How to Loop Through an Array in JavaScript
-
Use the
for
Loop to Loop Through an Array in JavaScript -
Use the
while
Loop to Loop Through an Array in JavaScript -
Use the
do...while
Loop to Loop Through an Array in JavaScript -
Use the
forEach
Loop to Loop Through an Array in JavaScript -
Use
for...of
to Loop Through an Array in JavaScript -
Use
for...in
to Loop Through an Array in JavaScript -
Use the
map
Method to Loop Through an Array in JavaScript -
Use the
reduce
Method to Loop Through an Array in JavaScript -
Use the
filter
Method to Loop Through an Array in JavaScript -
Use
some
to Loop Through an Array in JavaScript -
Use
every
to Loop Through an Array in JavaScript
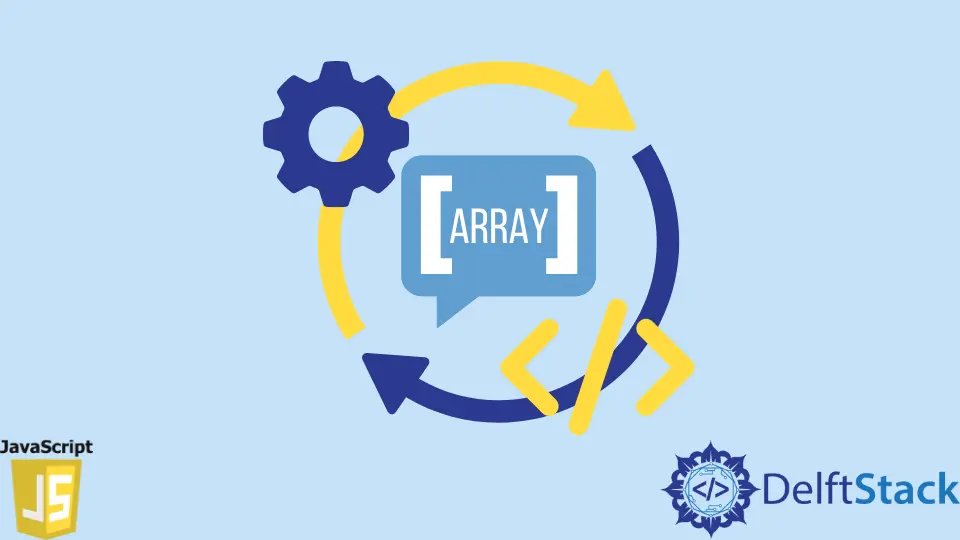
This tutorial explains how to loop through an array in JavaScript. We can do this using traditional methods in Vanilla JavaScript like for
loop and while
loop or using the newer methods introduced by ES5, ES6 like forEach
, for ... of
, for ... in
, and many other methods depending on our use case and speed/efficiency of code.
Use the for
Loop to Loop Through an Array in JavaScript
The traditional for
loop is similar to the for
loop in other languages like C++, Java, etc. It has 3 parts:
- The initialization of variables/iterators is executed before the execution of for loop.
- The condition to check every time before the loop is executed.
- The step to perform every time after loop execution.
const arr = [1, 2, 3, 4, 5, 6];
var length = arr.length;
for (var i = 0; i < length; i++) {
console.log(arr[i]);
}
Output:
1
2
3
4
5
6
We take an iterator i
and loop over the array by using a for
loop that increments i
by 1
after every iteration and moves it to the next
element.
Use the while
Loop to Loop Through an Array in JavaScript
The while
loop is very simple because it has a condition that it checks after every iteration, and unless this condition is satisfied, it keeps on executing.
const arr = [1, 2, 3, 4, 5, 6];
var length = arr.length;
let i = 0;
while (i < length) {
console.log(arr[i]);
i++;
}
Like the for
loop, we take iterator i
and increase it till the array’s length to loop through all elements.
Use the do...while
Loop to Loop Through an Array in JavaScript
The do...while
loop is the same as the while
loop except for the fact that it is executed at least once even if the loop condition is false. So, we have to be careful while writing this loop.
const arr = [1, 2, 3, 4, 5, 6];
var length = arr.length;
var i = 0;
do {
console.log(arr[i]);
i++;
} while (i < length);
Use the forEach
Loop to Loop Through an Array in JavaScript
ES5 introduced forEach
as a new way to iterate over arrays. forEach
takes a function as an argument and calls it for every element present inside the array.
const arr = [1, 2, 3, 4, 5, 6];
arr.forEach(item => console.log(item));
Here, we have written an arrow function to print out the argument passed into the function and given that function to forEach
to iterate over array and print that element.
Use for...of
to Loop Through an Array in JavaScript
ES6 introduced the concept of iterable objects and provided for ... of
that can be used to iterate Array objects.
let arr = [1, 2, 3, 4, 5, 6];
for (const item of arr) {
console.log(item);
}
We use it as a normal loop but easily iterate a wide range of objects and not just arrays.
Use for...in
to Loop Through an Array in JavaScript
We can iterate arrays using for ... in
, but it is not recommended because it enumerates the object’s properties. It enumerates even the methods attached to Array.prototype
in addition to the array elements.
var arr = [1, 2, 3, 4, 5, 6];
for (var i in arr) {
console.log(arr[i]);
}
Use the map
Method to Loop Through an Array in JavaScript
The map
method loops over the array, uses the callback function on each element, and returns a new array i.e. it takes input an array and maps it to a new array with computations performed by the callback function.
arr = [1, 2, 3, 4, 5, 6];
const square = (x) => {
return Math.pow(x, 2);
} mapped = arr.map(square);
console.log(arr);
console.log(mapped);
Here we iterated over the input array and formed an array with squares of elements inside the array.
Use the reduce
Method to Loop Through an Array in JavaScript
The reduce
method loops over the array and calls the reducer
function to store the value of array computation by an accumulator’s function. An accumulator is a variable remembered throughout all iterations to store the accumulated results of looping through an array.
const arr = [1, 2, 3, 4];
const reducer = (accumulator, curr) => accumulator + curr;
console.log(arr.reduce(reducer));
Here we loop over the array and sum all the elements inside an accumulator.
Use the filter
Method to Loop Through an Array in JavaScript
The filter
methods loop through the array and filter out elements satisfying a specific given condition.
const fruits =
['apple', 'orange', 'pomegranete', 'banana', 'pear', 'blueberry'];
const result = fruits.filter(word => word.length > 6);
console.log(result);
Here we loop through the array and check if the given fruit name’s length is larger than 6. If yes, then we include it in our result array, i.e. we filter out the required elements.
Use some
to Loop Through an Array in JavaScript
It is used to loop through the array and check if a given condition is satisfied by at least one of the elements present inside the array.
array = [1, 2, 3, 4, 5, 6];
const under_three = x => x < 3;
console.log(array.some(under_three));
Here we use some
to check if any element present inside the array that is less than 3
. It returns a boolean value true
or false
.
Use every
to Loop Through an Array in JavaScript
It is used to loop through the array and check if a given condition is satisfied by all the elements inside an array.
array = [1, 2, 3, 4, 5, 6];
const under_three = x => x < 3;
console.log(array.every(under_three));
Here we use every
to check if all the elements present inside the array are less than 3
or not. It returns a boolean value true
or false
.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn