How to Count Number of Keys in Object in JavaScript
-
Use
Object.keys()
to Count Number of Keys Inside Object in JavaScript -
Use
Map()
to Count Number of Keys Inside Object in JavaScript
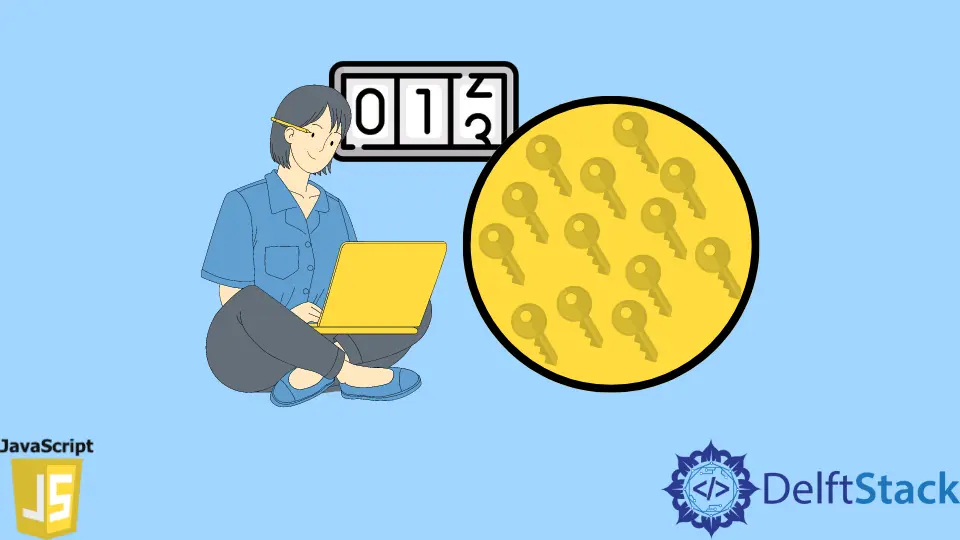
In lots of different programming languages, objects in JavaScript are often compared to real-lifestyle objects. The concept of objects in JavaScript is similar to that of objects in real life.
In JavaScript, an object is an independent entity. This entity has its properties and type.
Compare it to a mobile or laptop, for example. A mobile/laptop is an object with properties.
A mobile/laptop has a color, design, weight, material, OS, etc. Similarly, JavaScript objects can have properties that define their properties.
In today’s post, we’ll learn how to count the number of keys inside objects in JavaScript.
Use Object.keys()
to Count Number of Keys Inside Object in JavaScript
A JavaScript object has associated properties. A property of an object can be declared as a variable attached to the Object
.
The properties of an object define the properties of the Object
. You access an object’s properties with a simple dot notation.
This method returns an array of enumerable property names of a given object, repeated in a similar order as a normal loop would.
Object.keys()
returns an array. This array holds the elements that are strings corresponding to enumerable properties found directly on the Object
.
The order of the properties is the same as that provided by manually scrolling through the object properties.
Syntax:
Object.keys(inputObject)
The inputObject
is the input parameter whose enumerable’s own properties are to be returned. It returns an array of strings representing all enumerable properties of the specified Object
.
You can find more information about Object.keys
in the documentation for Object.keys()
.
Example:
const object = {
fruit: 'apple',
vegetable: 'tomato'
} console.log(Object.keys(object).length)
When you run the above code in any browser, you will get the following output.
Output:
2
Use Map()
to Count Number of Keys Inside Object in JavaScript
The Map
object contains key and value pairs similar to the JavaScript Object
, but it remembers the original insertion order of the keys. Objects and primitive values can be used either as a key or value.
A Map
object iterates through its elements in the order of insertion: a for...of
loop of JavaScript returns an array of keys, values similar to the JavaScript objects for each iteration.
Object
and map
share the same properties: both allow you to perform operations like set keys to values, delete keys, retrieve those values, and detect if something is stored in a key. For this reason, Object
was used as Map
in the past.
The number of elements is easily obtained from its size property. The number of elements in an object must be determined manually.
Essentially, Map
keeps track of its size, so we’re just returning a numeric field. It’s much, much faster than any other method.
Once you have control over the object, convert it to a map object. Find more information about Map
in the documentation for Map()
.
Example:
const testMap = new Map()
testMap.set('fruit', 'apple')
testMap.set('vegetable', 'tomato')
console.log(testMap.size)
In the above JavaScript example, we are creating a new instance of the Map
object. Once the instance is created, we will set the object’s properties.
When you run the above code in any browser, you will get the following output.
Output:
2
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn