How to Get First Element of Array in JavaScript
-
Get First Array Element Using
find()
in JavaScript -
Get First Array Element Using an
array[index]
in JavaScript -
Get First Array Element Using
shift()
in JavaScript -
Get First Array Element Using
filter()
in JavaScript - Get First Array Element Using Destructuring Assignment in JavaScript
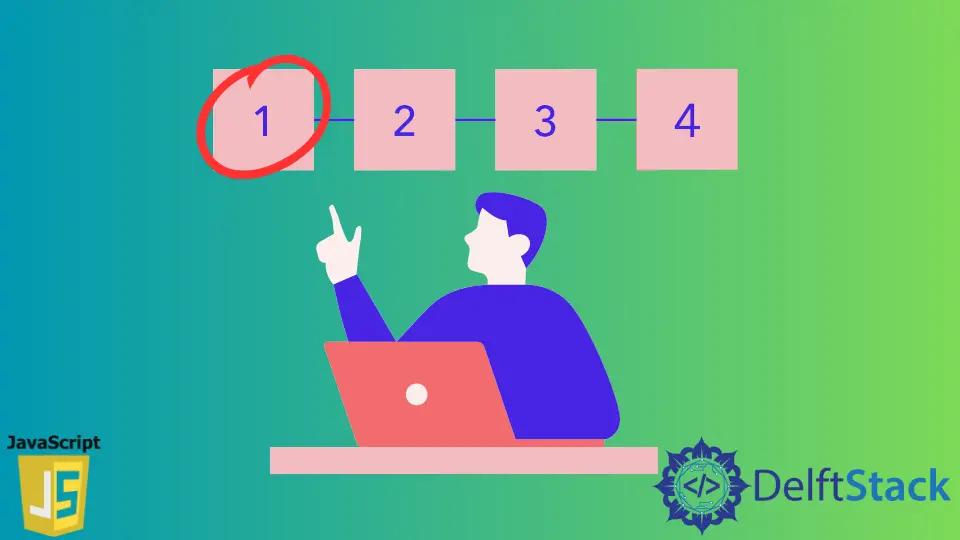
Arrays are an essential part of any programming language because it holds many elements in an ordering manner. All these elements in them are accessed via an index. In JavaScript, arrays are regular objects that hold the value on the specific key, a numeric key.
This article will introduce how to get the first element of an array in JavaScript.
JavaScript provides various methods to get the first element. Let’s learn those methods.
Get First Array Element Using find()
in JavaScript
find()
is an in-built array method provided by JavaScript, which finds the elements whose value matches the specified condition.
Syntax of find()
in JavaScript
Array.prototype.find(element => $condition)
Parameters
$condition
: It is a mandatory parameter. The user can pass any condition related to the array element, and it will try to find out the first matching element which satisfies the condition.
Return Value
Return the first value which matches with a given condition. If no condition matches, it will return undefined
.
Example code:
const arrayElements = [5, 10, 0, 20, 45];
const firstElement = arrayElements.find(element => element != undefined);
console.log(firstElement);
Output:
5
Get First Array Element Using an array[index]
in JavaScript
As discussed earlier, each array element has a unique index assigned to it. The numeric key/index
inside an array starts with 0
. Using this index, you can retrieve the first element of an array in JavaScript.
Syntax of array[index]
in JavaScript
Array.prototype[$index]
Parameters
$index
: It is a mandatory parameter that accepts only integer value that specifies the index of the element to be retrieved.
Return Value
It returns the element whose index is specified.
Example code:
const arrayElements = [5, 10, 0, 20, 45];
const firstElement = arrayElements[0];
console.log(firstElement);
Output:
5
Get First Array Element Using shift()
in JavaScript
It is an in-built array method provided by JavaScript, which removes the first element of an array and returns the removed element. The only problem with this array method is that it mutates/modifies the original array.
Syntax of shift()
in JavaScript
Array.prototype.shift()
Return Value
It returns the first element of the array, which is removed from the original array. If an array is an empty array, it will return undefined
.
Example code:
const arrayElements = [5, 10, 0, 20, 45];
const firstElement = arrayElements.shift(0);
console.log(firstElement);
console.log(arrayElements);
Output:
5
Array [10, 0, 20, 45]
Get First Array Element Using filter()
in JavaScript
It is a JavaScript in-built array method that filters out all the elements which satisfy the given condition. It will create a copy of the matching elements array. The sole difference between find()
and filter()
is that first stops the traversing as soon as the first matching element is found while later will continue till the last element of an array. It is less efficient if an array size is larger because of the traversal property.
Syntax of filter()
in JavaScript
Array.prototype.filter(element => $condition)
Parameters
$condition
: It is a mandatory parameter. The user can pass any condition related to the array element, and it will try to find out all matching element that satisfies the condition.
Return Value
Return the new array containing elements that match with a given condition.
Example code:
const arrayElements = [5, 10, 0, 20, 45];
const firstElement =
arrayElements.filter(element => element != undefined).shift();
console.log(firstElement);
console.log(arrayElements);
Output:
5
Array [5, 10, 0, 20, 45]
Get First Array Element Using Destructuring Assignment in JavaScript
Destructing assignments is a very powerful syntax available in JavaScript which allows unpacking the elements of array or properties of an object to be geted into distinct variables.
Syntax of Destructuring Assignment in JavaScript
[$variable1, $variable2, ...$restVariables] = [10, 20, 30, 40];
{$variable1} = {key: value};
Return Value
It returns the new variable through which you can access the value of an array or object.
Example code:
const arrayElements = [5, 10, 0, 20, 45];
let [firstElement] = arrayElements;
console.log(firstElement);
Output:
5
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedInRelated Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript