Increment by 2 in for Loop in JavaScript
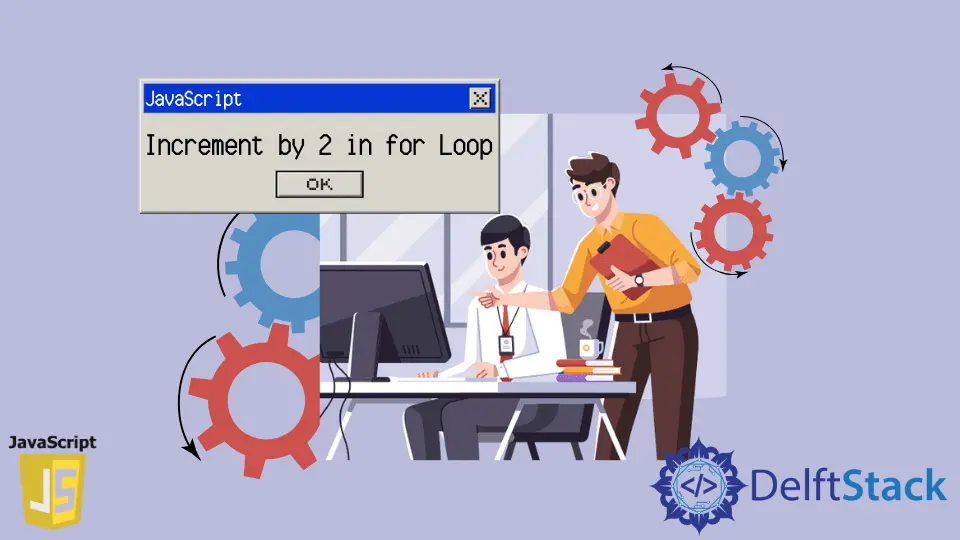
This tutorial focuses on the JavaScript for
loop and incrementing the variable by 2 in the for
loop using an example.
Loops in JavaScript
Programming languages use loops to repeat a block of code. JavaScript loops are used to iterate through the code section using for
, while
, do-while
, or for-in
loops.
It makes the code compact. It is mainly used in an array.
If you want to display a Math table, you can use a loop. It’s just a simple example; you can achieve much more with loops.
JavaScript supports four types of loops.
for
loopdo-while
loopfor-in
loopwhile
loop
Increment by 2 in a for
Loop in JavaScript
The for
statement creates a loop with three optional expressions in parentheses and is separated by semicolons. Then, a statement (typically a block
statement) runs inside the loop.
Syntax:
for ($initialization; $condition; $final - expression) {
// execute the code inside it
}
The $Initialization
is a variable declaration containing assignment expressions evaluated once before the loop begins. This variable is generally used to initialize a variable.
This expression can declare new variables with the keywords var
or let
optionally.
Variables declared with var
aren’t local to the loop; they are in the same scope as the for
loop. Variables declared with let
are local to the declaration.
Using const
instead of let
or var
will throw an error Uncaught TypeError: Assignment to constant variable
.
The $condition
is an expression that must be evaluated before each loop iteration. This is an optional expression.
By default, the condition always evaluates to true. The code/statement will only be executed if this expression evaluates true.
If the expression evaluates to false, execution skips the code/statement written inside the block.
The $final-expression
is an expression to be evaluated at the end of each loop iteration. This expression is executed before the next evaluation of the condition.
It is generally used to increment or decrement the counter variable. You can find more information about the for
loop here.
Example:
for (let i = 0; i <= 15; i += 3) {
console.log('Printing every third element', i)
}
In the above code, we are initializing variable i
with 0. Condition is that until i
is less than or equal to 15, the for
loop will continue to work and execute the code inside it.
Once the code is executed, the final expression updates the i
variable by adding 3 to the current value. You can update the variable by any number.
Once you run the above code in the browser, it will run the for
loop 6 times until i
is less than or equal to 15. The output will look something like this.
Output:
"Printing every third element", 0
"Printing every third element", 3
"Printing every third element", 6
"Printing every third element", 9
"Printing every third element", 12
"Printing every third element", 15
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn