JavaScript Event Listener List
- Get JavaScript Event Listener List
- Use the Chrome Developer Tools Console to Get JavaScript Event Listener List
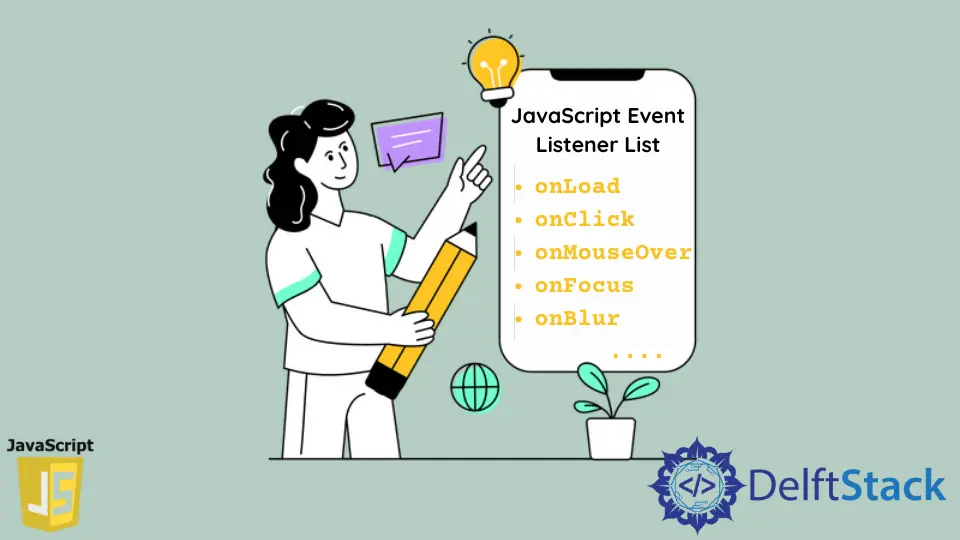
A website has many events that make the web page more interactive. For example, you click a Contact Us
button, and it quickly opens a form for you to contact because of event listeners.
This tutorial will learn how to list JavaScript Event Listeners collectively and individually. We can use different methods and techniques using Chrome Developer Tools Console, discussed with respective examples.
Get JavaScript Event Listener List
Let’s understand the basic code (HTML and JavaScript) first.
HTML Code:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Get a list of event listeners</title>
<script src="./eventList.js"></script>
</head>
<body>
<label>First name:</label>
<input type="text" id="firstname" name="first-name">
<br />
<label>Last name:</label>
<input type="text" id="lastname" name="last-name">
<br />
<button id="btn">Submit</button>
</body>
</html>
JavaScript Code:
window.onload = function() {
document.addEventListener('keypress', e => {
if (e.key === 'Enter') {
e.preventDefault();
return false;
}
});
document.getElementById('btn').addEventListener('click', function() {
console.log('You have clicked the submit button');
});
}
Output:
We have two <input>
elements of type text
and one button
on which we have attached events. There are three events, keypress
, click
, and onload
.
The keypress
event gets triggered if you press the Enter key while staying anywhere on the document
. The click
event gets fired when you click on the Submit
button.
And, when the whole page is loaded, the onload
event is fired. Now, how can we know about the event listener?
Copy the following JavaScript code to practice.
window.onload = function() {
document.addEventListener('keypress', e => {
console.log(e);
if (e.key === 'Enter') {
e.preventDefault();
return false;
}
});
document.getElementById('btn').addEventListener('click', function(event) {
console.log(event);
console.log('You have clicked the submit button');
});
}
Output:
If we press the Enter key in the first name or last name text box, it shows KeyboardEvent
with properties. And if we click on the Submit
button, the PointerEvent
listens.
In this way, we know which element has what event listener. You can use console.log(event.target);
instead of console.log(event);
in the above code if you want to know the element on which a particular event is attached.
We pressed the Enter key on both text fields and clicked on the button. Now, see the following output. The complete HTML elements are printed.
Output:
This case is useful when we know about events but need debugging.
What if we have hundreds of events? How do we know what event is for which HTML element?
Go to the page, Right-Click
on the element, and select the Inspect
option. Then, select the Event Listeners
tab and check a list of events attached to that particular HTML element.
Observe the following output.
Output:
In the output given above, you may have noted that we have three events listeners: click
, keypress
, and load
when clicking on the Submit
button, while keypress
and load
are for <input>
elements.
Use the Chrome Developer Tools Console to Get JavaScript Event Listener List
We can also use the getEventListeners(Object)
method on Chrome Developer Tools Console to get event listeners. Check the following output.
Output:
What if we want to have a complete list of events in one go? You can do this on any website to know which event is listening to what HTML element.
Type the following command on the console.
Array.from(document.querySelectorAll('*')).forEach(e => {
const ev = getEventListeners(e);
if (Object.keys(ev).length !== 0) {
console.log(e, ev)
}
});
Output:
The above output gives all event listeners their respective HTML elements side-by-side.