How to Get the Class Name of an Object in JavaScript
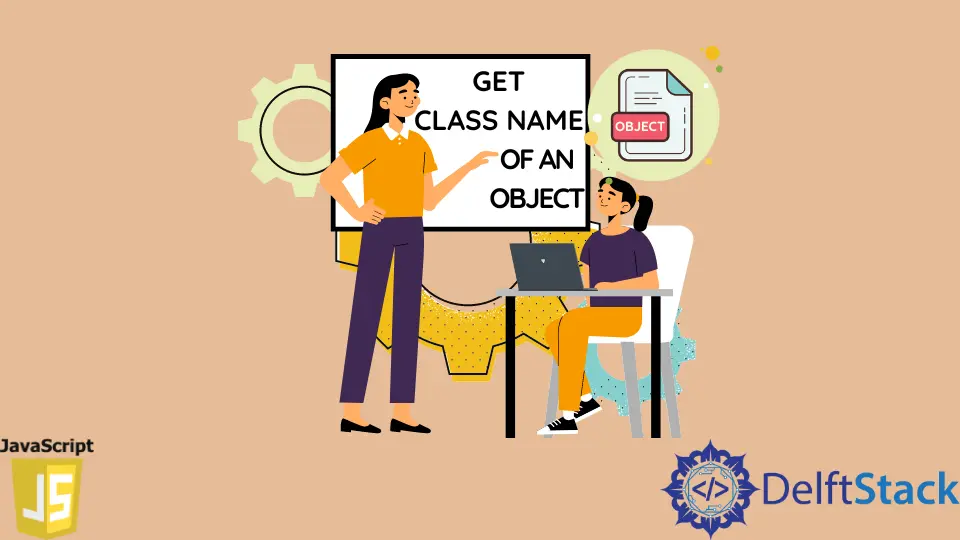
A constructor is automatically created inside the class in object-oriented programming whenever we define a class. It will be present inside the class, but it will not be visible. It only gets created when a programmer doesn’t define it explicitly. This constructor is called a default constructor.
We are interested in understanding constructors because the constructors have the same name as the class name. So, it’s easy for us to get the class name of an object in JavaScript using its constructor name.
It doesn’t matter whether the constructor is a default constructor or you create it (the programmer); the constructor’s name will always be the same as its class name.
Get the Class Name of an Object Using the constructor
Function in JavaScript
To create an object of a class, we first have to call its constructor. After calling the constructor, we store its reference inside a variable so that later we can do whatever we want with that object. Let’s understand this with an example.
Here, we have an empty class called Person
. We create an object of this class using the new
keyword and call its default constructor Person()
. Then we store it inside a variable called obj
.
Now that we have created an object of a Person
class, let’s get the object’s class name with the help of its constructor.
To get the name of a constructor, we can use a built-in function present inside JavaScript called constructor
. It will return a function. To get the specific class name, we have to use the name
property of the constructor
function.
This is shown in the below code snippet.
class Person {}
var obj = new Person();
console.log(obj.constructor.name);
Output:
Person
You can also create a function inside a class that will return the above constructor
function. And after creating an object of this class, you can call this function any number of times and get the class name of that object.
This is shown in the below code snippet.
class Person {
getClassName() {
return this.constructor.name;
}
}
Here, we have to use a this
keyword that will reference the current object on which the getClassName()
method is being called and return its class name.
The only concern with this is that the constructor’s name will change after minification if you’re minifying the JavaScript. And if you called the getClassName
function, it will return some other class name that will be present after minification and not Person
.
Also, the name
property is not supported below IE9. It is not a big concern as most users use modern browsers like Chrome and Firefox.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn