How to Detect Keyboard Input Event in JavaScript
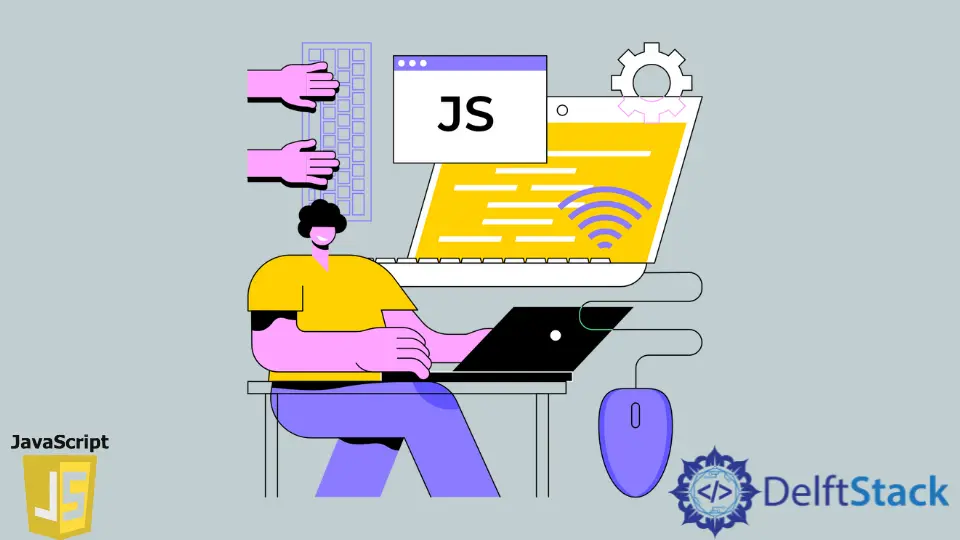
An event listener is simply a JavaScript function that’s triggered when a particular user input event happens. A simple example of such an event is a mouse click or keyboard button press. Event listener functions have to be first registered with a target. After that, whenever a particular event we’re interested in happens, our listener function is triggered. Multiple listeners can be attached to the same target that can listen to the same or different event types.
Today’s post shows you how to recognize keyboard input events in JavaScript.
Detect Keyboard Input Event Using addEventListener()
in JavaScript
This is a built-in method provided by JavaScript that registers an event listener. It is a method of the EventTarget
interface. Whenever the specified event is detected on the target, our function that is configured is called.
Syntax
target.addEventListener($type, $listener);
target.addEventListener($type, $listener, $options);
target.addEventListener($type, $listener, $useCapture);
Parameter
$type
: It is a mandatory parameter that only accepts a string that specifies the type of event to be listened to. It is case sensitive and supports various events likemouse
,keyboard
,input
,database
, etc.$listener
: It is a mandatory parameter, an object that will receive a notification when an event of the specified type occurs. This object must be implementing the EventListener interface or a JavaScript function.$options
: It is an optional parameter that specifies the characteristics of the event listener. Some of the characteristics arecapture
,once
,passive
, andsignal
.$useCapture
: It is an optional parameter that accepts Boolean values indicating whether events of this type are sent to the registered listener before being sent to an EventTarget below in the DOM tree.
There are three types of keyboard events that you can listen to keydown
, keypress
, and keyup
. The browser fires a keydown
event, when a key on the keyboard is pressed, and when it is released, a keyup
event fires. Each Keyboard event has its own keyCode
or key
. For eg, Enter button has key Enter and keyCode 13
.
Example code:
<input type="text" id="textId">
document.getElementById('textId').addEventListener('keydown', myFunction);
function myFunction() {
switch (event.key) {
case 'ArrowDown':
console.log('ArrowDown');
break;
case 'ArrowUp':
console.log('ArrowUp');
break;
case 'ArrowLeft':
console.log('ArrowLeft');
break;
case 'ArrowRight':
console.log('ArrowRight');
break;
default:
console.log(event.key, event.keyCode);
return;
}
event.preventDefault();
}
Output:
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn