How to List Object Properties in JavaScript
-
List Properties of an Object Using the
for...in
Loop in JavaScript -
List Properties of an Object Using the
Object.keys()
Function in JavaScript -
List the Properties of an Object With
Object.getOwnPropertyNames()
in JavaScript
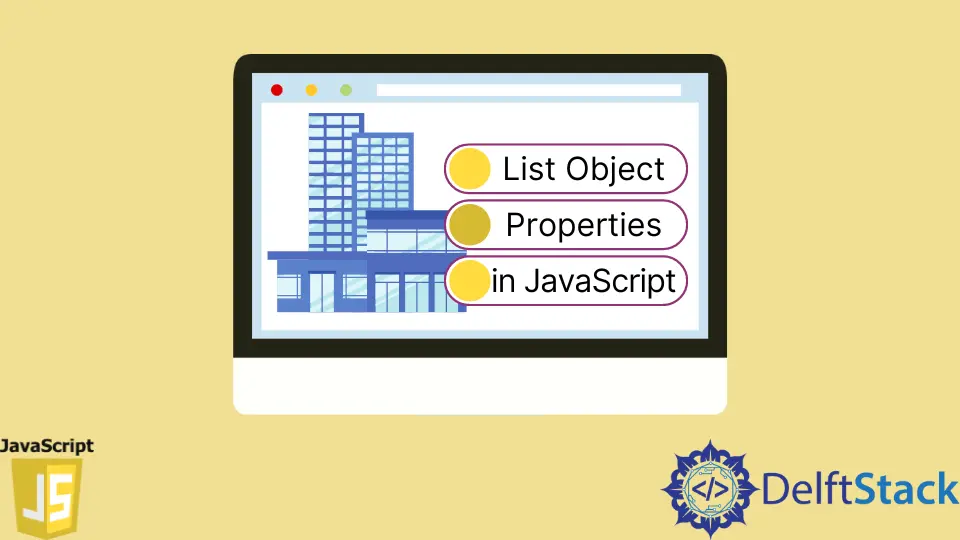
JavaScript provides various methods to work with Object like Object.values()
and Object.prototype.valueOf()
to work with object values, Object.keys()
and Object.prototype.hasOwnProperty()
to work with object keys.
User can perform any action on Object keys and properties. This tutorial explains listing object properties using the for...in
loop, Object.getOwnPropertyNames()
, and the Object.keys()
function in JavaScript.
List Properties of an Object Using the for...in
Loop in JavaScript
To list the properties of an object, you can use the for...in
loop in JavaScript. The for...in
loop iterates over the enumerable properties of an object.
These are properties of an object whose enumerable
flag is set to true. This includes inherited enumerable properties. However, this loop ignores the non-enumerable properties whose enumerable
flag is set to false.
For example, let’s create an object with three properties and list it using the for...in
loop. See the code below.
Syntax:
for (variable in object) {
statement
}
This function iterates the object
, and the variable
is assigned a different property name with each iteration. This method only extracts enumerable properties from an object
.
For more information, read more about the for...in
method.
const inputObject = {
id: 42,
name: 'John Doe',
address: {city: 'Mumbai', country: 'India'}
};
for (const property in inputObject) {
console.log(property);
}
In the sample code block above, we have defined three properties of an object - id
, name
, and address
. When you iterate the inputObject
, all properties in the inputObject
are checked.
The output of the code block above will look like the one shown below.
Output:
id
name
address
List Properties of an Object Using the Object.keys()
Function in JavaScript
This is a built-in method provided by JavaScript that is used to extract the properties or keys of an object. It iterates the object and returns an array of enumerable properties.
Non-enumerable properties are properties of objects that do not appear in the loop when the respective object is passed with Object.keys()
or for ...in
in the loop.
Syntax:
Object.keys(object);
This function takes an object
as an input, and it is a mandatory parameter. This method only extracts enumerable properties from an Object
.
For more information, read more about the keys
method.
const inputObject = {
id: 42,
name: 'John Doe',
address: {city: 'Mumbai', country: 'India'}
};
Object.defineProperty(
inputObject, 'salary', {value: '30,000$', enumerable: false})
console.log(Object.keys(inputObject));
In the previous example, we defined three properties of an object ID
, name
, and address
. When you pass inputObject
, all properties of the inputObject
are checked.
The output of the code block above will look like the one shown below.
Output:
["id", "name", "address"]
List the Properties of an Object With Object.getOwnPropertyNames()
in JavaScript
This is a built-in method provided by JavaScript used to extract the properties or keys of an object. It iterates the object and returns an array of properties, including non-enumerable properties.
Syntax:
Object.getOwnPropertyNames(object);
This function takes a mandatory parameter object
as an input. This method only extracts the enumerable and non-enumerable properties of an Object
and not the values.
For more information, read more about the getOwnPropertyNames
method.
The main difference between getOwnPropertyNames()
and Object.keys()
is that getOwnPropertyName()
returns both the properties enumerable and non-enumerable. Whereas the Object.keys()
only returns enumerable keys.
Non-enumerable properties can be created using Object.defineProperty
. An enumerable
flag is set to true
when the property initializer initializes properties.
You can use the propertyIsEnumerable()
method to check whether an object contains enumerable or non-enumerable properties.
const inputObject = {
id: 42,
name: 'John Doe',
address: {city: 'Mumbai', country: 'India'}
};
Object.defineProperty(
inputObject, 'salary', {value: '30,000$', enumerable: false})
console.log(Object.getOwnPropertyNames(inputObject));
In the previous example, we defined three properties of an object ID
, name
, and address
. When you pass inputObject
, all properties and non-enumerable properties of the inputObject
are checked.
The output of the code block above will look like the one shown below.
Output:
["id", "name", "address", "salary"]
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn