How to Pick Random Value From Array in JavaScript
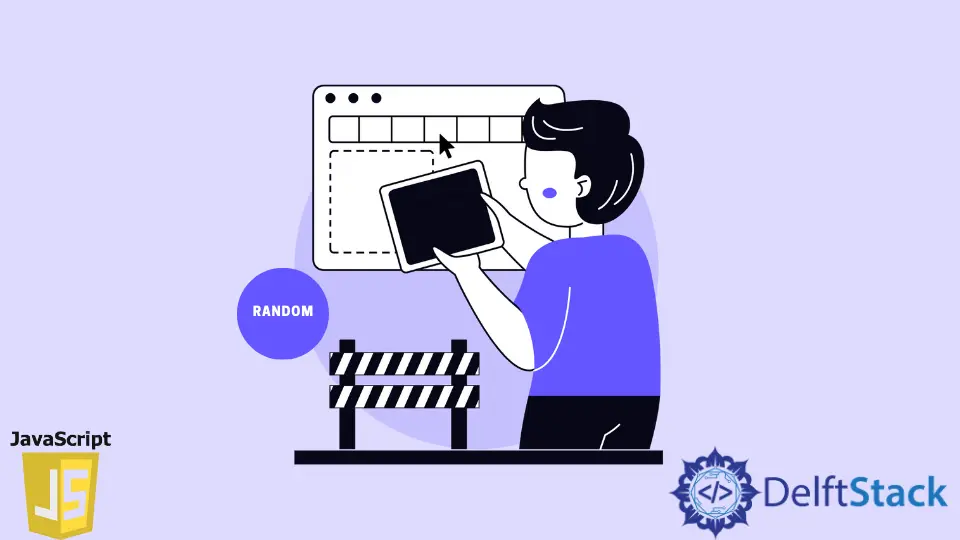
This tutorial will discuss how to pick a random value from an array using the Math.random()
function in JavaScript.
Pick a Random Value From an Array Using the Math.random()
Function in JavaScript
We can pick a value from a given array by using its index in JavaScript. To pick a random value from a given array, we need to generate a random index in the range of 0 to the length of the array. We can generate a random value by using the Math.random()
function and to set the range of this random value, we have to multiply it with the length of the array which we can get by using the length
function.
The random value generated from the Math.random()
function is a floating-point value. To convert the floating-point value to integer, we have to use the Math.floor()
function. The Math.floor()
function converts a floating-point number to an integer which will be less than the given number. For example, let’s create an array of five values and pick one random value from it and show it on the console. See the code below.
var myArray = ['one', 'two', 'three', 'four', 'five'];
var rand = Math.floor(Math.random() * myArray.length);
var rValue = myArray[rand];
console.log(rValue)
Output:
two
In the above code, the random index will be stored in the variable rand
, and using this index we can pick a random value from the array which will be stored in the variable rValue
. You can also use the bitwise NOT
operator ~~
or bitwise OR
operator |
instead of the Math.floor()
function to convert the floating-point number to an integer. Using bitwise operators is faster but it may not work for an array containing millions of values. For example, let’s generate a random number using the bitwise NOT
operator. See the code below.
var myArray = ['one', 'two', 'three', 'four', 'five'];
var rand = ~~(Math.random() * myArray.length);
var rValue = myArray[rand];
console.log(rValue)
Output:
one
Now, let’s generate a random number using the bitwise OR
operator. See the code below.
var myArray = ['one', 'two', 'three', 'four', 'five'];
var rand = Math.random() * myArray.length | 0;
var rValue = myArray[rand];
console.log(rValue)
Output:
three
If you run the code again the output will change. You can also make a function to pick random values from a given array so that you don’t have to rewrite all the code. For example, let’s make a function to pick random values from a given array and test it with an array. See the code below.
function RandArray(array) {
var rand = Math.random() * array.length | 0;
var rValue = array[rand];
return rValue;
}
var myArray = ['one', 'two', 'three', 'four', 'five', 'six'];
var rValue = RandArray(myArray);
console.log(rValue)
Output:
six
If you run the code again the output will change. Now, to pick a random value from an array, you only have to call the RandArray()
function.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript