readline = require('readline');
const fs = require('fs');
Since the readline
module is built into Node.js, we don’t explicitly install it. We can use the fs
module to create a readable stream. It is because the readline
module is only compatible with Readable Streams.
Example Code:
const readLine = require('readline');
const f = require('fs');
var file = './demo.txt';
var rl = readLine.createInterface(
{input: f.createReadStream(file), output: process.stdout, terminal: false});
rl.on('line', function(text) {
console.log(text);
});
We can save the file app.js
into the same folder as file demo.txt
and run with the command node app.js
.
Output:
Line 1
Line 2
Line 3
Line 5
Use the line-reader
Module in Node.js to Read a Local File in JavaScript
We can use the Node.js line-reader
module to read the file in JavaScript. The module is open source, and we need to install it with the commands npm install line-reader --save
or yarn add line-reader
.
Reading the content of a file using the line-reader
module is easy as it provides the eachLine()
method. It lets us read the file line by line. We can import it at the top of our file app.js
as, const lineReader = require('line-reader')
.
The eachLine()
method takes a callback function with two arguments. The arguments are line
and last
. The option line
stores the content, and the option last
tells if the line read is the last line in the file. The second option represents a boolean value.
Example code:
const lineReader = require('line-reader');
lineReader.eachLine('./demo.txt', (line, last) => {
console.log(line);
})
We can save the file app.js
to the folder where the file demo.txt
is located, or we can set the path to the file explicitly, which will read the content of the file line by line prints the output to the console.
Output:
Line 1
Line 2
Line 3
Line 5