JavaScript in_array Function
-
Use
includes()
Method to Search an Element From an Array -
Use a
for
Loop to Check an Elements Presence - Use a Function to Check an Object From an Object
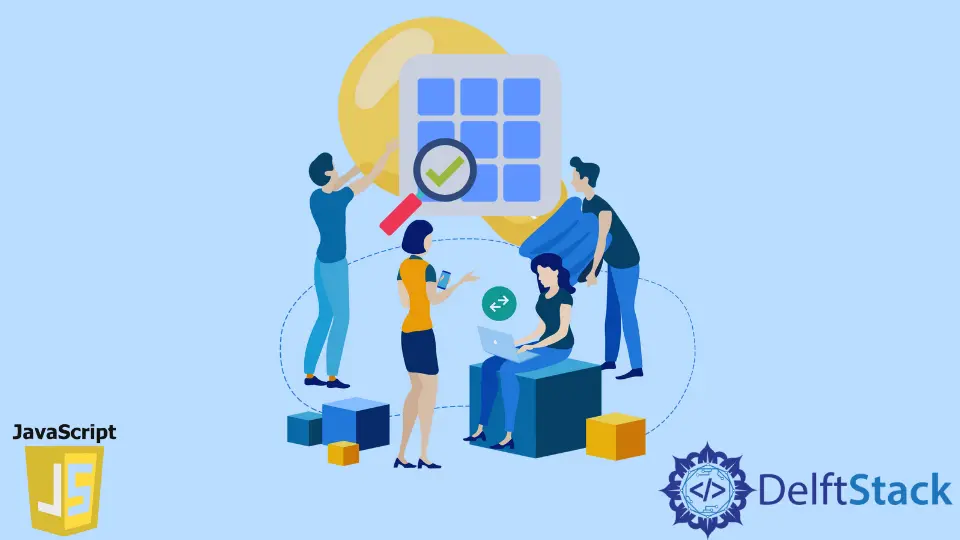
In JavaScript, if we wish to make a traverse to find a certain string or number from an array, we will have to use the includes()
method. Before introducing this convention, we usually followed the search task by initiating a for
loop over the array indexes.
Because there’s a difference between pass by value
and pass by reference
, the includes()
method doesn’t give us the right answer.
We will learn the use of the includes()
method, we will try to create the includes()
methods functionality manually, and see how to create the function in case we are making a check between objects in this article.
Use includes()
Method to Search an Element From an Array
The includes()
method generally takes the array name (where we will search for our element) at the start and then followed by a dot(.)
. In the next part, we mention our element.
Code Snippet:
var name = ['Rayan', 'Emily', 'Sarah'];
var check = 'Rayan';
console.log(name.includes(check));
Output:
This method returns results in Boolean, so our output will be consoled out as true
or false
.
Use a for
Loop to Check an Elements Presence
In this example, we will create an array and a variable that will store the element for search. We will create a simple for
loop to check our element and return the result in true
or false
.
Code Snippet:
var a = 2;
var b = [1, 2, 3];
var verdict;
for (var i = 0; i < b.length; i++) {
if (b[i] === a) {
verdict = true;
break;
} else {
verdict = false;
}
}
console.log(verdict);
Output:
Use a Function to Check an Object From an Object
JavaScript has two data types, primitive
and objects
. The primitive
data follow the pass by value
, so it was easier for us to perform the task above, but in the case of objects
, the references are passed for comparing anything.
We will not derive the perfect answer if we follow the general way of checking or the includes()
method.
Code Snippet:
function Compare(b, a) {
if (b.length != a.length) return false;
var length = a.length;
for (var i = 0; i < length; i++) {
if (b[i] !== a[i]) return false;
}
return true;
}
function inArray(a, b) {
var length = b.length;
for (var i = 0; i < length; i++) {
if (typeof b[i] == 'object') {
if (Compare(b[i], a)) return true;
} else {
if (b[i] == a) return true;
}
}
return false;
}
var a = [1, 2];
var b = [[1, 2], 3, 4];
console.log(inArray(a, b));
Output:
If you console out the specific b[i]
and a
, the values will be the same as [1,2]
. You will also find that a data type is an object
for both, but both a
and b
hold different references, and as we know, objects enable pass by reference
.
We cannot pass a reference by choice. What we did here is, in our inArray
function, we have figured out if there is any object type data within the b
object.
If the result is true, we go for the Compare
function where we have explicitly checked the b[i]
objects value and the a
objects value to get our preferable output.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript